Python Tutorial: Understanding Tuple and String Data Types
Python, a versatile and widely used programming language, offers a rich variety of data types that enable developers to manipulate and manage different types of information. Two fundamental data types in Python are tuples and strings. These data types serve distinct purposes and are crucial for various programming tasks. In this tutorial, we’ll delve into the world of tuples and strings, exploring their characteristics, use cases, and how to work with them effectively.
Table of Contents
1. Introduction to Tuples and Strings
1.1. What are Tuples and Strings?
Tuples are ordered collections that can hold various data types. They are defined using parentheses and can store a combination of numbers, strings, and even other tuples. Tuples are similar to lists, but unlike lists, they are immutable, meaning their values cannot be changed after creation.
On the other hand, strings are sequences of characters enclosed in single, double, or triple quotes. They are also ordered and immutable, allowing for efficient manipulation and retrieval of individual characters.
1.2. Immutable Nature of Tuples and Strings
One of the key characteristics that tuples and strings share is their immutability. Once a tuple or string is created, its contents cannot be altered directly. Instead, any operation that appears to modify a tuple or string actually creates a new instance with the desired changes. This immutability brings benefits such as enhanced performance and reliability in various programming scenarios.
2. Exploring Tuples
2.1. Creating Tuples
Tuples can be created by enclosing comma-separated values within parentheses. For instance:
python fruits_tuple = ('apple', 'banana', 'orange')
2.2. Accessing Tuple Elements
Elements within a tuple can be accessed using indexing. Python uses a zero-based index, so the first element is at index 0, the second at index 1, and so on:
python second_fruit = fruits_tuple[1] # Retrieves 'banana'
2.3. Modifying Tuples
As mentioned earlier, tuples are immutable, so direct modification of their elements is not possible. However, you can create new tuples based on existing ones:
python modified_tuple = fruits_tuple[:1] + ('grape',) + fruits_tuple[2:]
2.4. Tuple Concatenation and Repetition
Tuples can be concatenated using the + operator, allowing you to combine multiple tuples:
python more_fruits = ('kiwi', 'pineapple') all_fruits = fruits_tuple + more_fruits
Additionally, you can repeat a tuple’s content using the * operator:
python repeated_fruits = fruits_tuple * 3
3. Understanding Strings
3.1. Creating Strings
Strings can be created using single, double, or triple quotes:
python single_quoted = 'This is a string.' double_quoted = "This is also a string." triple_quoted = '''This string can span multiple lines.'''
3.2. Accessing String Characters
Just like tuples, strings support indexing for character access:
python first_char = single_quoted[0] # Retrieves 'T'
3.3. Basic String Operations
Strings offer various built-in operations, such as calculating their length:
python length = len(single_quoted)
3.4. String Concatenation and Repetition
Strings can be concatenated using the + operator:
python greeting = "Hello, " + "world!"
Similarly, you can repeat a string using the * operator:
python repeated_greeting = greeting * 3
4. Comparing Tuples and Strings
4.1. Use Cases of Tuples and Strings
Tuples are handy for grouping related data that should remain unchanged, such as coordinates or configuration settings. Strings, on the other hand, are used to represent textual information, making them vital for tasks involving text processing, manipulation, and representation.
4.2. Differences in Manipulation
While both tuples and strings are immutable, tuples are more versatile in terms of holding heterogeneous data types. Strings, being sequences of characters, have specialized methods for text-based operations, such as searching, replacing, and formatting.
5. Advanced Tuple and String Manipulation
5.1. Tuple Packing and Unpacking
Tuples support packing multiple values into a single tuple, which can then be unpacked into separate variables:
python coordinates = (3, 7) x, y = coordinates # Unpacking
5.2. String Slicing
Strings can be sliced to extract substrings:
python substring = single_quoted[8:13] # Retrieves 'a string'
5.3. String Methods for Manipulation
Python provides a plethora of built-in string methods for various manipulations, such as upper(), lower(), replace(), and more:
python upper_case = single_quoted.upper()
Conclusion
In this tutorial, we’ve explored the key features of Python’s tuple and string data types. Tuples offer a way to store ordered collections of elements while strings enable the manipulation of textual data. Understanding the immutability and distinct characteristics of these data types is essential for writing efficient and effective Python code. By leveraging the knowledge gained here, you’ll be better equipped to handle a wide range of programming tasks, from data storage to text processing, and much more.
Table of Contents
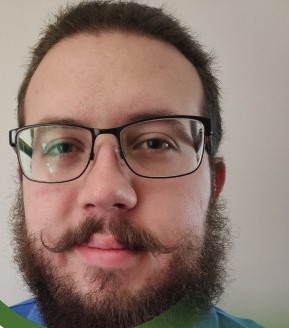
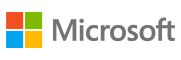