Exploring Python-SQL Integrations: A Tutorial on Database Management
Python is a versatile language with extensive library support, enabling developers to perform a variety of tasks with relative ease. It’s for this reason that many businesses choose to hire Python developers for their data management needs. In this blog post, we’ll explore the functionality of Python in the realm of databases, specifically leveraging SQL. By combining the power of Python and SQL, you can create robust systems capable of handling vast amounts of data efficiently. With the expertise of skilled Python developers, the process becomes even more streamlined and efficient, further demonstrating the value of their role in modern data operations.
Introduction to SQL
SQL, which stands for Structured Query Language, is a standard language used for managing data in relational databases. It’s used for executing queries, retrieving data, inserting new records, updating and deleting existing records, creating and modifying the structure of databases.
Introduction to SQLite
SQLite is a software library that provides a relational database management system. The ‘lite’ in SQLite means light-weight in terms of setup, database administration, and required resources. It’s integrated with Python and does not require any separate installation.
SQLite in Python
Python provides the `sqlite3` module, which allows you to work with databases using SQL in Python. The `sqlite3` module can connect to SQLite databases, execute SQL statements, and manage transactions. To use this module, you don’t need to install anything else.
Let’s get started with a simple example.
```python import sqlite3 # Connect to SQLite database in memory conn = sqlite3.connect(':memory:') ```
In the above code, we imported the sqlite3 module and established a connection with a database. The ‘:memory:’ argument creates a temporary DB in the RAM.
Creating Tables
After connecting to the database, you may want to create a table to store data. This can be achieved using the `CREATE TABLE` SQL statement.
```python c = conn.cursor() c.execute(''' CREATE TABLE employees ( id INTEGER PRIMARY KEY, name TEXT, salary REAL, department TEXT ) ''') ```
In the above code, we created a cursor object using the `cursor()` method of the connection object. We then used this cursor to execute our SQL query. We created a table named ’employees’ with columns id, name, salary, and department.
Inserting Data
To insert data into the table, we use the `INSERT INTO` SQL statement.
```python c.execute(''' INSERT INTO employees VALUES (1, 'John Doe', 80000, 'Marketing'), (2, 'Jane Doe', 90000, 'Sales'), (3, 'Mike Smith', 70000, 'HR') ''') conn.commit() ```
We’ve inserted three rows into the employees table. After executing an INSERT statement, it’s crucial to commit these changes to the database using the `commit()` method of the connection object.
Querying Data
You can use the `SELECT` SQL statement to query data.
```python c.execute('SELECT * FROM employees') rows = c.fetchall() for row in rows: print(row) ```
`fetchall()` retrieves all rows from the last executed statement. `fetchone()` retrieves the next row from the last executed statement. Here, we are fetching all rows from the employees table and printing them.
Updating Data
The `UPDATE` SQL statement allows you to update existing records.
```python c.execute('UPDATE employees SET salary = 85000 WHERE name = "John Doe"') conn.commit() ```
Here, we updated the salary of John Doe to 85000.
Deleting Data
The `DELETE` SQL statement lets you delete existing records.
```python c.execute('DELETE FROM employees WHERE id = 3') conn.commit() ```
We deleted the employee with the id 3.
Closing the Connection
After finishing your work with the database, it’s important to close the connection.
```python conn.close() ```
Working with Real-World Databases
While SQLite databases are useful for practice and light applications, you’ll likely interact with more robust databases such as MySQL or PostgreSQL in the real world. Fortunately, Python also supports these databases through libraries like `psycopg2` for PostgreSQL and `mysql-connector-python` for MySQL.
Working with these libraries involves similar processes as we’ve seen with sqlite3, but with different syntax for establishing connections and different handling of cursors and transactions.
Conclusion
Python’s capability to interact with SQL databases greatly enhances its versatility as a programming language. This functionality underscores the importance of hiring Python developers who can leverage this capability effectively. Whether they’re working with a simple SQLite database or a more complex PostgreSQL database, Python developers can make database management tasks straightforward and efficient. Be it creating, querying, updating, or deleting data, the blend of Python and SQL is a powerful tool in any developer’s toolkit.
This post just scratches the surface of what’s possible. As you become more comfortable with these concepts, you’ll find that Python provides many more tools to facilitate your work with SQL databases. This is why hiring proficient Python developers can make a big difference in your projects. From ORM libraries like SQLAlchemy that allow developers to interact with your database using Pythonic code, to data analysis libraries like Pandas that can load data directly from SQL queries into DataFrames, the possibilities are almost endless.
Table of Contents
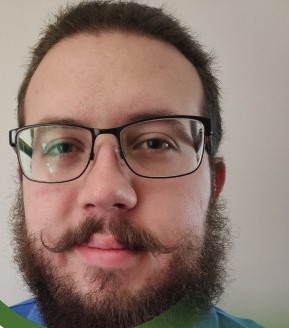
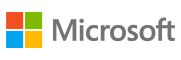