React Native and Microsoft Azure: Cloud Integration in Mobile Apps
In the ever-evolving landscape of mobile app development, delivering rich and feature-packed applications is a constant challenge. One solution to this challenge is the integration of cloud services into your mobile apps. Microsoft Azure, with its wide array of cloud offerings, can be a game-changer in this regard. In this blog post, we will explore how to harness the power of Microsoft Azure by integrating it with React Native, a popular framework for building cross-platform mobile apps.
Table of Contents
1. Why Integrate Azure with React Native?
Before we dive into the technical details, let’s take a moment to understand why you might want to integrate Microsoft Azure with your React Native app.
1.1. Scalability
Azure offers scalable solutions for a variety of scenarios. Whether your app experiences sudden spikes in traffic or needs to accommodate a growing user base, Azure’s scalability ensures your app can handle the load.
1.2. Storage
With Azure Blob Storage and Azure SQL Database, you can efficiently store and manage your app’s data. This allows you to focus on building great user experiences while Azure takes care of data storage.
1.3. Authentication and Authorization
Azure Active Directory provides robust authentication and authorization capabilities, ensuring that only authorized users can access your app’s resources. This is crucial for securing your app’s data and functionality.
1.4. AI and Machine Learning
Azure provides AI and machine learning services that can be seamlessly integrated into your React Native app. You can incorporate features like image recognition, natural language processing, and predictive analytics.
Now that we’ve established the benefits of integrating Azure with React Native, let’s walk through some key integration scenarios.
2. Setting Up Your React Native Project
Before you can start integrating Azure services, you need a React Native project up and running. If you’re new to React Native, you can set up a basic project using the following commands:
bash npx react-native init MyApp cd MyApp npx react-native run-android
Make sure you have Node.js and npm (Node Package Manager) installed on your machine before running these commands.
3. Azure Integration Scenarios
Now that you have your React Native project ready, let’s explore some common Azure integration scenarios.
3.1. Azure Functions for Backend Logic
Azure Functions are serverless compute services that allow you to run code in response to various triggers. You can use Azure Functions to build the backend logic for your React Native app. Here’s a simple example of how to create an Azure Function in JavaScript and integrate it with your app.
- Create an Azure Function
- Navigate to the Azure portal (https://portal.azure.com).
- Click on “Create a resource” and search for “Function App.”
- Follow the steps to create a new Function App.
- Once your Function App is created, go to the “Functions” section and click “Add.”
3.1.1. Writing an Azure Function
Here’s an example of a simple Azure Function written in JavaScript that returns a “Hello, Azure!” message:
javascript module.exports = async function (context, req) { context.res = { body: "Hello, Azure!" }; };
3.1.2. Calling the Azure Function from React Native
In your React Native app, you can use the fetch API to call the Azure Function:
javascript fetch('https://your-function-app-url.azurewebsites.net/api/your-function-name') .then((response) => response.json()) .then((data) => { console.log(data.body); // "Hello, Azure!" }) .catch((error) => { console.error(error); });
3.2. Azure Cosmos DB for Data Storage
Azure Cosmos DB is a globally distributed, multi-model database service that can be a perfect fit for storing your app’s data. Here’s how you can use Azure Cosmos DB with React Native.
3.2.1. Create an Azure Cosmos DB
- In the Azure portal, click “Create a resource” and search for “Azure Cosmos DB.”
- Follow the steps to create a new Azure Cosmos DB account.
- Using Azure Cosmos DB in React Native
- You can use the official Azure Cosmos DB SDK for JavaScript to interact with your database from your React Native app. Here’s an example of how to insert data into a Cosmos DB container:
javascript const { CosmosClient } = require("@azure/cosmos"); const client = new CosmosClient({ endpoint: "your-cosmos-db-endpoint", key: "your-cosmos-db-key" }); const databaseId = "YourDatabaseId"; const containerId = "YourContainerId"; const container = client.database(databaseId).container(containerId); const newItem = { id: "1", name: "Sample Item" }; const { resource: createdItem } = await container.items.create(newItem); console.log(`Created item with id: ${createdItem.id}`);
3.3. Azure App Service for Hosting
Azure App Service allows you to host your React Native app’s backend, APIs, and even the frontend. You can easily deploy your app to Azure and scale it as needed.
- Deploy Your React Native App to Azure App Service
- Navigate to the Azure portal.
- Click on “Create a resource” and search for “Web App.”
- Follow the steps to create a new Web App.
- Once your Web App is created, you can deploy your React Native app using various methods, such as Azure DevOps, GitHub Actions, or manual deployment via FTP.
4. Authentication and Authorization with Azure AD
Securing your React Native app is paramount, and Azure Active Directory (Azure AD) can help you achieve this. Azure AD provides identity and access management services that you can integrate seamlessly into your app.
4.1. Configure Azure AD for React Native
- In the Azure portal, navigate to the “Azure Active Directory” section.
- Click on “App registrations” and create a new app registration.
- Configure the app registration with the necessary permissions and redirect URIs for your React Native app.
4.2. Implement Authentication
You can use libraries like react-native-app-auth or react-native-azure-ad to implement authentication with Azure AD in your React Native app. Here’s an example using react-native-app-auth:
javascript import { authorize, refresh, revoke } from 'react-native-app-auth'; const config = { clientId: 'your-client-id', redirectUrl: 'your-redirect-uri', scopes: ['openid', 'profile', 'offline_access'], serviceConfiguration: { authorizationEndpoint: 'https://login.microsoftonline.com/your-tenant-id/oauth2/v2.0/authorize', tokenEndpoint: 'https://login.microsoftonline.com/your-tenant-id/oauth2/v2.0/token', }, }; // Login const { accessToken, refreshToken } = await authorize(config); // Refresh token const newToken = await refresh(config, { refreshToken }); // Logout await revoke(config, { tokenToRevoke: accessToken });
5. Leveraging Azure AI and Machine Learning
Azure offers a wide range of AI and machine learning services that you can integrate into your React Native app to provide intelligent features.
5.1. Vision AI with Azure Cognitive Services
Azure Cognitive Services provide pre-built AI models for tasks like image recognition, object detection, and facial recognition. Here’s an example of using the Azure Cognitive Services API in React Native:
javascript const apiKey = 'your-cognitive-services-api-key'; const endpoint = 'your-cognitive-services-endpoint'; const imageUrl = 'https://example.com/image.jpg'; fetch(`${endpoint}/vision/v3.1/analyze?visualFeatures=Description`, { method: 'POST', headers: { 'Content-Type': 'application/json', 'Ocp-Apim-Subscription-Key': apiKey, }, body: JSON.stringify({ url: imageUrl }), }) .then((response) => response.json()) .then((data) => { console.log(data.description.captions[0].text); }) .catch((error) => { console.error(error); });
5.2. Language Processing with Azure Text Analytics
Azure Text Analytics allows you to perform sentiment analysis, language detection, and keyphrase extraction from text. Here’s an example of using Azure Text Analytics in React Native:
javascript const apiKey = 'your-text-analytics-api-key'; const endpoint = 'your-text-analytics-endpoint'; const text = 'This is a great product!'; fetch(`${endpoint}/text/analytics/v3.0/sentiment`, { method: 'POST', headers: { 'Content-Type': 'application/json', 'Ocp-Apim-Subscription-Key': apiKey, }, body: JSON.stringify({ documents: [{ id: '1', language: 'en', text }], }), }) .then((response) => response.json()) .then((data) => { console.log(`Sentiment: ${data.documents[0].sentiment}`); }) .catch((error) => { console.error(error); });
6. Best Practices for React Native and Azure Integration
As you embark on your journey to integrate React Native with Microsoft Azure, here are some best practices to keep in mind:
6.1. Security
- Always secure your API keys, secrets, and credentials. Consider using Azure Key Vault to manage sensitive information.
- Implement proper authentication and authorization mechanisms to protect your app’s resources.
6.2. Monitoring and Logging
- Set up monitoring and logging for your Azure resources and React Native app to quickly identify and resolve issues.
6.3. Scalability
- Design your app and Azure services with scalability in mind. Use Azure’s auto-scaling capabilities to handle traffic spikes.
6.4. Cost Management
- Monitor your Azure resource consumption and use Azure Cost Management tools to optimize costs.
6.5. Documentation
- Document your Azure integration thoroughly, including API endpoints, authentication methods, and data schemas.
Conclusion
Integrating React Native with Microsoft Azure can open up a world of possibilities for your mobile app. Whether you need serverless functions, data storage, authentication, or AI capabilities, Azure has you covered. By following best practices and leveraging Azure’s robust services, you can create powerful and feature-rich mobile apps that delight your users.
Remember that the landscape of technology is always evolving, so stay updated with the latest advancements in both React Native and Azure to keep your mobile app at the forefront of innovation. Happy coding!
Table of Contents
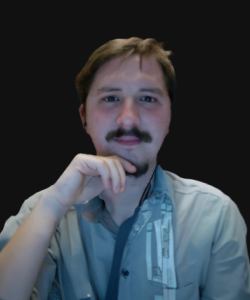
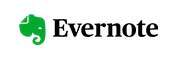