How to sort a list in Python?
Sorting is a common operation in programming, and Python provides straightforward and flexible tools for ordering lists. Here’s a concise explanation of how to sort lists in Python:
- Using the `sorted()` Function:
The built-in `sorted()` function returns a new list that contains all the items from the original list in ascending order. This function doesn’t modify the original list but instead provides a new sorted list. It’s versatile and can be used with any iterable, not just lists.
- List’s `sort()` Method:
Lists in Python come with a `sort()` method that modifies the list it’s called on and sorts it in place. This means the original list is changed, and no new list is created. This method is efficient when you wish to modify the original list directly and don’t need to preserve its original order.
- Reverse Sorting:
Both `sorted()` and the `sort()` method accept a `reverse` argument, which, when set to `True`, will sort the list in descending order.
- Custom Sorting Using a Key:
For more complex sorting needs, both `sorted()` and `sort()` accept a `key` argument. This argument expects a function to be passed to it, which will be used to produce a comparison key from each list element. For instance, if you had a list of strings and you wanted to sort them by their length, you could use the `len` function as the key.
- Stability in Sorting:
One notable feature of Python’s sorting tools is that they are stable. This means that when multiple records have equal keys, their original order is preserved in the sorted list. This can be particularly useful in multi-step sorting processes.
- Sorting Other Iterables:
While lists are the most commonly sorted data structure, remember that the `sorted()` function can work with any iterable, producing a list as the result. This means you can sort tuples, strings, and even dictionaries based on their keys.
Python offers versatile and user-friendly mechanisms for sorting lists and other iterables. Whether you’re performing simple ascending sorts or dealing with more complex custom sorting logic, Python’s built-in tools are both powerful and efficient, simplifying many common sorting tasks.
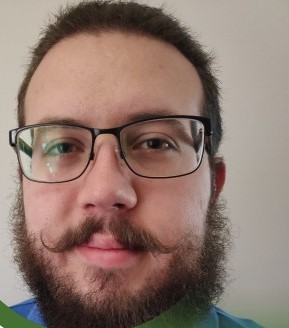
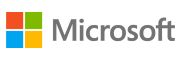