What is the difference between a tuple and a list in Python?
In Python, both tuples and lists are fundamental data structures used to store collections of items. While they have some similarities, they exhibit key differences in usage, behavior, and intent.
- Mutability:
The most pronounced difference between a tuple and a list is their mutability.
– List: Lists are mutable. This means you can modify a list after its creation by adding, removing, or changing items.
– Tuple: Tuples are immutable. Once a tuple is created, you cannot alter its content. This immutability can be useful in scenarios where you want to ensure the data remains constant throughout the program.
- Syntax:
– List: Lists are defined using square brackets, e.g., `[1, 2, 3]`.
– Tuple: Tuples are defined using parentheses, e.g., `(1, 2, 3)`. A tuple with a single element requires a trailing comma,
e.g., `(1,)`.
- Use Cases:
– List: Given their mutability, lists are typically used for collections of items that might need modification, like a list of user names, scores, or product details.
– Tuple: Tuples are often chosen for fixed collections that shouldn’t change, like coordinates (x, y, z) or RGB color values. Their immutability makes them hashable, allowing them to be used as keys in dictionaries, a role lists cannot fulfill.
- Performance:
Since tuples are immutable, they can have performance advantages in certain scenarios. For instance, iterating over a tuple can be faster than iterating over a list, especially as the size of the collection grows.
- Methods:
– List: Lists offer a range of methods for manipulation, such as `append()`, `remove()`, `insert()`, and more.
– Tuple: Being immutable, tuples come with fewer built-in methods. Commonly used ones include `count()` and `index()`.
While both tuples and lists serve as containers for storing multiple items, they cater to different needs and scenarios in programming. Lists are dynamic and versatile, perfect for collections that might change. In contrast, tuples are fixed and consistent, ideal for representing data structures that should remain constant. A seasoned Python developer will choose between them based on the specific requirements of their task.
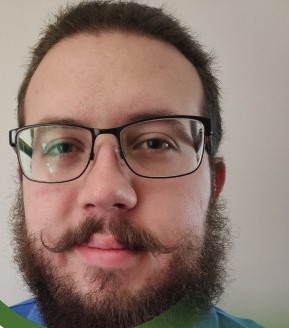
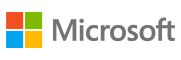