How to use regular expressions in Python?
Regular expressions (often abbreviated as regex or regexp) are sequences of characters that define a search pattern. In Python, the `re` module in the standard library provides functions and classes for working with regular expressions. Here’s a concise guide on using regex in Python:
- The `re` Module:
Before using regex functions, you need to `import re`. This module provides all the necessary tools for creating and manipulating regular expressions in Python.
- Common Functions:
– `re.match()`: Determines if the regex matches at the beginning of the string.
– `re.search()`: Searches the string for a match and returns a match object if found. Unlike `match()`, it looks for patterns throughout the string.
– `re.findall()`: Returns all non-overlapping matches as a list of strings.
– `re.finditer()`: Similar to `findall()`, but returns an iterator yielding match objects.
– `re.sub()`: Replaces occurrences of the regex pattern with a specified string.
- Compiling Regular Expressions:
For efficiency, especially when a particular regex pattern will be used multiple times, you can compile it into a regex object using `re.compile()`. This object provides methods like `match()`, `search()`, and others, which operate identically to the module-level functions but use the compiled pattern.
- Match Objects:
When a search or match is successful, a match object is returned. This object provides methods to get information about the search and the result:
– `.group()`: Returns one or more matching groups.
– `.start()` and `.end()`: Return the starting and ending positions of the match.
– `.span()`: Returns a tuple containing the (start, end) positions of the match.
- Pattern Modifiers:
There are several modifiers that can be used to change the behavior of the regex:
– `re.I` or `re.IGNORECASE`: Makes the match case-insensitive.
– `re.M` or `re.MULTILINE`: Makes `^` and `$` match the start and end of each line, respectively.
– `re.S` or `re.DOTALL`: Makes the `.` character match any character, including newline (`\n`).
The `re` module in Python provides a comprehensive toolkit for working with regular expressions, allowing for sophisticated string searching, matching, and manipulation. As with any powerful tool, regular expressions require practice to use effectively. Understanding the core functions and features of the `re` module is essential for harnessing the full power of regex in Python.
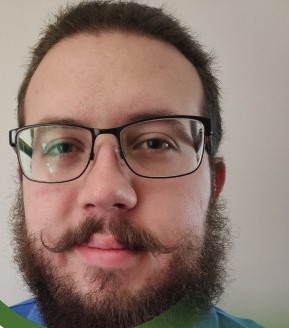
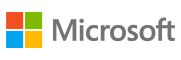