React Native and Augmented Reality: Building AR Experiences
In the ever-evolving landscape of technology, the fusion of React Native and Augmented Reality (AR) has given birth to a new realm of possibilities. Augmented Reality, the technology that overlays digital information onto the real world, has captured the imagination of developers and users alike. React Native, on the other hand, has simplified cross-platform app development, enabling developers to build efficient and powerful applications using a single codebase. The convergence of these two technologies opens up a whole new dimension of creativity, enabling developers to craft immersive and interactive AR experiences that were once considered the stuff of science fiction.
Table of Contents
1. Understanding Augmented Reality
Before we dive into the amalgamation of React Native and AR, let’s have a quick overview of Augmented Reality itself. Augmented Reality enhances our perception of the physical world by overlaying digital elements such as images, videos, 3D models, or data onto the real environment. Unlike Virtual Reality (VR), which creates an entirely digital environment, AR supplements the real world with computer-generated information. This technology has found applications in various fields, from gaming and entertainment to education and industrial training.
2. The Role of React Native in AR
React Native, the open-source framework developed by Facebook, has revolutionized the way we create mobile applications. It allows developers to build cross-platform apps using a single codebase, saving time and effort. When it comes to AR, React Native offers a streamlined approach to creating AR experiences that can run seamlessly on both iOS and Android devices. This means that developers familiar with React Native can leverage their existing skills to delve into the world of AR without having to learn an entirely new set of tools and languages.
3. Setting Up Your Project
To get started with building AR experiences using React Native, you’ll need a few tools and libraries. One of the most essential libraries for AR development is react-native-arkit for iOS or react-native-arcore for Android, depending on your target platform. These libraries provide the necessary components and APIs to interact with the AR capabilities of the devices.
Here’s how you can set up a new React Native project with AR support:
Step 1: Create a new React Native project
bash npx react-native init ARApp cd ARApp
Step 2: Install the AR library for your platform
For iOS (using react-native-arkit):
bash npm install react-native-arkit
For Android (using react-native-arcore):
bash npm install react-native-arcore
Step 3: Link the library to your project
bash npx react-native link react-native-arcore # or npx react-native link react-native-arcore
4. Building Your First AR Component
With your project set up, it’s time to create your first AR component. Let’s start by rendering a simple AR view with a floating object. In this example, we’ll use the ViroARPlaneSelector component from the react-native-arcore library for Android.
Step 1: Import the necessary components
javascript import React, { Component } from 'react'; import { ViroARScene, ViroARPlaneSelector } from 'react-native-arcore'; import { ViroBox } from 'react-viro';
Step 2: Create your AR scene
javascript class ARScene extends Component { render() { return ( <ViroARScene> <ViroARPlaneSelector> <ViroBox position={[0, 0.5, -1]} scale={[0.1, 0.1, 0.1]} /> </ViroARPlaneSelector> </ViroARScene> ); } }
Step 3: Render your AR scene
javascript export default class App extends Component { render() { return ( <ViroARSceneNavigator initialScene={{ scene: ARScene }} /> ); } }
In this code, we’re creating an AR scene using ViroARScene, and within that scene, we’re using ViroARPlaneSelector to place a 3D box object on a detected plane. This allows the object to appear as if it’s a part of the real world.
5. Interacting with AR Objects
Creating static AR scenes is just the tip of the iceberg. To truly leverage the power of React Native and AR, we need to make these experiences interactive. Let’s explore how we can enable user interactions with AR objects.
Step 1: Add state for object manipulation
javascript class ARScene extends Component { constructor() { super(); this.state = { selectedObject: null, }; } render() { return ( // ... ); } }
Step 2: Implement object selection and manipulation
javascript <ViroARScene> <ViroARPlaneSelector onPlaneSelected={() => this.setState({ selectedObject: 'box' })}> <ViroBox position={[0, 0.5, -1]} scale={this.state.selectedObject === 'box' ? [0.15, 0.15, 0.15] : [0.1, 0.1, 0.1]} onClick={() => this.setState({ selectedObject: 'box' })} /> </ViroARPlaneSelector> </ViroARScene>
In this code, we’re enabling the selection and manipulation of the 3D box object. When a detected plane is selected, the box scales up, giving the illusion of being picked up. Additionally, the user can tap on the box to select and deselect it.
6. Adding Realism with Environmental Understanding
To make AR experiences more immersive, it’s important to take into account the environment in which the AR objects are placed. This involves understanding the physical surroundings and integrating the AR elements accordingly.
Step 1: Access environmental data
javascript <ViroARPlaneSelector onPlaneSelected={(data) => this.handlePlaneSelected(data)}> {/* ... */} </ViroARPlaneSelector>
Step 2: Adjust object placement based on environmental data
javascript handlePlaneSelected(data) { const { position, rotation } = data; this.setState({ selectedObject: 'box', objectPosition: position, objectRotation: rotation, }); }
By using environmental data provided by the onPlaneSelected callback, we can position and orient AR objects in a way that seamlessly integrates with the physical space.
Conclusion
The combination of React Native and Augmented Reality is an exciting frontier for developers. It allows for the creation of captivating and interactive experiences that blur the lines between the real and digital worlds. With the power of React Native’s cross-platform capabilities and the immersive nature of AR, developers can build applications that provide users with unique and engaging interactions. As you embark on your journey into this dynamic realm, remember that experimentation and creativity are your best allies. Happy coding, and may your AR endeavors be truly extraordinary!
Table of Contents
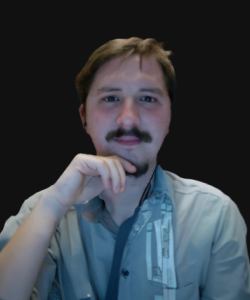
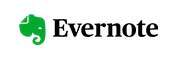