React Native and Expo Push Notifications: Engaging Users on Mobile
Mobile apps have become an integral part of our daily lives, offering convenience, entertainment, and valuable services. However, with millions of apps available in app stores, it’s essential to keep users engaged with your app to ensure its success. One powerful tool for achieving this is push notifications. In this blog, we will explore how to implement push notifications in React Native apps using Expo, enhancing user engagement and retention.
Table of Contents
1. Understanding the Importance of Push Notifications
1.1. Why Are Push Notifications Crucial?
Push notifications are short messages sent to a user’s device by a mobile app, even when the app is not actively in use. They serve as a direct communication channel between the app and its users. Here’s why push notifications are crucial for mobile app engagement:
- Real-Time Updates: Push notifications deliver real-time information and updates to users, keeping them informed about events, news, or changes within the app.
- Re-Engagement: They bring users back to your app, increasing the chances of users using your app more frequently.
- Enhanced User Experience: Push notifications can provide personalized and valuable content, enhancing the overall user experience.
- Retention: Engaged users are more likely to stick with your app over time, reducing churn rates.
1.2. The Impact on User Retention
User retention is a critical metric for the success of any mobile app. Push notifications play a significant role in improving user retention by keeping users engaged and active within your app. According to studies, apps that use push notifications effectively have up to 3 times higher user retention rates compared to those that do not.
Now that we understand the importance of push notifications, let’s dive into implementing them in your React Native app using Expo.
2. Setting Up Your Development Environment
Before we can start working with push notifications in a React Native app using Expo, we need to set up our development environment.
2.1. Installing Node.js and NPM
If you haven’t already, you need to install Node.js and NPM (Node Package Manager) on your system. You can download and install them from the official website: Node.js Download.
To check if Node.js and NPM are installed, open your terminal and run the following commands:
bash node -v npm -v
2.2. Creating a React Native Project with Expo
Expo is a framework that simplifies React Native development. Let’s create a new React Native project using Expo. Run the following command:
bash npm install -g expo-cli expo init MyPushNotificationApp
Follow the prompts to configure your project, and once it’s created, navigate to the project folder:
bash cd MyPushNotificationApp
Now, we are ready to add push notifications to our app.
3. Adding Expo Push Notifications to Your App
Expo provides a straightforward way to add push notifications to your app. Follow these steps to get started:
3.1. Setting Up Expo Notifications
First, we need to install the necessary packages for push notifications:
bash expo install expo-notifications
Next, import the Notifications module in your code:
javascript import * as Notifications from 'expo-notifications';
3.2. Requesting Permissions
Before sending push notifications to a user’s device, we must request the necessary permissions. Typically, you’d request permissions in a user-friendly way when the user first launches your app. Add the following code to request permissions:
javascript // Request permissions for push notifications async function registerForPushNotifications() { const { status } = await Notifications.requestPermissionsAsync(); if (status !== 'granted') { alert('Permission to receive push notifications denied!'); return; } }
3.3. Handling Notifications
Now that we have permissions, we can handle incoming push notifications. Expo allows you to listen for notifications and perform actions when they arrive. Add the following code to handle notifications:
javascript // Handle incoming push notifications Notifications.addNotificationReceivedListener(notification => { // Handle the notification here console.log('Notification received:', notification); }); Notifications.addNotificationResponseReceivedListener(response => { // Handle the user's response to the notification (e.g., navigate to a specific screen) console.log('Notification response received:', response); });
With these basic steps, your React Native app is now capable of receiving and handling push notifications. But what about customizing the content and appearance of your notifications?
4. Customizing Your Push Notifications
Customizing push notifications is essential to make them engaging and relevant to your users. Expo allows you to personalize your notifications in various ways.
4.1. Creating Engaging Content
You can customize the content of your push notifications by specifying a title and a body. Here’s an example:
javascript const notification = { title: 'New Message', body: 'You have a new message from John Doe.', };
This makes your notifications informative and attention-grabbing.
4.2. Using Custom Sounds and Icons
Expo also allows you to use custom sounds and icons for your notifications. To use a custom sound, specify the sound property in your notification object:
javascript const notification = { title: 'New Message', body: 'You have a new message from John Doe.', sound: 'custom-sound.mp3', // Place your custom sound file in the app's assets folder };
To use a custom icon, specify the android.channelId property in your notification object:
javascript const notification = { title: 'New Message', body: 'You have a new message from John Doe.', android: { channelId: 'custom-channel', // Create a custom channel with your icon }, };
Customizing the appearance and content of your push notifications can significantly impact user engagement.
5. Sending Push Notifications from Your Server
Now that your app can receive push notifications, you need a way to send them. Typically, you’ll have a server responsible for sending notifications to your users. Here’s how to set up a notification server and send notifications to specific users.
5.1. Setting Up a Notification Server
You can use various backend technologies to set up a notification server. One popular choice is Node.js with the expo-server-sdk package. Install it in your server project:
bash npm install expo-server-sdk
Now, let’s send a push notification from your server:
javascript const { Expo } = require('expo-server-sdk'); // Create a new Expo SDK client const expo = new Expo(); // An array of the user's Expo push tokens const pushTokens = ['ExpoPushToken1', 'ExpoPushToken2']; // The message to send const message = { title: 'New Update', body: 'Check out the latest features in our app!', }; // Construct the push notification const notifications = []; for (const pushToken of pushTokens) { if (!Expo.isExpoPushToken(pushToken)) { console.error(`Invalid push token: ${pushToken}`); continue; } notifications.push({ to: pushToken, sound: 'default', title: message.title, body: message.body, data: { /* optional data for your app */ }, }); } // Send the notifications const chunks = expo.chunkPushNotifications(notifications); (async () => { for (const chunk of chunks) { try { const ticketChunk = await expo.sendPushNotificationsAsync(chunk); console.log(ticketChunk); } catch (error) { console.error(error); } } })();
In this example, we construct the push notification, specifying the target user’s Expo push token. The expo-server-sdk simplifies the process of sending notifications to Expo’s servers, which then deliver the notifications to the user’s device.
6. Analyzing User Engagement
To measure the effectiveness of your push notifications and further enhance user engagement, it’s essential to analyze user interaction with notifications.
6.1. Tracking Notification Metrics
Expo provides tools for tracking notification metrics. You can measure the open rate, response rate, and other key metrics to assess how well your notifications are performing. This data helps you refine your notification strategy over time.
6.2. A/B Testing with Notifications
A/B testing allows you to experiment with different notification styles and content to determine which ones are most effective. You can use tools like Firebase A/B Testing or custom solutions to test variations of your notifications and see which ones lead to higher user engagement.
7. Best Practices for Push Notifications
While push notifications can be a powerful engagement tool, it’s crucial to follow best practices to avoid annoying users. Here are some tips:
7.1. Timing and Frequency
Send notifications at times when users are most likely to be active.
Avoid sending too many notifications in a short period to prevent user fatigue.
7.2. Personalization
Personalize notifications based on user behavior and preferences.
Segment your user base and send targeted notifications to specific groups.
7.3. A/B Testing
Continuously experiment and optimize your notification content and timing.
Monitor user feedback and adjust your strategy accordingly.
Conclusion
Push notifications are a valuable tool for engaging users in your React Native and Expo mobile apps. When used effectively, they can boost user retention, drive user engagement, and enhance the overall user experience. By following best practices and continuously analyzing user data, you can create a notification strategy that keeps your users coming back for more. Start implementing push notifications in your app today and watch your user engagement soar!
Table of Contents
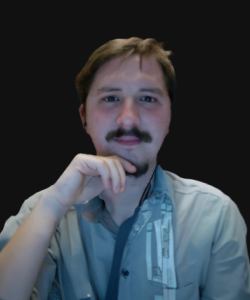
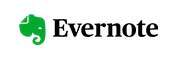