React Native and Facebook Login: Implementing Social Authentication
In today’s digital age, social authentication has become a cornerstone for user onboarding in mobile applications. React Native, with its ability to build cross-platform apps efficiently, offers seamless integration with various social login providers. One such provider is Facebook, offering a robust authentication mechanism that simplifies the user login process while enhancing app security. In this guide, we’ll explore how to implement Facebook login in React Native applications, empowering developers to leverage social authentication effectively.
Why Choose Facebook Login?
Before diving into implementation, let’s understand why integrating Facebook login is beneficial for React Native apps:
- Streamlined User Experience: Facebook login eliminates the need for users to create and remember new credentials, reducing friction during the signup process.
- Enhanced Security: Leveraging Facebook’s authentication infrastructure adds an extra layer of security to your application, guarding against unauthorized access and potential data breaches.
- Access to User Data: With user consent, Facebook login grants access to valuable user data, enabling personalized experiences and targeted marketing efforts.
Now, let’s delve into the implementation steps:
Step 1: Set Up Facebook Developer Account
To get started, create a Facebook Developer account and register your React Native application. Follow the official documentation provided by Facebook for detailed instructions on setting up your app credentials.
Step 2: Install Required Packages
In your React Native project directory, install the necessary packages using npm or yarn:
npm install @react-native-fbsdk/facebook
Or
yarn add @react-native-fbsdk/facebook
Step 3: Configure Android Manifest and Info.plist
For Android, update your android/app/src/main/AndroidManifest.xml file to include Facebook App ID and permissions. Similarly, for iOS, update your Info.plist file with the Facebook App ID.
Step 4: Implement Facebook Login Button
In your React Native component, import the necessary modules and add the Facebook login button:
import React from 'react'; import { LoginButton, AccessToken } from '@react-native-fbsdk/facebook'; const FacebookLoginButton = () => { const handleFacebookLogin = async () => { try { const result = await AccessToken.getCurrentAccessToken(); if (result) { // User is already logged in console.log('User is already logged in:', result.accessToken.toString()); } else { // Trigger Facebook login LoginManager.logInWithPermissions(['public_profile', 'email']).then( (loginResult) => { if (loginResult.isCancelled) { console.log('Facebook login cancelled'); } else { console.log('Facebook login success:', loginResult.grantedPermissions.toString()); } }, (error) => { console.log('Facebook login error:', error); } ); } } catch (error) { console.log('Error fetching Facebook access token:', error); } }; return <LoginButton onLoginFinished={() => handleFacebookLogin()} />; }; export default FacebookLoginButton;
Step 5: Handle Authentication Callback
Once the user completes the Facebook login flow, handle the authentication callback to retrieve the access token and user data. You can then use this information to authenticate the user within your application.
Step 6: Customize User Experience
Enhance the user experience by personalizing the app interface based on the user’s Facebook profile data. You can fetch additional user details such as name, email, profile picture, etc., and tailor the app content accordingly.
Conclusion
Integrating Facebook login into your React Native application offers numerous benefits, including simplified user onboarding, enhanced security, and access to valuable user data. By following the steps outlined in this guide, developers can seamlessly implement social authentication, providing users with a frictionless login experience while driving engagement and retention.
External Resources:
Table of Contents
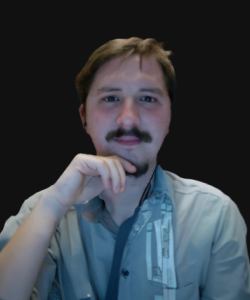
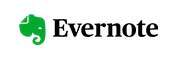