Building Barcode Scanning in React Native Apps
In today’s digital age, efficiency is key, especially for businesses. One of the most powerful tools for streamlining operations is barcode scanning. With the rise of mobile applications, integrating barcode scanning into React Native apps has become essential for businesses looking to enhance productivity and accuracy. In this guide, we’ll walk you through the process of building barcode scanning functionality into your React Native apps, complete with examples and best practices.
Why Barcode Scanning?
Barcode scanning offers numerous benefits for businesses, including:
- Efficiency: Barcode scanning automates data entry processes, reducing the need for manual input and minimizing errors.
- Accuracy: By scanning barcodes, businesses can ensure accurate data capture, leading to better inventory management and order fulfillment.
- Cost-Effectiveness: Implementing barcode scanning in React Native apps eliminates the need for specialized hardware, saving businesses both time and money.
Getting Started
To begin building barcode scanning functionality in your React Native app, you’ll need to install the necessary dependencies. One popular library for barcode scanning is react-native-camera, which provides support for both iOS and Android platforms.
npm install react-native-camera --save
Once installed, you’ll need to link the library to your project using either react-native link or manual linking, depending on your React Native version.
Implementing Barcode Scanning
Now that you have the necessary dependencies installed, let’s dive into implementing barcode scanning in your React Native app.
1. Initialize Camera Component
Start by adding a camera component to your app’s UI. You can use the RNCamera component provided by react-native-camera.
import { RNCamera } from 'react-native-camera'; <RNCamera style={{ flex: 1 }} type={RNCamera.Constants.Type.back} onBarCodeRead={(barcode) => console.log(barcode)} />
2. Handle Barcode Scans
Utilize the onBarCodeRead callback to capture barcode data when a barcode is scanned. You can then perform actions such as parsing the barcode data and updating your app’s state or sending the data to a backend server.
onBarCodeRead={(barcode) => { console.log(barcode.data); // Perform additional actions with barcode data }}
3. Styling and Customization
Customize the appearance and behavior of the camera component to fit your app’s design and requirements. You can adjust properties such as camera type, barcode types to scan, and styling options.
<RNCamera style={styles.camera} type={RNCamera.Constants.Type.back} flashMode={RNCamera.Constants.FlashMode.auto} onBarCodeRead={(barcode) => console.log(barcode)} barCodeTypes={[RNCamera.Constants.BarCodeType.qr]} />
Best Practices
To ensure a seamless user experience and optimal performance, consider the following best practices when implementing barcode scanning in your React Native app:
- Optimize Camera Settings: Adjust camera settings such as resolution and focus mode to balance performance and accuracy.
- Handle Permissions: Request necessary permissions (e.g., camera access) from users before enabling barcode scanning.
- Error Handling: Implement robust error handling to gracefully handle scenarios such as camera permissions denied or barcode scanning failures.
- Testing: Thoroughly test barcode scanning functionality across different devices and scenarios to identify and address any issues.
Conclusion
Integrating barcode scanning into your React Native app can significantly improve operational efficiency and accuracy for your business. By following the steps outlined in this guide and incorporating best practices, you can seamlessly incorporate barcode scanning functionality into your app and unlock its full potential.
For further reading and advanced topics on barcode scanning in React Native apps, check out the following resources:
- React Native Camera Documentation
- Barcode Scanning with React Native: A Complete Guide
- Building a Barcode Scanner in React Native
Happy scanning!
Remember, the key to success lies in continuous learning and experimentation. Embrace the journey of building innovative solutions with React Native and barcode scanning!
Table of Contents
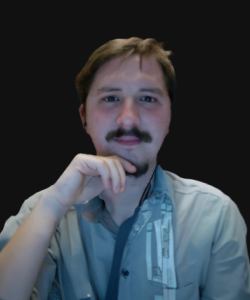
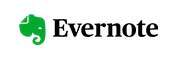