React Native and Bluetooth: Connecting Devices to Your Mobile App
In today’s digital age, mobile applications have become an integral part of our lives. From fitness trackers to smart home devices, the ability to connect external devices to a mobile app enhances user experiences significantly. React Native, a popular framework for building cross-platform mobile apps, provides developers with the tools to create dynamic applications that can seamlessly communicate with Bluetooth-enabled devices. In this article, we’ll delve into the world of React Native and Bluetooth connectivity, exploring how to connect various devices to your mobile app while ensuring a smooth and engaging user experience.
Table of Contents
1. Understanding React Native and Bluetooth Integration:
1.1. What is React Native?
React Native is an open-source framework developed by Facebook that allows developers to build native mobile applications using JavaScript and React. It enables you to create apps for multiple platforms, such as iOS and Android, using a single codebase. This cross-platform capability makes React Native a popular choice among developers aiming to streamline their development process.
1.2. The Power of Bluetooth Connectivity
Bluetooth technology has revolutionized the way devices communicate with each other. From wireless headphones to IoT devices, Bluetooth enables seamless connections without the hassle of cables. Integrating Bluetooth connectivity into your React Native app opens doors to a wide range of possibilities, from controlling smart devices to transmitting health data in real time.
2. Prerequisites for Bluetooth Integration:
2.1. Setting Up Your Development Environment
Before diving into Bluetooth integration, ensure that you have a working React Native development environment. You’ll need Node.js, npm (Node Package Manager), and the React Native CLI (Command Line Interface) installed. You can set up a new project using the following commands:
bash npx react-native init BluetoothApp cd BluetoothApp
2.2. Understanding Bluetooth Technology Basics
Bluetooth operates on a wireless communication protocol that enables short-range data exchange between devices. Devices can communicate using different Bluetooth profiles, such as A2DP for audio streaming and GATT (Generic Attribute Profile) for exchanging data. Understanding these profiles is crucial for developing effective Bluetooth-enabled apps.
3. Scanning for Bluetooth Devices:
3.1. Requesting Bluetooth Permissions
In Android and iOS, Bluetooth permissions are required to scan for nearby devices. In your React Native app, you’ll need to request these permissions using libraries like react-native-permissions or the built-in permissions system.
javascript import { Platform } from 'react-native'; import { request, PERMISSIONS } from 'react-native-permissions'; const requestBluetoothPermission = async () => { const permission = Platform.OS === 'android' ? PERMISSIONS.ANDROID.BLUETOOTH_ADMIN : PERMISSIONS.IOS.BLUETOOTH_PERIPHERAL; const result = await request(permission); if (result === 'granted') { // Permission granted, start scanning for devices } else { // Permission denied, handle accordingly } };
3.2. Discovering Nearby Devices
Once you have the necessary permissions, you can initiate the process of scanning for nearby Bluetooth devices using the react-native-ble-plx library.
javascript import BleManager from 'react-native-ble-manager'; const startDeviceScan = () => { BleManager.scan([], 5, true) .then(() => { console.log('Scanning...'); }) .catch(error => { console.error('Scan error:', error); }); };
3.3. Displaying Device Information
As the scan identifies nearby devices, you can retrieve and display relevant information about these devices in your app’s user interface.
javascript BleManager.start({ showAlert: false }); BleManager.addListener('BleManagerDiscoverPeripheral', peripheral => { console.log('Discovered device:', peripheral); // Update UI with discovered device information });
4. Establishing a Connection:
4.1. Pairing and Bonding Devices
Pairing and bonding are processes that establish a secure connection between devices. Pairing involves creating a link between devices, while bonding stores the connection information for future use.
javascript BleManager.connect(peripheralId) .then(() => { console.log('Connected to device:', peripheralId); // Perform actions with the connected device }) .catch(error => { console.error('Connection error:', error); });
4.2. Handling Connection States
Managing connection states is essential for providing users with real-time feedback about their interactions with Bluetooth devices.
javascript BleManager.addListener('BleManagerConnectPeripheral', peripheral => { console.log('Connected to:', peripheral); }); BleManager.addListener('BleManagerDisconnectPeripheral', peripheral => { console.log('Disconnected from:', peripheral); });
4.3. Error Handling for Connection Failures
Bluetooth connections can fail due to various reasons, such as device out of range or connection timeout. Implement robust error handling to provide users with meaningful feedback.
javascript BleManager.connect(peripheralId) .then(() => { // Successful connection }) .catch(error => { console.error('Connection error:', error); if (error.errorCode === 'PeripheralDisconnected') { console.log('Device disconnected.'); } else { console.log('Connection failed for an unknown reason.'); } });
5. Exchanging Data Between Devices:
5.1. Sending and Receiving Data
Once a connection is established, you can exchange data between your React Native app and the connected Bluetooth device.
javascript const data = 'Hello, Bluetooth Device!'; BleManager.write(peripheralId, serviceUUID, characteristicUUID, data) .then(() => { console.log('Data sent:', data); }) .catch(error => { console.error('Write error:', error); });
5.2. Implementing Data Protocols
Define data protocols that both your app and the Bluetooth device understand. This ensures seamless communication and prevents data misinterpretation.
javascript const encodeData = (data) => { // Convert data to a format suitable for transmission return data; }; const decodeData = (encodedData) => { // Convert received data to its original format return encodedData; };
5.3. Real-time Data Synchronization
For applications that require real-time data synchronization, consider implementing a notification mechanism that allows the Bluetooth device to notify your app when new data is available.
javascript BleManager.startNotification(peripheralId, serviceUUID, characteristicUUID) .then(() => { console.log('Started notifications.'); }) .catch(error => { console.error('Notification error:', error); }); BleManager.addListener('BleManagerDidUpdateValueForCharacteristic', data => { console.log('Received data:', decodeData(data.value)); // Update UI with received data });
6. Best Practices for a Seamless Experience:
6.1. Optimizing Connection Speed and Stability
Minimize connection latency by optimizing your app’s code and minimizing unnecessary data transfers. Implement reconnection mechanisms to handle temporary connection losses.
6.2. Ensuring Data Security and Privacy
Bluetooth data transmissions can be vulnerable to interception. Implement encryption and authentication mechanisms to ensure the security and privacy of exchanged data.
6.3. Handling Background Tasks and Interruptions
Bluetooth functionality should work seamlessly even when the app is in the background or interrupted by phone calls. Implement background tasks and proper state management to ensure a smooth user experience.
7. Case Study: Building a Bluetooth-enabled React Native App:
7.1. Defining App Requirements
Let’s consider a fitness tracking app that connects to a heart rate monitor via Bluetooth. The app should display real-time heart rate data and store historical data for analysis.
7.2. Architecting the App for Bluetooth Integration
Organize your app’s components and screens in a way that facilitates Bluetooth integration. Separate concerns, and create dedicated modules for Bluetooth-related functionalities.
7.3. Writing and Testing Bluetooth-related Code
Follow the code examples and guidelines provided earlier to implement Bluetooth functionality in your fitness tracking app. Test the app thoroughly on different devices and scenarios to ensure reliability.
Conclusion
The marriage of React Native and Bluetooth technology opens up a realm of possibilities for mobile app developers. Whether you’re building a smart home control app, a fitness tracker, or an IoT management tool, the ability to seamlessly connect devices to your app enhances user engagement and utility. By understanding the fundamentals of Bluetooth integration, managing connection states, and exchanging data effectively, you can create robust and user-friendly applications that harness the power of wireless communication. As you embark on your journey to connect devices to your React Native app via Bluetooth, remember to adhere to best practices for security, privacy, and a seamless user experience. With the right knowledge and tools, you can create innovative and dynamic apps that bring the physical and digital worlds together.
Table of Contents
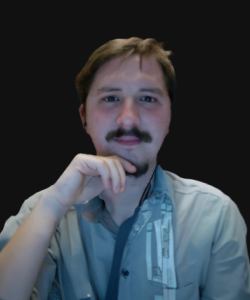
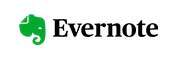