How to work with Excel files in Python?
Working with Excel files in Python has been made remarkably straightforward thanks to a few specialized libraries. The most notable one is `openpyxl` for `.xlsx` files, while `xlrd` and `xlwt` can be employed for the older `.xls` format.
- Reading Excel files:
To read Excel files, `openpyxl` provides a simple interface. First, you’ll want to load your workbook, and then select the desired sheet. From there, cells can be accessed by row and column indices.
```python from openpyxl import load_workbook wb = load_workbook('example.xlsx') sheet = wb.active cell_value = sheet.cell(row=1, column=1).value ```
- Writing to Excel files:
Writing or modifying Excel files is similarly intuitive. After making changes, simply save the workbook.
```python from openpyxl import Workbook wb = Workbook() sheet = wb.active sheet.cell(row=1, column=1, value="Hello, Excel!") wb.save('output.xlsx') ```
- Handling Complex Features:
`openpyxl` isn’t limited to basic reading and writing. It also supports creating charts, adding images, conditional formatting, and even managing complex styling, making it a comprehensive solution for Excel operations in Python.
- Alternatives:
For users seeking a more data analysis-oriented approach, the `pandas` library provides functions like `read_excel()` and `to_excel()`, streamlining the process of reading and writing Excel files into its DataFrame structure.
Recommendation:
For most tasks, `openpyxl` is robust and comprehensive. However, if you’re already using `pandas` for data analysis, leveraging its built-in Excel functions can be efficient. Always remember to install the required libraries using `pip`, e.g., `pip install openpyxl pandas`.
Python offers powerful tools for interacting with Excel files, whether for data extraction, automation, or analysis, making it an excellent choice for Excel-related tasks.
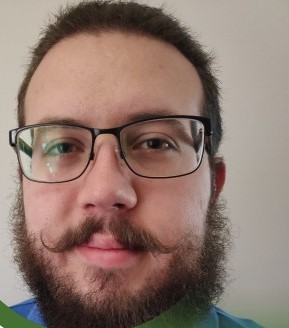
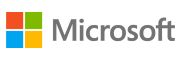