What are Python generators?
Python Generators are a special type of iterator, offering a more concise way to create iterable objects without having to define complex classes with methods like `__iter__()` and `__next__()`. They allow for lazy evaluation, generating values on the fly and yielding them one by one, which can be particularly efficient for large data sets or streams.
Key Points about Generators:
- Creation using Functions:
Unlike regular functions that return a single value using the `return` statement, generators use the `yield` keyword to return a sequence of values. When a generator function is called, it doesn’t execute the function body immediately but returns a generator object.
```python def simple_generator(): yield 1 yield 2 yield 3 ```
- Lazy Evaluation:
Generators produce values on-the-go and don’t store them in memory. This means they can represent infinite sequences and are memory-efficient, making them useful for large data streams.
- Usage:
You can use generators in a manner similar to other iterables, like lists. The most common way is to loop through the yielded values using a `for` loop.
```python for value in simple_generator(): print(value) ```
- Generator Expressions:
Similar to list comprehensions, Python supports generator expressions that allow for a more concise creation of generators.
```python squared = (x*x for x in range(10)) ```
This creates a generator that yields squared numbers from 0 to 81.
- Stateful Nature:
Generators maintain their state between successive calls. After yielding a value, execution is paused, and it resumes just after the last `yield` upon the next iteration. Once exhausted, a generator can’t be restarted or reused.
- Use Cases:
Generators are especially valuable when dealing with:
– Large data sets where loading everything into memory isn’t feasible.
– Infinite streams of data, like reading real-time sensor data.
– Situations where computations on subsequent data depend on previous values.
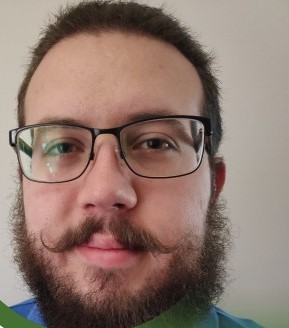
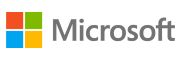