How to work with CSV files in Python?
Working with CSV files in Python** is facilitated primarily by the built-in `csv` module, which provides functionality to read from and write to CSV files effortlessly.
- Reading CSV Files:
To read a CSV file, you can use the `csv.reader()` function. This function returns an iterator which produces rows in the CSV file as lists of strings. Here’s a simple example:
```python import csv with open('data.csv', 'r') as file: reader = csv.reader(file) for row in reader: print(row) ```
- Writing CSV Files:
For writing to a CSV file, the `csv.writer()` function is used. You can then use the `writerow()` method of this writer object to write individual rows.
```python import csv data = [["Name", "Age", "Occupation"], ["Alice", 29, "Engineer"], ["Bob", 35, "Manager"]] with open('data.csv', 'w', newline='') as file: writer = csv.writer(file) writer.writerows(data) ```
- DictReader and DictWriter:
For a more structured approach, `csv` offers `DictReader` and `DictWriter` classes, which allow you to work with CSV files as dictionaries. This can be especially handy when dealing with files with header rows.
```python # Reading using DictReader with open('data.csv', 'r') as file: reader = csv.DictReader(file) for row in reader: print(row['Name'], row['Age']) # Writing using DictWriter headers = ["Name", "Age", "Occupation"] data = [{"Name": "Alice", "Age": 29, "Occupation": "Engineer"}, {"Name": "Bob", "Age": 35, "Occupation": "Manager"}] with open('data.csv', 'w', newline='') as file: writer = csv.DictWriter(file, fieldnames=headers) writer.writeheader() writer.writerows(data) ```
The `csv` module in Python makes it incredibly straightforward to work with CSV files. Whether dealing with simple lists or structured dictionaries, this module has tools to help you efficiently process CSV data. Always remember to handle exceptions and edge cases, especially when working with diverse and large datasets.
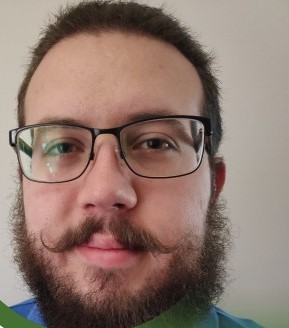
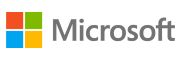