Understanding the Basics of Variables and Data Types in Python
Python is an incredibly versatile, powerful programming language widely adopted across various industries. Its simplicity and readability have made it a favorite among beginners and experienced developers alike. This tutorial will demystify the basics of Python, focusing on variables and data types.
Introduction to Python Variables
A variable is a named space in memory where a programmer can store data and manipulate it later. Variables are at the heart of any programming language, and Python is no exception.
Creating a variable in Python is as simple as assigning a value to a name using the `=` operator. For instance:
x = 10 name = "Alice"
In the example above, `x` is a variable holding the integer `10`, and `name` is another variable holding the string `”Alice“`.
Data Types in Python
Different types of data that can be stored and manipulated in Python are classified into various data types. The primary ones are:
1. Numbers: Python supports three numeric data types: integer (int), floating point number (float), and complex numbers (complex).
x = 5 # An integer assignment y = 0.5 # A floating point z = 1+2j # A complex number; note 'j' to specify the imaginary part
2. String (str): Strings are sequences of character data enclosed in quotes. Python supports single, double, and triple quotes to denote a string.
s1 = 'Hello' # A string with single quotes s2 = "Hello World" # A string with double quotes s3 = '''Hello, World!''' # A multiline string with triple quotes
3. List: A list in Python is an ordered collection of items that can be of any type. It is mutable, meaning the values in a list can be changed.
fruits = ['apple', 'banana', 'cherry'] # A list of strings numbers = [1, 2, 3, 4, 5] # A list of integers
4. Tuple: A tuple is similar to a list, but it is immutable. This means that once a tuple is created, it cannot be modified.
t = ('apple', 'banana', 'cherry') # A tuple of strings
5. Dictionary (dict): A dictionary is an unordered collection of key-value pairs. It’s a mutable data type, and the values can be of any type.
person = { 'name': 'Alice', 'age': 25, 'country': 'USA' } # A dictionary with key-value pairs
6. Boolean (bool): A Boolean data type can hold one of two values: `True` or `False`. Booleans are often used in conditional expressions.
is_raining = False # A boolean value
Type Function
The `type()` function is a built-in Python function that helps determine the data type of a variable. For example:
x = 10 print(type(x)) # Outputs: <class 'int'>
In this case, the `type()` function confirms that `x` is an integer.
Dynamic Typing
Python is dynamically typed, meaning the type of variable can change throughout the program. Here’s an example:
x = 10 print(type(x)) # Outputs: <class 'int'> x = "Hello" print(type(x)) # Outputs: <class 'str'>
Initially, `x` was an integer, but later it was assigned a string. Python easily handled this switch.
Type Conversion
Python provides in-built functions to convert one data type to another, like `int()`, `float()`, `str()`, etc.
num_str = "123" num_int = int(num_str) # Converts string to integer print(type(num_int)) # Outputs: <class 'int'>
In this case, the string `“123”` was converted to an integer using the `int()` function.
Conclusion
Understanding variables and data types is fundamental to programming in Python. From storing simple numbers to complex data structures, Python’s flexibility and dynamic typing system make it an excellent choice for a wide range of programming tasks.
Remember, practice is the key to mastering any programming language. Spend some time creating and manipulating variables with different data types to consolidate your learning. As you grow more comfortable, you’ll be ready to tackle more complex Python challenges.
Table of Contents
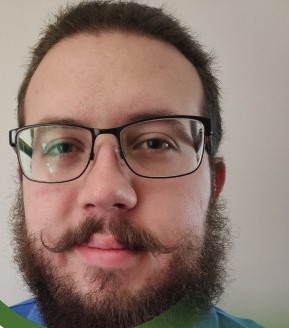
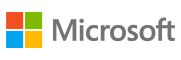