Building PDF Generation in React Native Apps
In the ever-evolving world of mobile app development, there’s a constant demand for features that enhance user experience and streamline business processes. One such feature is the ability to generate PDFs within your React Native application. Whether you need to generate invoices, reports, or any other type of document, having the capability to create PDFs on the fly can be incredibly valuable. In this blog post, we’ll walk you through the process of building PDF generation functionality in your React Native app, step by step.
Table of Contents
1. Why Generate PDFs in React Native?
Before diving into the technical details, let’s first understand why you might need to generate PDFs in your React Native app:
1.1. Document Generation:
Create invoices, receipts, or bills for your e-commerce app.
Generate reports or summaries for data analysis and business insights.
Produce certificates, tickets, or boarding passes for event apps.
1.2. Offline Access:
Allow users to download and view content offline in a standardized format.
Ensure that important information is accessible even without an internet connection.
1.3. Data Export:
Enable users to export app data and content for sharing or archiving purposes.
Enhance data portability and compatibility with other systems.
Now that you understand the potential benefits, let’s proceed with building PDF generation in your React Native app.
2. Getting Started
2.1. Setting Up Your React Native Project:
If you haven’t already, create a new React Native project or use an existing one. You can set up a new project using the following command:
bash npx react-native init YourProjectName
2.2. Choosing a PDF Generation Library:
To generate PDFs in React Native, we have a few libraries to choose from. Some popular options include:
- react-native-pdf: A widely-used library that allows you to display and create PDFs.
- react-native-pdf-lib: A library for creating and manipulating PDF documents.
- react-native-html-to-pdf: A library for converting HTML content to PDF format.
In this tutorial, we’ll use the react-native-pdf-lib library. You can install it in your project with the following command:
bash npm install react-native-pdf-lib
2.3. Link the Library:
After installation, you need to link the library to your project. Run the following command:
bash npx react-native link react-native-pdf-lib
With the initial setup complete, let’s move on to the steps required to generate PDFs in your React Native app.
3. Step-by-Step Guide to PDF Generation
3.1. Import the Necessary Modules:
First, import the required modules from the react-native-pdf-lib library:
javascript import { PDFDocument, rgb } from 'react-native-pdf-lib';
3.2. Create a Function for PDF Generation:
Next, create a function that will handle the PDF generation process. For example:
javascript const generatePDF = async () => { const pdfDoc = await PDFDocument.create(); // Add a page to the PDF const page = pdfDoc.addPage([300, 400]); // Draw text on the page page.drawText('Hello, React Native PDF!', { x: 50, y: 350, size: 30, color: rgb(0, 0, 0), }); // Save the PDF to a file or return it as a base64 string const pdfBytes = await pdfDoc.save(); // For example, save the PDF to a file const pdfPath = `${RNFS.DocumentDirectoryPath}/example.pdf`; await RNFS.writeFile(pdfPath, pdfBytes, 'base64'); console.log(`PDF saved to: ${pdfPath}`); };
In this example, we’re creating a simple PDF document with one page and adding text to it. You can customize the content and layout as needed for your specific use case.
3.3. Call the PDF Generation Function:
You can trigger the PDF generation function in response to user actions, such as a button click or form submission. For instance:
javascript <button onPress={generatePDF}>Generate PDF</button>
3.4. Testing Your PDF Generation:
To test your PDF generation functionality, run your React Native app on an emulator or a physical device. When you trigger the PDF generation function, it should create a PDF file with the specified content.
4. Advanced PDF Generation
4.1. Adding Images:
To include images in your PDF, you can use the drawImage method. Here’s an example:
javascript const image = await pdfDoc.embedPng('data:image/png;base64,...'); page.drawImage(image, { x: 50, y: 200, width: 200, height: 100, });
4.2. Styling Text:
You can style text using different fonts, sizes, and colors. Here’s how you can specify font and size:
javascript page.drawText('Styled Text', { x: 50, y: 300, size: 20, font: await pdfDoc.embedFont('data:application/x-font-ttf;base64,...'), color: rgb(255, 0, 0), });
4.3. Multiple Pages:
For multi-page documents, simply add more pages to the PDF document and customize each page as needed:
javascript const page1 = pdfDoc.addPage([300, 400]); const page2 = pdfDoc.addPage([300, 400]); // Customize page content for page1 and page2
4.4. Saving and Sharing:
You can save the generated PDF to the device’s storage and provide options for sharing it with other apps. To share a PDF, you can use libraries like react-native-share or react-native-fs.
Conclusion
Adding PDF generation capabilities to your React Native app opens up a world of possibilities for document management, data export, and offline access. With the react-native-pdf-lib library, you have a powerful tool at your disposal for creating customized PDFs tailored to your app’s needs.
This tutorial has walked you through the process of setting up your project, choosing a PDF generation library, and creating PDFs step by step. Remember that PDF generation can be as simple or as complex as your project requires, so don’t hesitate to explore the library’s documentation for more advanced features and customization options.
Now, go ahead and empower your React Native app with the ability to generate beautiful and functional PDF documents, taking your user experience to the next level. Happy coding!
Table of Contents
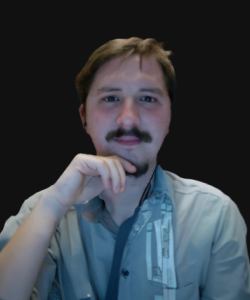
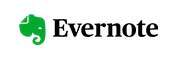