React Native and Firebase Cloud Firestore: Real-Time Data Syncing
In the world of mobile app development, user engagement and experience are paramount. Real-time data syncing is a key ingredient in creating responsive and dynamic applications. In this blog post, we’ll explore how to achieve real-time data syncing in your React Native app using Firebase Cloud Firestore.
Table of Contents
1. Why Real-Time Data Syncing Matters
Before we dive into the technical details, let’s understand why real-time data syncing is crucial for mobile apps.
1.1. Seamless User Experience
Imagine using a messaging app where you have to manually refresh the chat to see new messages. Frustrating, right? Real-time data syncing ensures that users receive updates instantly, providing a smooth and engaging experience.
1.2. Collaborative Features
Apps with collaborative features, such as collaborative document editing or real-time gaming, rely on instant data synchronization to maintain consistency across users.
1.3. Enhanced Productivity
For productivity apps like task managers or project management tools, real-time updates enable users to stay up-to-date with the latest changes, fostering productivity and collaboration.
2. Setting Up React Native with Firebase
Before we get into the real-time data syncing part, let’s set up a React Native project and integrate Firebase.
Step 1: Create a React Native Project
If you haven’t already set up React Native on your machine, you can follow the official documentation to get started: React Native Getting Started.
Once your project is set up, you can proceed to the next step.
Step 2: Set Up Firebase
Firebase provides a real-time database called Firestore, which we will use to achieve data syncing. To integrate Firebase into your React Native project, follow these steps:
Create a Firebase Project
- Go to the Firebase Console.
- Click on “Add Project” and follow the setup instructions.
Set Up Your App
After creating the project, click on “Add app” and select the appropriate platform (iOS/Android).
Follow the setup instructions, which typically involve adding configuration files to your project.
Install Firebase Dependencies
In your React Native project directory, install the Firebase dependencies:
bash npm install --save @react-native-firebase/app npm install --save @react-native-firebase/firestore
Initialize Firebase
Initialize Firebase in your app by adding the following code to your index.js or App.js file:
javascript import { AppRegistry } from 'react-native'; import { FirebaseAppProvider } from '@react-native-firebase/app'; import App from './App'; import { name as appName } from './app.json'; const firebaseConfig = { apiKey: '<your-api-key>', authDomain: '<your-auth-domain>', projectId: '<your-project-id>', storageBucket: '<your-storage-bucket>', messagingSenderId: '<your-messaging-sender-id>', appId: '<your-app-id>', }; const Main = () => ( <FirebaseAppProvider config={firebaseConfig}> <App /> </FirebaseAppProvider> ); AppRegistry.registerComponent(appName, () => Main);
Make sure to replace the placeholders (<your-api-key>, <your-auth-domain>, etc.) with your Firebase project’s credentials.
Now that we have Firebase integrated into our React Native app, let’s explore how to achieve real-time data syncing using Firestore.
3. Real-Time Data Syncing with Firebase Firestore
Firebase Firestore is a NoSQL cloud database that allows you to store and sync data in real-time. To demonstrate real-time data syncing, we’ll build a simple chat application.
Step 1: Create a Firestore Collection
In Firestore, data is organized into collections and documents. We’ll create a collection called “messages” to store our chat messages. Each message will be a document in this collection.
javascript import firestore from '@react-native-firebase/firestore'; // Create a reference to the "messages" collection const messagesRef = firestore().collection('messages');
Step 2: Add Messages to Firestore
To send a message, you can add a document to the “messages” collection. Each document should have a unique ID, which we can generate using firebase.firestore.FieldValue.serverTimestamp() to ensure chronological order.
javascript const sendMessage = async (text, sender) => { try { // Add a new document to the "messages" collection await messagesRef.add({ text, sender, timestamp: firestore.FieldValue.serverTimestamp(), }); } catch (error) { console.error('Error sending message: ', error); } };
Step 3: Real-Time Data Syncing
Now, let’s set up real-time data syncing to receive messages as they are added to the Firestore collection. We can use Firestore’s onSnapshot method to listen for changes.
javascript const subscribeToMessages = (callback) => { const unsubscribe = messagesRef .orderBy('timestamp') .onSnapshot((snapshot) => { const messages = []; snapshot.forEach((doc) => { messages.push({ id: doc.id, ...doc.data() }); }); callback(messages); }); return unsubscribe; };
In the code above, we order the messages by timestamp to ensure they are received in chronological order. The onSnapshot method triggers a callback whenever there are changes to the collection.
Step 4: Displaying Messages
Now that we’re receiving real-time updates, let’s display the messages in our chat interface.
javascript import React, { useEffect, useState } from 'react'; import { View, Text, TextInput, Button, FlatList } from 'react-native'; const ChatScreen = () => { const [messages, setMessages] = useState([]); const [text, setText] = useState(''); useEffect(() => { const unsubscribe = subscribeToMessages((newMessages) => { setMessages(newMessages); }); return () => unsubscribe(); }, []); const handleSend = () => { sendMessage(text, 'User'); setText(''); }; return ( <View> <FlatList data={messages} keyExtractor={(item) => item.id} renderItem={({ item }) => ( <View> <Text>{item.sender}: {item.text}</Text> </View> )} /> <View> <TextInput value={text} onChangeText={setText} placeholder="Type your message..." /> <Button title="Send" onPress={handleSend} /> </View> </View> ); }; export default ChatScreen;
In the ChatScreen component, we use useState to manage the messages and text input. We also use useEffect to subscribe to real-time updates when the component mounts. When a message is sent, we call the sendMessage function to add it to Firestore.
Conclusion
Real-time data syncing is a crucial feature for creating engaging and dynamic mobile apps. By integrating Firebase Firestore into your React Native app, you can easily achieve real-time data synchronization, as demonstrated in our chat application example.
This is just the beginning of what you can do with Firebase Firestore and React Native. You can extend this functionality to build collaborative apps, real-time games, or any application where instant data updates are essential.
Remember to handle security rules in your Firestore database to protect your data and ensure that only authorized users can read and write data. Firebase provides a robust authentication system that you can integrate into your app as well.
Incorporate real-time data syncing into your React Native app, and watch your user engagement and satisfaction levels soar.
Happy coding!
Table of Contents
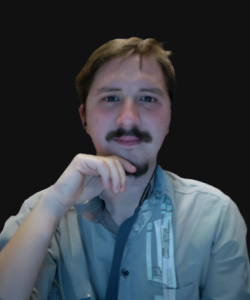
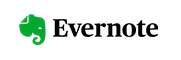