How to start a new Python project?
Starting a new Python project is an organized process that helps in building and maintaining a sustainable codebase.
- Create a New Directory: Begin by creating a dedicated directory for your project. This ensures all your project-related files and dependencies are in one place. For instance:
```bash mkdir my_python_project cd my_python_project ```
- Virtual Environment: Before installing any packages, it’s a best practice to set up a virtual environment. This isolates your project’s dependencies from system-wide Python installations. Use the `venv` module (included in the standard library since Python 3.3) to create one:
```bash python3 -m venv venv_name ```
To activate the virtual environment:
– On Windows: `venv_name\Scripts\activate`
– On macOS and Linux: `source venv_name/bin/activate`
- Initialize a Git Repository: To manage version control, initialize a git repository:
```bash git init ```
Also, create a `.gitignore` file to exclude specific files or directories (like your virtual environment) from version tracking.
- Dependencies Management: If you anticipate using third-party libraries, you’ll need to manage these dependencies. After installing any package via `pip`, always freeze the project’s dependencies into a `requirements.txt` file:
```bash pip freeze > requirements.txt ```
This makes it easier for others (or you, in the future) to install the correct versions of required libraries.
- Structuring the Project: Start with a basic structure. For instance:
– `main.py`: Entry point of your application.
– `/modules`: Directory containing various Python modules.
– `/tests`: For any test scripts or unit tests.
- Documentation: Always document your code, ideally in the form of docstrings and comments. Consider setting up tools like Sphinx for generating detailed documentation.
- ReadMe: Include a `README.md` file detailing the purpose of your project, how to set it up, and how to use it. It serves as a brief guide for anyone encountering your project.
Starting a new Python project is not just about writing code. It’s about creating a maintainable, scalable, and understandable codebase that caters to the project’s needs and future growth.
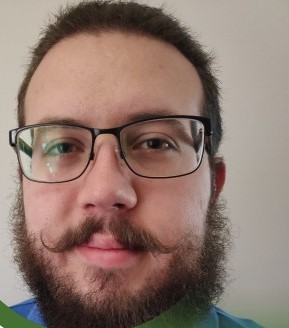
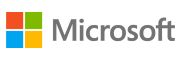