How to work with time zones in Python?
Handling time zones correctly is pivotal in applications where time-sensitive operations or user interactions occur across different regions. Python, through its standard library and third-party packages, offers tools to manage time zones effectively.
- The `datetime` module: Python’s built-in `datetime` module has a `tzinfo` class that serves as a base for working with time zones. However, this is abstract and is more of a foundation for timezone implementations rather than a complete solution.
```python from datetime import datetime, timedelta, timezone UTC = timezone.utc current_time_utc = datetime.now(UTC) ```
- The `pytz` library**: For comprehensive timezone support, the third-party `pytz` library is highly recommended. It provides an extensive list of time zones and handles daylight saving time intricacies.
```python import pytz local_timezone = pytz.timezone("Europe/Berlin") local_time = datetime.now(local_timezone) ```
- Conversion Between Time Zones**: With `pytz`, it’s simple to convert between time zones.
```python pacific_time = local_time.astimezone(pytz.timezone("US/Pacific")) ```
- Naive vs Aware DateTimes: In Python, a datetime object can be “naive” (without timezone information) or “aware” (with timezone information). Always be certain about which type you’re dealing with. Using `pytz`, you can localize a naive datetime, transforming it into an aware one.
```python naive_utc_time = datetime.utcnow() aware_utc_time = pytz.utc.localize(naive_utc_time) ```
- Handling Daylight Saving Time: Time zones are not just offsets from UTC; they have rules about daylight saving time. `pytz` can handle these changes, so when doing arithmetic with datetimes, the transitions are managed correctly.
- Best Practices:
– Store Datetimes in UTC: In databases and internal systems, always save datetimes in UTC and convert to the desired timezone only when presenting to the user.
– Be Cautious with Arithmetic: When adding or subtracting time from datetimes, especially around daylight saving transitions, use timezone-aware datetimes.
While Python provides foundational tools for time zone handling in its standard library, using `pytz` is advised for complete and accurate timezone operations. Always be conscious of the type of datetime you’re dealing with and strive for consistent practices in your applications.
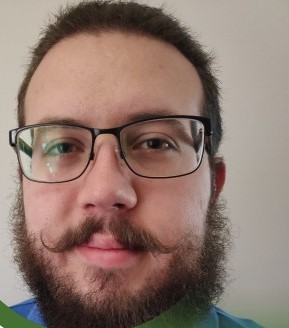
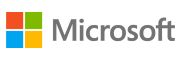