React Native and Amazon AWS: Integrating Cloud Services in Apps
In today’s fast-paced digital landscape, mobile app developers are constantly seeking ways to enhance the functionality and capabilities of their applications. One effective strategy to achieve this is by integrating cloud services into your React Native apps. Among the myriad of cloud providers available, Amazon Web Services (AWS) stands out as a powerful and versatile choice. In this comprehensive guide, we will explore how to seamlessly integrate Amazon AWS services into your React Native app, opening doors to cloud-powered features that can transform your application’s performance and user experience.
Table of Contents
1. Why Integrate Amazon AWS with React Native?
Before delving into the technical aspects of integrating AWS with React Native, let’s understand why this combination is a game-changer for mobile app development.
1.1. Scalability
Amazon AWS offers a wide range of scalable services that can accommodate your app’s growth. Whether you’re dealing with a small user base or expecting exponential growth, AWS can scale to meet your needs without compromising performance.
1.2. Reliability
AWS is renowned for its robust infrastructure and high availability. Your app can benefit from AWS’s reliability, ensuring minimal downtime and a smooth user experience.
1.3. Cost-Efficiency
AWS provides a pay-as-you-go pricing model, allowing you to pay only for the resources you use. This cost-efficient approach is particularly attractive for startups and small businesses.
1.4. Versatility
AWS offers a vast ecosystem of services, including storage, databases, AI/ML, serverless computing, and more. This versatility enables you to implement a wide range of features and functionalities in your app.
Now that we understand the advantages of integrating AWS with React Native, let’s dive into the practical steps to achieve this integration.
2. Setting Up Your AWS Account
To get started, you need an AWS account. If you don’t already have one, follow these steps:
2.1. Sign Up for AWS
Visit the AWS website and click on the “Create an AWS Account” button. Follow the registration process, providing the necessary information and payment details.
2.2. Access the AWS Management Console
Once your account is set up, log in to the AWS Management Console. This is your dashboard for managing AWS resources and services.
2.3. Create an IAM User
To securely access AWS resources from your React Native app, it’s essential to create an IAM (Identity and Access Management) user with the appropriate permissions. Here’s how:
2.3.1. Navigate to IAM
In the AWS Management Console, search for “IAM” and click on the “IAM” service.
2.3.2. Create a New User
Click on “Users” in the left navigation pane and then click “Add user.”
2.3.3. Configure User Details
Enter a username for your IAM user and choose the access type. For programmatic access (which is suitable for React Native apps), select “Programmatic access.”
2.3.4. Set Permissions
In the “Set permissions” step, you can either attach existing policies or create custom policies to grant specific permissions to your IAM user. For simplicity, you can start by attaching the “AmazonS3FullAccess” policy if you plan to work with Amazon S3, a popular AWS storage service.
2.3.5. Review and Create User
Review your user’s details and permissions, then click “Create user.”
2.3.6. Save Access Key
Once the user is created, make sure to save the access key ID and secret access key. You will need these credentials to authenticate your React Native app with AWS.
3. Integrating AWS Services with React Native
Now that you have your AWS account set up and an IAM user with appropriate permissions, let’s explore how to integrate AWS services into your React Native app.
3.1. Installing AWS Amplify
AWS Amplify is a set of libraries and tools that simplifies the process of integrating AWS services into your mobile app. To get started, you need to install Amplify:
bash npm install -g @aws-amplify/cli
3.2. Configuring Amplify
Once Amplify is installed, configure it to use your AWS account:
bash amplify configure
Follow the prompts to sign in with your AWS IAM user and set up your default AWS region.
3.3. Initializing Your React Native Project
If you haven’t already created a React Native project, do so using the following command:
bash npx react-native init MyAWSApp
Navigate to your project directory:
bash cd MyAWSApp
3.4. Adding Amplify to Your Project
To add Amplify to your React Native project, run the following command:
bash amplify init
This command will guide you through configuring your project with Amplify. Be sure to select the AWS profile corresponding to the IAM user you created earlier.
3.5. Adding AWS Services
With Amplify integrated into your project, you can easily add AWS services. For example, to add Amazon S3 for storing and retrieving files, use the following command:
bash amplify add storage
Follow the prompts to configure your storage options. Amplify will generate the necessary code to interact with your chosen AWS service.
3.6. Building and Deploying
After adding AWS services, it’s time to build and deploy your React Native app with Amplify:
bash npm run build amplify push
This will deploy your app and the associated AWS resources to the cloud.
3.7. Accessing AWS Services in React Native
Now that your app is connected to AWS, you can access AWS services from your React Native code. Here’s an example of how to upload a file to Amazon S3:
javascript import { Storage } from 'aws-amplify'; async function uploadFile() { try { const result = await Storage.put('myFile.jpg', file, { level: 'public', }); console.log('File uploaded successfully:', result.key); } catch (error) { console.error('Error uploading file:', error); } }
In this example, we’re using the Amplify Storage library to upload a file to an S3 bucket. You can similarly interact with other AWS services like DynamoDB, Lambda, or Cognito in your React Native app.
4. Common AWS Services for React Native Apps
Here are some AWS services that can greatly enhance the functionality of your React Native app:
4.1. Amazon S3 (Simple Storage Service)
Amazon S3 provides scalable and secure object storage. Use it for storing user-generated content, media files, or any data that needs to be persisted.
4.2. Amazon DynamoDB
DynamoDB is a fully managed NoSQL database service that offers low-latency, high-performance data storage. It’s an excellent choice for storing app data and user profiles.
4.3. AWS Lambda
AWS Lambda allows you to run serverless functions in response to various events. You can use Lambda functions to add serverless compute capabilities to your app.
4.4. Amazon Cognito
Cognito provides authentication, authorization, and user management services. Securely manage user identities and access control in your app.
4.5. AWS AppSync
AppSync is a managed service that simplifies the process of building GraphQL APIs. It’s a great choice if you want to implement real-time data synchronization in your app.
5. Best Practices for AWS Integration in React Native
While integrating AWS services with React Native, it’s essential to follow best practices to ensure your app’s performance, security, and scalability.
5.1. Secure Credentials
Always store AWS credentials securely. Never hardcode them in your app’s source code or expose them publicly.
5.2. Implement Authorization
Use AWS IAM roles and policies to restrict access to AWS resources based on user permissions.
5.3. Monitor and Optimize
Leverage AWS monitoring and optimization tools to ensure cost-efficiency and performance. AWS CloudWatch and AWS Trusted Advisor can help you keep an eye on resource usage and identify cost-saving opportunities.
5.4. Backup and Recovery
Implement backup and recovery strategies for critical data stored in AWS. Regularly back up your data and test your recovery procedures.
Conclusion
Integrating Amazon AWS services into your React Native app can significantly enhance its capabilities, scalability, and performance. With the power of AWS at your fingertips, you can build feature-rich and reliable mobile applications that cater to the demands of today’s users. By following the steps outlined in this guide and adhering to best practices, you can harness the full potential of cloud computing for your React Native projects. So, go ahead and unlock the limitless possibilities of AWS in your mobile app development journey!
Table of Contents
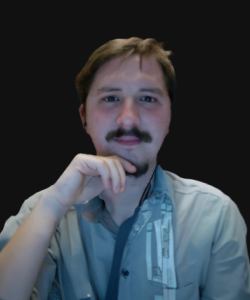
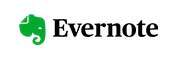