Understanding Recursion and its Applications in Python
Recursion is a powerful programming concept that enables a function to call itself during its execution. It might sound mind-boggling at first, but once you grasp its essence, recursion becomes an essential tool in your programming arsenal. Recursion can lead to elegant and concise solutions to complex problems that might otherwise be challenging to implement iteratively.
In this blog, we’ll take a deep dive into recursion, understand how it works, explore its applications, and provide practical code samples in Python to solidify your understanding. By the end of this article, you’ll be equipped with the knowledge and confidence to wield recursion effectively in your own projects.
1. Understanding Recursion:
What is Recursion?
Recursion is a programming technique where a function calls itself to solve a problem. Instead of solving the entire problem at once, the function divides it into smaller subproblems. These subproblems are solved using the same function, and the results are combined to solve the original problem.
Base Case and Recursive Case:
Every recursive function has two essential components: the base case and the recursive case. The base case acts as the stopping condition, preventing the function from calling itself indefinitely. The recursive case defines the rules and steps to break down the problem into smaller subproblems.
For instance, consider calculating the factorial of a number using recursion. The base case would be when the number is 1, as the factorial of 1 is always 1. The recursive case would involve multiplying the number with the factorial of (n-1).
Code Sample – Factorial using Recursion:
python def factorial(n): if n == 1: return 1 else: return n * factorial(n - 1)
The Call Stack:
Recursion relies on a data structure called the “call stack” to keep track of the function calls. Every time a function is called, its local variables and context are pushed onto the call stack. When a function returns, it is popped from the call stack, and the program resumes execution from the calling function.
It’s crucial to design recursive functions with a proper base case and ensure the problem size decreases with each recursive call. Otherwise, the function will lead to infinite recursion, causing a stack overflow error.
2. Anatomy of Recursive Functions:
Recursive Function Structure:
A recursive function typically follows this structure:
Define the base case(s).
Implement the recursive case(s) that call the function itself with modified arguments.
Code Sample – Simple Recursive Function:
Infinite Recursion and How to Avoid It:
Infinite recursion occurs when a base case is not met or is improperly defined. This leads to the function calling itself indefinitely, causing a stack overflow error. To avoid infinite recursion, always ensure the base case is correctly implemented and that the recursive calls converge towards the base case.
Code Sample – Incorrect Infinite Recursion:
python def incorrect_countdown(n): print(n) incorrect_countdown(n + 1) # Incorrect recursive call
3. The Beauty of Recursion:
Visualizing Recursive Algorithms:
Recursion can sometimes be challenging to grasp conceptually. Visualizing the flow of recursive algorithms can aid in understanding how they work. Tools like “Python Tutor” can visually demonstrate the execution of recursive functions, allowing you to follow the call stack and variable values step-by-step.
Recursive vs. Iterative Approaches:
While recursion can lead to elegant and concise code, it’s essential to recognize that not all problems are best suited for recursion. In some cases, an iterative approach might be more efficient and practical. Striking a balance between recursion and iteration is crucial to becoming a proficient programmer.
4. Recursive Applications in Python:
Factorials using Recursion:
Factorials are a classic example of a problem that can be elegantly solved using recursion. The factorial of a non-negative integer ‘n’ is the product of all positive integers less than or equal to ‘n’. We have already seen a sample implementation of the factorial function above.
Fibonacci Sequence using Recursion:
The Fibonacci sequence is another classic example where recursion shines. The sequence starts with 0 and 1, and each subsequent number is the sum of the two preceding ones. The nth Fibonacci number can be calculated recursively by adding the (n-1)th and (n-2)th Fibonacci numbers.
Code Sample – Fibonacci Sequence using Recursion:
python def fibonacci(n): if n <= 0: return 0 elif n == 1: return 1 else: return fibonacci(n - 1) + fibonacci(n - 2)
Directory Tree Traversal:
Recursion is invaluable when traversing directory trees and nested data structures. The structure of directories can be visualized as a tree, with subdirectories and files as nodes. Recursive directory tree traversal helps in listing all files and directories within a given directory and its subdirectories.
Code Sample – Directory Tree Traversal using Recursion:
python import os def list_files(directory): for item in os.listdir(directory): item_path = os.path.join(directory, item) if os.path.isdir(item_path): list_files(item_path) else: print(item_path)
5. Tail Recursion Optimization:
Tail Recursion and Its Limitations:
Tail recursion occurs when a recursive function makes its last operation a recursive call. Tail recursion can be optimized to avoid unnecessary stack space consumption, making it as efficient as an iterative solution. However, Python does not support tail call optimization natively.
Tail Recursion Optimization in Python:
Although Python lacks built-in tail call optimization, you can simulate it using various techniques. One common approach is to use a wrapper function to handle the recursion while maintaining the benefits of tail recursion.
Code Sample – Tail Recursion Optimization using a Wrapper Function:
python def factorial(n): return factorial_helper(n, 1) def factorial_helper(n, acc): if n == 0: return acc else: return factorial_helper(n - 1, acc * n)
6. Handling Complex Problems with Recursion:
Divide and Conquer Strategy:
Divide and Conquer is a powerful algorithmic technique that involves breaking down a complex problem into smaller, more manageable subproblems. Recursion can be instrumental in implementing the divide and conquer strategy, as each subproblem can be independently solved using the same function.
Backtracking Algorithms:
Backtracking is an algorithmic paradigm for solving problems by trying all possible options and then choosing the best result. Recursive backtracking is often used in puzzles, games, and constraint satisfaction problems.
Conclusion:
Recursion is a fascinating and versatile programming concept that can open up new avenues of problem-solving. It enables elegant, concise, and often efficient solutions to complex problems. By mastering recursion in Python, you gain a valuable tool in your programming arsenal. So, embrace recursion, practice its applications, and unlock your potential as a proficient Python programmer. Happy coding!
Table of Contents
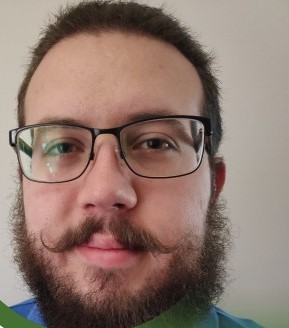
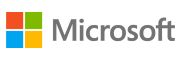