What is inheritance in Python?
Inheritance is one of the fundamental pillars of object-oriented programming. It allows for the creation of a new class that is based on an existing class. The new class inherits attributes and behaviors (methods) from the existing class, facilitating code reuse, logical organization, and the establishment of relationships between classes.
Understanding Inheritance in Python:
- Base and Derived Classes:
In the context of inheritance, the existing class from which another class inherits is often referred to as the base or parent class. The new class that inherits from the base class is termed the derived or child class.
- Basic Inheritance:
To create a derived class, you specify the base class in parentheses after the derived class’s name.
```python class BaseClass: pass class DerivedClass(BaseClass): pass ```
Here, `DerivedClass` inherits from `BaseClass`.
- Extending and Overriding:
– Extending: The derived class can introduce new attributes or methods that weren’t present in the base class.
– Overriding: If the derived class has a method with the same name as a method in the base class, the derived class’s version will override the base class’s version. This is useful when the behavior in the derived class needs to differ from the base class.
- The `super()` Function:
This is used to call methods from the base class in the derived class. For instance, it’s commonly used in the `__init__` method to ensure that the initialization code of the base class is executed when an object of the derived class is created.
- Multiple Inheritance:
Python supports multiple inheritance, where a derived class can inherit from more than one base class.
```python class Base1: pass class Base2: pass class Derived(Base1, Base2): pass ```
- Benefits:
– Code Reuse: Avoid rewriting the same code; inherit functionalities from base classes.
– Logical Structure: Establish clear hierarchies and relationships between classes.
– Extensibility: Easily extend functionalities by adding to or modifying derived classes.
Inheritance in Python allows developers to establish relationships between classes, fostering a logical organization of code. It promotes code reuse, reduces redundancy, and paves the way for building complex systems in a modular fashion. Understanding inheritance is essential for any Python developer looking to harness the power of object-oriented programming fully.
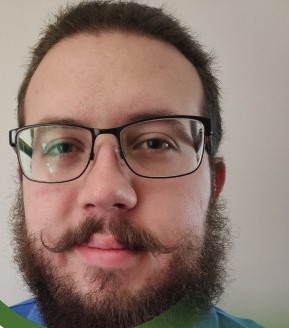
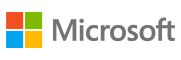