React Native Geolocation: Integrating Maps and Location Services
In the world of mobile app development, providing location-based services has become almost a necessity. From ride-sharing apps to fitness trackers, the ability to leverage geolocation data enhances user experiences and opens doors to a wide range of functionalities. React Native, a popular framework for building cross-platform mobile apps, offers a powerful Geolocation module that enables developers to effortlessly integrate maps and location services. In this tutorial, we’ll dive into the world of React Native Geolocation and explore how to harness its capabilities to create location-aware applications.
Table of Contents
1. Understanding the Basics of Geolocation
1.1. What is Geolocation?
Geolocation is the process of determining a device’s physical location using a combination of techniques, such as GPS, Wi-Fi positioning, and cellular tower triangulation. It’s an essential feature for modern mobile applications that rely on delivering personalized content and services based on a user’s geographic location.
1.2. How Does Geolocation Work in React Native?
React Native provides a Geolocation module that acts as a bridge between JavaScript code and native device functionality. This module exposes a set of methods and events that allow developers to request and receive location data from the device.
2. Setting Up a New React Native Project
2.1. Prerequisites
Before diving into the development process, make sure you have Node.js and npm (Node Package Manager) installed on your system. You’ll also need to have the React Native CLI set up. If not, you can install it using the following command:
bash npm install -g react-native-cli
2.2. Project Initialization
Let’s create a new React Native project called “LocationApp” using the React Native CLI:
bash react-native init LocationApp
Navigate to the project directory:
bash cd LocationApp
3. Integrating Maps with React Native
3.1. Choosing a Map Library
There are several map libraries available for React Native, each with its own set of features and customization options. One of the most popular choices is the react-native-maps library, which provides a comprehensive set of components for integrating maps into your app.
3.2. Installing and Linking the Map Library
To install react-native-maps, use the following command:
bash npm install react-native-maps
The library requires native module linking. After installing, link the library to your project using:
bash react-native link react-native-maps
3.3. Displaying a Map
Now that the library is set up, you can start integrating a map into your app’s components. Import the necessary components from react-native-maps and create a basic map component:
javascript import React from 'react'; import { View } from 'react-native'; import MapView from 'react-native-maps'; const MapScreen = () => { return ( <View style={{ flex: 1 }}> <MapView style={{ flex: 1 }} initialRegion={{ latitude: 37.78825, longitude: -122.4324, latitudeDelta: 0.0922, longitudeDelta: 0.0421, }} /> </View> ); }; export default MapScreen;
3.4. Customizing the Map
The MapView component allows you to customize the map’s appearance and behavior. You can add markers, overlays, and controls to enhance the user experience. Refer to the react-native-maps documentation for detailed information on customization options.
4. Accessing Device Location
4.1. Requesting User Permission
Before accessing the user’s location, you need to request permission to use location services. This is crucial for respecting user privacy and security. You can use the PermissionsAndroid module to handle permission requests on Android.
javascript import { PermissionsAndroid } from 'react-native'; async function requestLocationPermission() { try { const granted = await PermissionsAndroid.request( PermissionsAndroid.PERMISSIONS.ACCESS_FINE_LOCATION, { title: 'Location Permission', message: 'Location App needs access to your location.', buttonNeutral: 'Ask Me Later', buttonNegative: 'Cancel', buttonPositive: 'OK', }, ); if (granted === PermissionsAndroid.RESULTS.GRANTED) { console.log('Location permission granted.'); } else { console.log('Location permission denied.'); } } catch (err) { console.warn(err); } }
4.2. Retrieving Current Location
Once you have permission, you can use the Geolocation module to retrieve the device’s current location.
javascript import Geolocation from '@react-native-community/geolocation'; Geolocation.getCurrentPosition( position => { const { latitude, longitude } = position.coords; console.log(`Latitude: ${latitude}, Longitude: ${longitude}`); }, error => { console.warn(`Error getting location: ${error.message}`); }, { enableHighAccuracy: true, timeout: 15000, maximumAge: 10000 } );
4.3. Handling Location Changes
You can also subscribe to location updates using the watchPosition method. This allows you to continuously track the device’s movement.
javascript const watchId = Geolocation.watchPosition( position => { const { latitude, longitude } = position.coords; console.log(`Latitude: ${latitude}, Longitude: ${longitude}`); }, error => { console.warn(`Error getting location: ${error.message}`); }, { enableHighAccuracy: true, distanceFilter: 100 } ); // To stop watching for location updates: Geolocation.clearWatch(watchId);
5. Implementing Location-Based Features
5.1. Displaying User Location on the Map
With the retrieved location data, you can display the user’s current location on the map using a marker:
javascript <MapView> <MapView.Marker coordinate={{ latitude, longitude }} title="You are here" description="Your current location" /> </MapView>
5.2. Geofencing
Geofencing involves defining virtual boundaries and receiving notifications when a device enters or exits these boundaries. You can implement geofencing using a combination of location data and triggers.
5.3. Creating Location-Aware Components
To create components that respond to the user’s location, you can use the location data to customize the behavior or content. For example, you could show nearby points of interest, adjust the app’s theme based on the time of day, or provide location-specific recommendations.
Conclusion
React Native Geolocation provides a robust foundation for integrating maps and location services into your mobile applications. With the ability to access user location, customize map components, and create location-aware features, you can enhance user experiences and unlock a world of possibilities. Whether you’re building a navigation app, a social platform, or an on-demand service, harnessing the power of geolocation will undoubtedly elevate your app to new heights. So, start exploring the world of React Native Geolocation and bring your location-based app ideas to life!
Table of Contents
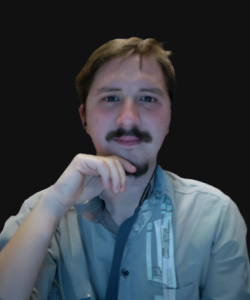
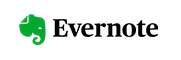