How to use lambda functions in Python?
Lambda functions, sometimes referred to as “anonymous functions” or “lambda expressions”, are a unique and powerful feature in Python, allowing for the creation of small, inline functions without the need for a formal function declaration.
- Basic Structure:
A lambda function’s syntax is succinct:
```python lambda arguments: expression ```
This structure allows you to define a function with specified `arguments` and return the value of the `expression` when the lambda is invoked.
- Characteristics:
– Simplicity: Lambda functions are concise, designed for simple operations that can be expressed in a single expression. They don’t support multiple expressions or statements.
– Anonymity: Unlike regular functions defined using the `def` keyword, lambda functions don’t have a name (hence “anonymous”). However, they can be assigned to variables, effectively giving them a name if needed.
– Flexibility: They can be used wherever function objects are required, making them suitable for places where short, throwaway functions are advantageous.
- Common Use Cases:
– Sorting & Filtering: Lambda functions shine when used with functions like `sorted()`, `filter()`, and `map()`. For example, if you want to sort a list of dictionaries based on a particular key, a lambda can be used to specify the sorting key.
– Functional Constructs: They’re frequently employed in functional programming constructs or when a short-lived function is passed as an argument to higher-order functions.
– Event-Driven Programming: Lambdas are also useful in scenarios like GUI programming, where you might want to define a quick action for a button click without formally defining a full function.
- Points of Caution:
– Readability: Due to their concise nature, overusing lambda functions or employing them for complex operations can reduce code readability. If the logic becomes non-trivial, it’s usually a good idea to use a named, regular function instead.
– Limitations: Lambda functions inherently have limitations, like being restricted to a single expression. They don’t support statements, assignments, or multiple expressions.
Lambda functions in Python provide developers with a tool for writing quick, throwaway functions without a full declaration. When used judiciously, they can make code concise and expressive. However, it’s essential to balance brevity with clarity, ensuring your code remains understandable to others (and your future self!).
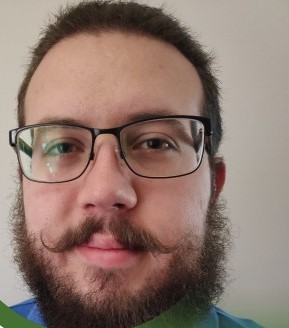
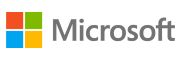