Understanding React Native Layouts: Flexbox and Stylesheets
In the realm of mobile app development, creating appealing and responsive layouts is a fundamental aspect. React Native, a popular framework for building native-like mobile applications using JavaScript, offers powerful tools for designing layouts. Two essential tools in this regard are Flexbox and Stylesheets. In this article, we will delve into these concepts, exploring how they work together to create stunning and adaptable user interfaces.
Table of Contents
1. The Power of Responsive Design
In the era of countless device sizes and screen orientations, responsive design has become an imperative. Users expect applications to adapt seamlessly to their devices, whether it’s a smartphone, tablet, or something in between. This is where React Native shines, providing developers with tools to create layouts that automatically adjust to different screen dimensions.
2. Flexbox: A Layout Miracle
Flexbox, short for Flexible Box Layout, is a layout model that enables the distribution of space and alignment of items within a container. It’s an integral part of CSS, and React Native has embraced it to simplify layout creation. With Flexbox, you define the relationship between items inside a container, allowing them to grow, shrink, and align based on available space.
2.1. Flexbox Terminology
Before diving into the code, let’s cover some important Flexbox terminology:
- Flex Container: The parent element containing flex items.
- Flex Items: The child elements within the flex container.
- Main Axis: The primary axis along which flex items are laid out.
- Cross Axis: The perpendicular axis to the main axis.
- Main Start/End: The beginning and end of the main axis.
- Cross Start/End: The beginning and end of the cross axis.
3. Flexbox in React Native
Implementing Flexbox in React Native is straightforward. Let’s take a look at a basic example:
jsx import React from 'react'; import { View, StyleSheet } from 'react-native'; const FlexboxExample = () => { return ( <View style={styles.container}> <View style={styles.item} /> <View style={styles.item} /> <View style={styles.item} /> </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, flexDirection: 'row', justifyContent: 'space-between', alignItems: 'center', }, item: { width: 50, height: 50, backgroundColor: 'blue', }, }); export default FlexboxExample;
In this example, we create a flex container (container) with three flex items (item). The container uses the Flexbox properties flexDirection, justifyContent, and alignItems to control the layout. The items will be arranged in a row, have equal space between them, and be vertically centered within the container.
4. Exploring Stylesheets
While Flexbox manages the layout of components, Stylesheets handle the visual appearance. Stylesheets in React Native resemble regular CSS, but with a JavaScript twist. They allow you to define styles for your components in a structured and reusable manner.
4.1. Creating and Using Stylesheets
Here’s how you can create and use Stylesheets in React Native:
jsx import React from 'react'; import { View, Text, StyleSheet } from 'react-native'; const StylesheetExample = () => { return ( <View style={styles.container}> <Text style={styles.title}>Welcome to React Native Stylesheets</Text> <View style={styles.box} /> </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', }, title: { fontSize: 24, fontWeight: 'bold', marginBottom: 20, }, box: { width: 150, height: 150, backgroundColor: 'orange', }, }); export default StylesheetExample;
In this example, we define a Stylesheet named styles using the StyleSheet.create method. This ensures better performance by precomputing styles during app initialization. The defined styles can then be applied to corresponding components using the style prop.
5. Integrating Flexbox and Stylesheets
The real magic happens when Flexbox and Stylesheets are combined. You can create dynamic and visually pleasing layouts by applying Flexbox properties within your Stylesheet definitions. Let’s see an example:
jsx import React from 'react'; import { View, StyleSheet } from 'react-native'; const FlexboxStylesExample = () => { return ( <View style={styles.container}> <View style={styles.box1} /> <View style={styles.box2} /> <View style={styles.box3} /> </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, flexDirection: 'row', justifyContent: 'space-between', alignItems: 'center', padding: 20, }, box1: { width: 80, height: 80, backgroundColor: 'red', }, box2: { width: 120, height: 120, backgroundColor: 'green', }, box3: { width: 100, height: 100, backgroundColor: 'blue', }, }); export default FlexboxStylesExample;
In this example, we create a container with three boxes. The container uses Flexbox properties to arrange the boxes in a row with space between them. Each box has its own size and background color defined in the Stylesheet. This integration enables you to achieve both structured layout and appealing visual design effortlessly.
Conclusion
In the realm of React Native development, understanding layouts is crucial for building captivating and user-friendly applications. Flexbox and Stylesheets are essential tools that empower developers to create responsive designs while maintaining a clean and organized codebase. By grasping the concepts of Flexbox and Stylesheets and mastering their integration, you’ll be well-equipped to craft stunning and adaptable user interfaces for your mobile apps. So go ahead, experiment with different layouts, unleash your creativity, and deliver exceptional user experiences through your React Native applications.
Table of Contents
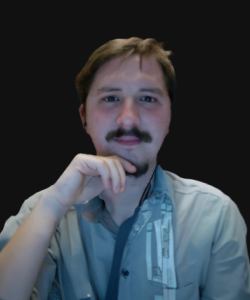
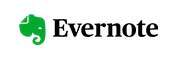