What are modules and how to use them in Python?
In Python, a module is a file containing Python definitions, functions, and statements. The purpose of modules is to organize code in a logical and coherent manner, promoting modularity, code reuse, and a clean namespace. By using modules, developers can avoid function and variable name conflicts, and structure larger applications into manageable pieces.
- Using Modules:
To access the functions, classes, and variables defined in a module, you’ll first need to import it. Python provides various ways to import modules:
– Basic Import: Use the `import` statement followed by the module name.
```python import math print(math.sqrt(25)) ```
– Alias Import: If a module name is long or conflicts with another name in your code, you can provide an alias for it.
```python import datetime as dt ```
– Selective Import: If you only need specific functions or classes from a module, you can import just those.
```python from math import sqrt, pi ```
– Wildcard Import: You can import everything from a module using a wildcard (`*`). However, this is generally discouraged as it can clutter the namespace.
```python from math import * ```
- Creating Your Own Modules:
Any Python file can act as a module. For example, if you have a file `utilities.py` with several functions, you can import it in another Python script using `import utilities`.
- Python Package:
When your application grows, you might want to organize multiple modules into packages. A package is simply a way of collecting related modules together within a single directory. To make Python treat a directory as a package, it must contain a file named `__init__.py` (which can be empty).
- The Standard Library:
Python comes with a rich set of modules known as the Standard Library. These modules offer functionalities for a wide range of tasks, from file I/O, regular expressions, and web services, to data compression, testing, and more.
Modules in Python provide a structured way to organize and reuse code. By understanding how to create, use, and import modules, developers can write organized, modular, and efficient code, leveraging both the vast Python Standard Library and their own custom modules.
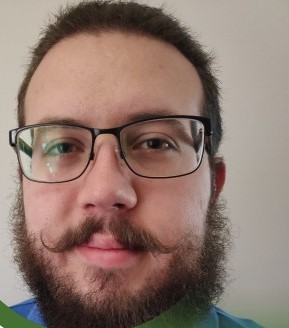
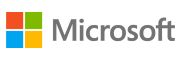