Understanding Lambda Functions in Python
Python is known for its simplicity and readability, making it a popular choice among developers. One of the features that contribute to this simplicity is Lambda functions. Lambda functions are anonymous functions, allowing you to create small, throwaway functions without formally defining them using the def keyword. They can be a powerful tool in your Python arsenal, especially when dealing with functional programming concepts or writing more concise code. In this blog post, we will dive into Lambda functions, explore their syntax and use cases, and see how they can simplify your code.
1. What are Lambda Functions?
Lambda functions, also known as anonymous functions, are short, throwaway functions without a formal name. Unlike regular functions defined using the def keyword, Lambdas are defined using the lambda keyword. These functions can take any number of arguments but can have only one expression.
2. Lambda Syntax
The basic syntax of a Lambda function is as follows:
python lambda arguments: expression
A Lambda function can take multiple arguments, but they are separated by commas and are followed by a colon :. The expression that follows the colon is the return value of the Lambda function.
2.1 Single Expression Lambdas
Let’s start with a simple example. Suppose we have two numbers and want to find their sum using a Lambda function:
python add_numbers = lambda x, y: x + y result = add_numbers(5, 10) print(result) # Output: 15
In this example, add_numbers is a Lambda function that takes two arguments x and y and returns their sum.
2.2 Multiple Expression Lambdas
Lambda functions are typically used for short, single-expression tasks. However, Python allows you to use multiple expressions by using parentheses to group them:
python multiply_and_sum = lambda x, y: (x * y, x + y) product, total = multiply_and_sum(3, 4) print(product) # Output: 12 print(total) # Output: 7
In this example, the Lambda function multiply_and_sum takes two arguments x and y and returns a tuple containing the product and the sum of the two numbers.
3. Using Lambda Functions
Lambda functions are often used in conjunction with built-in functions or higher-order functions that take other functions as arguments.
3.1 Lambda with Built-in Functions
Let’s use a Lambda function with Python’s built-in map() function to calculate the squares of a list of numbers:
python numbers = [1, 2, 3, 4, 5] squared_numbers = map(lambda x: x**2, numbers) print(list(squared_numbers)) # Output: [1, 4, 9, 16, 25]
In this example, we pass the Lambda function lambda x: x**2 as the first argument to map(), which applies the function to each element of the numbers list.
3.2 Lambda with Higher-order Functions
Lambda functions are especially useful when working with higher-order functions like filter() to selectively remove elements from a list:
python numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = filter(lambda x: x % 2 == 0, numbers) print(list(even_numbers)) # Output: [2, 4, 6, 8, 10]
In this example, we use a Lambda function to check if a number is even, and the filter() function keeps only the even numbers from the numbers list.
3.3 Sorting with Lambda
Lambda functions are frequently used as key functions when sorting lists. For instance, let’s sort a list of tuples based on the second element of each tuple:
python data = [(3, 10), (1, 5), (2, 8), (4, 2)] sorted_data = sorted(data, key=lambda x: x[1]) print(sorted_data) # Output: [(4, 2), (1, 5), (2, 8), (3, 10)]
In this example, we use a Lambda function as the key argument in the sorted() function to specify that the sorting should be based on the second element of each tuple.
4. Limitations of Lambda Functions
While Lambda functions are useful in many scenarios, they do have some limitations:
- Limited to a single expression: Lambda functions can only have one expression, which may be limiting for complex logic.
- No statements allowed: Lambda functions cannot include statements, such as print, if, for, etc. They can only contain expressions.
- Reduced readability: Overusing Lambda functions, especially for complex operations, can decrease code readability and maintainability.
5. Best Practices and Use Cases
Though Lambda functions can be a powerful tool, they should be used judiciously and in appropriate scenarios. Here are some best practices and common use cases for Lambda functions.
5.1 Use Case 1: Sorting Dictionaries
Lambda functions can be handy when sorting dictionaries based on their values. For example:
python data = {'apple': 3, 'orange': 1, 'banana': 2} sorted_data = sorted(data.items(), key=lambda x: x[1]) print(sorted_data) # Output: [('orange', 1), ('banana', 2), ('apple', 3)]
In this example, we use a Lambda function as the key argument to sort the dictionary items based on their values.
5.2 Use Case 2: Filter Lists
Lambda functions are useful when filtering lists based on specific conditions:
python numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] filtered_numbers = list(filter(lambda x: x > 5, numbers)) print(filtered_numbers) # Output: [6, 7, 8, 9, 10]
Here, we use a Lambda function with filter() to keep only the numbers greater than 5.
5.3 Use Case 3: Key Functions in Sorting
As demonstrated earlier, Lambda functions can be used as key functions for sorting complex data structures:
python students = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 21}, {'name': 'Charlie', 'age': 23}] sorted_students = sorted(students, key=lambda x: x['age']) print(sorted_students)
In this example, we use a Lambda function as the key argument to sort a list of dictionaries based on the ‘age’ key.
6. Conclusion
Lambda functions are a powerful feature in Python, allowing you to create concise and anonymous functions for specific use cases. They are particularly useful when working with built-in functions or higher-order functions that take other functions as arguments. However, it is crucial to use Lambda functions judiciously and prioritize code readability and maintainability.
By mastering Lambda functions, you can enhance your Python programming skills and write more concise and expressive code. So, go ahead and experiment with Lambda functions in your projects to take full advantage of this feature and simplify your code. Happy coding!
Table of Contents
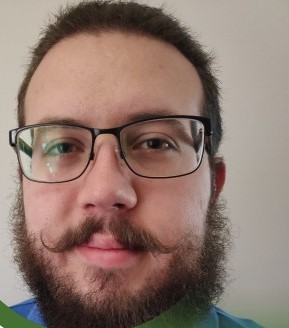
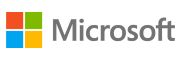