How to Use Python Functions for Web Automation
In today’s digital age, automation has become an essential aspect of various industries and disciplines. From business processes to personal productivity, the ability to automate tasks can significantly enhance efficiency and reduce human error. Web automation, in particular, has gained tremendous popularity due to its potential to handle repetitive tasks on websites, such as data scraping, form filling, and interaction with web services. Python, with its rich ecosystem of libraries and packages, provides a powerful platform for creating automation scripts. In this blog post, we’ll explore how to harness the capabilities of Python functions for web automation, enabling you to streamline your web-related tasks effectively.
Table of Contents
1. Introduction to Web Automation with Python
Web automation involves writing scripts or programs that perform various tasks on websites automatically. These tasks could include submitting forms, extracting data, clicking buttons, and navigating through web pages. Python, a versatile and user-friendly programming language, is an excellent choice for web automation due to its simplicity and a wide range of libraries designed for this purpose.
2. Benefits of Using Functions for Web Automation
Utilizing functions in your Python scripts can significantly enhance the clarity, reusability, and maintainability of your code. Functions allow you to break down complex tasks into smaller, manageable chunks, making it easier to debug and modify your automation scripts. By structuring your automation code using functions, you create a more organized and efficient workflow, ensuring that your web automation tasks are executed smoothly.
3. Getting Started: Setting Up Your Environment
Before diving into web automation, you need to set up your Python environment. Make sure you have Python installed on your system. You can download it from the official Python website. Additionally, you might need to install external libraries like requests and BeautifulSoup for web interaction and web scraping, respectively. You can install these libraries using the following commands:
python pip install requests pip install beautifulsoup4
4. Building a Foundation: Understanding Python Functions
In Python, a function is a block of organized, reusable code designed to perform a specific task. Functions take input, process it, and return output. Defining functions in Python involves using the def keyword, followed by the function name, parentheses containing any required parameters, and a colon. The function body is indented and contains the code that carries out the desired actions.
python def greet(name): return f"Hello, {name}!"
5. Using Python Functions for Web Automation
5.1. Automating Web Interaction with Requests
The requests library in Python allows you to send HTTP requests and interact with web pages programmatically. You can use the get() method to retrieve a web page’s content and the post() method to submit data to a web server. Here’s an example of how you can use the requests library to automate web interactions:
python import requests def get_web_page(url): response = requests.get(url) if response.status_code == 200: return response.text else: return None
5.2. Web Scraping with BeautifulSoup
Web scraping involves extracting information from web pages. The BeautifulSoup library simplifies this process by providing a convenient way to parse HTML and XML documents. You can use it to locate specific elements on a web page and extract their contents. Here’s a simple example:
python from bs4 import BeautifulSoup html_content = "<html><body><p>Hello, Web Scraping!</p></body></html>" soup = BeautifulSoup(html_content, 'html.parser') text = soup.p.get_text() print(text) # Output: Hello, Web Scraping!
5.3. Filling Forms Automatically
Filling out online forms manually can be time-consuming. Python’s automation capabilities can save you valuable time by automatically populating form fields and submitting forms. This is particularly useful for tasks like logging into websites or submitting data. Here’s a basic example using the requests library to log into a website:
python import requests def login(username, password): login_url = "https://example.com/login" data = { 'username': username, 'password': password } response = requests.post(login_url, data=data) if response.status_code == 200: return "Login successful" else: return "Login failed"
6. Organizing Your Code: Best Practices for Function-based Automation
As your automation scripts grow, maintaining clean and organized code becomes crucial. Follow these best practices:
- Break down complex tasks into functions for modularity.
- Use meaningful function names and comments to enhance code readability.
- Group related functions into separate modules for better organization.
- Implement error handling to gracefully handle unexpected scenarios.
7. Error Handling and Exception Management
Web automation scripts may encounter errors due to various reasons, such as network issues, website changes, or user input errors. Incorporating proper error handling and exception management is essential to ensure that your scripts continue running smoothly. Use try-except blocks to catch and handle exceptions gracefully:
python try: response = requests.get(url) response.raise_for_status() except requests.exceptions.RequestException as e: print(f"An error occurred: {e}")
8. Case Study: Automating Data Extraction and Report Generation
Let’s consider a real-world example: automating the process of extracting stock prices from a financial website and generating a daily report. By combining web scraping and data manipulation, you can create a script that fetches the latest stock prices, calculates price changes, and generates a report:
python import requests from bs4 import BeautifulSoup def get_stock_prices(): url = "https://example.com/stocks" response = requests.get(url) soup = BeautifulSoup(response.content, 'html.parser') stock_elements = soup.find_all('div', class_='stock') stock_prices = {} for stock_element in stock_elements: symbol = stock_element.find('span', class_='symbol').text price = float(stock_element.find('span', class_='price').text) stock_prices[symbol] = price return stock_prices def calculate_price_changes(old_prices, new_prices): price_changes = {} for symbol, new_price in new_prices.items(): if symbol in old_prices: old_price = old_prices[symbol] change = new_price - old_price price_changes[symbol] = change return price_changes def generate_report(price_changes): report = "Stock Price Changes:\n" for symbol, change in price_changes.items(): report += f"{symbol}: {change}\n" return report # Main script old_prices = get_stock_prices() new_prices = get_stock_prices() price_changes = calculate_price_changes(old_prices, new_prices) report = generate_report(price_changes) print(report)
9. Future Prospects and Advanced Techniques
While this blog post provides a solid foundation for using Python functions in web automation, the world of web automation is vast and constantly evolving. Consider exploring more advanced techniques, such as headless browsers (Selenium), handling dynamic web pages, and integrating APIs for more sophisticated automation tasks.
Conclusion
Python’s functions offer a powerful and organized approach to web automation. By breaking down complex tasks into modular functions, you can create efficient and maintainable scripts that handle a wide range of web-related tasks. Whether you’re automating data extraction, form filling, or report generation, Python’s capabilities empower you to optimize your workflow and save valuable time in the world of web automation. So, dive into the world of Python web automation and let your imagination be your guide in streamlining your web tasks.
Table of Contents
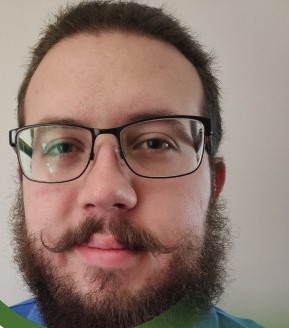
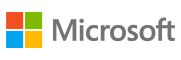