How to work with databases using Python ORM tools?
Working with databases in Python often involves the use of Object-Relational Mapping (ORM) tools. These tools allow developers to interact with databases using Python classes, making database operations more intuitive and abstracting away much of the SQL intricacies.
- Introduction to ORM:
ORM tools bridge the gap between Python’s object-oriented nature and the relational nature of databases. Instead of writing raw SQL queries, you define classes in Python, and these classes correspond to tables in the database. The attributes of these classes correspond to the columns of the tables.
- SQLAlchemy:
One of the most popular ORM tools in Python is `SQLAlchemy`. It provides both a high-level ORM and a low-level SQL expression language. To get started with SQLAlchemy:
– Define your classes and map them to the database tables.
– Create a session to interact with the database.
```python from sqlalchemy import create_engine, Column, Integer, String, Sequence from sqlalchemy.ext.declarative import declarative_base from sqlalchemy.orm import sessionmaker Base = declarative_base() class User(Base): __tablename__ = 'users' id = Column(Integer, Sequence('user_id_seq'), primary_key=True) name = Column(String(50)) engine = create_engine('sqlite:///:memory:') Base.metadata.create_all(engine) Session = sessionmaker(bind=engine) session = Session() new_user = User(name="Alice") session.add(new_user) session.commit() ```
- Django ORM
If you’re developing a web application using the Django framework, you’ll use its built-in ORM. Django’s ORM is very high-level, and it integrates seamlessly with the framework’s other features.
– In Django, each model class corresponds to a table in the database.
– Querying the database is done using Python code, abstracting away the SQL.
```python from django.db import models class Blog(models.Model): name = models.CharField(max_length=100) tagline = models.TextField() # Once defined, run 'python manage.py makemigrations' and 'python manage.py migrate' to update the database. # To create and save an instance: blog = Blog(name="My Blog", tagline="A simple blog.") blog.save() ```
ORM tools in Python, such as SQLAlchemy and Django ORM, provide an elegant way to interact with databases using Pythonic constructs. They abstract away the complexities of raw SQL, allowing developers to focus on application logic while maintaining efficient database operations.
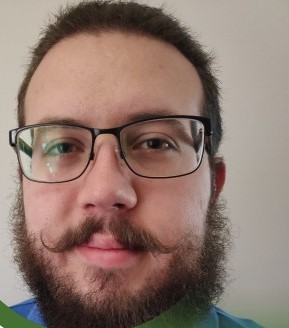
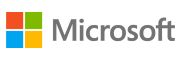