React Native and GraphQL: Integrating APIs for Data Fetching
In the world of mobile app development, React Native has emerged as a powerful framework for building cross-platform applications with a single codebase. Coupled with the flexibility of GraphQL, developers can create efficient data fetching solutions that cater to the specific needs of their applications. This blog post dives into the integration of GraphQL APIs into React Native projects, providing insights, examples, and a step-by-step guide to help developers leverage these technologies effectively.
1. Understanding GraphQL and Its Advantages
1.1 What is GraphQL?
GraphQL is a query language for APIs that enables clients to request precisely the data they need and nothing more. Unlike traditional REST APIs where clients often receive more data than required, GraphQL allows developers to design flexible and efficient queries, reducing over-fetching and under-fetching of data. With GraphQL, the structure of the response is dictated by the query itself, putting control in the hands of the client.
1.2 Advantages of GraphQL for Mobile Apps
For mobile app development, GraphQL offers several advantages:
- Efficient Data Fetching: Mobile devices often have limited resources and connectivity. GraphQL’s ability to fetch only the required data helps in optimizing app performance and conserving resources.
- Reduced Number of Requests: GraphQL allows you to retrieve multiple types of data in a single query, reducing the number of requests made to the server. This is particularly beneficial for improving network efficiency.
- Customizable Responses: Developers can define the shape and structure of the response according to the app’s requirements, leading to a more streamlined development process and better client-server communication.
- Real-time Updates: GraphQL supports real-time data with subscriptions, enabling apps to receive updates when data changes on the server, which is crucial for interactive and dynamic applications.
2. Setting Up a React Native Project
To get started with integrating GraphQL into your React Native application, you first need to set up a new project and install the necessary dependencies.
2.1 Creating a New React Native Project
You can create a new React Native project using the following command:
bash npx react-native init YourProjectName
2.2 Installing Dependencies
For GraphQL integration, you’ll need the Apollo Client library. Apollo Client simplifies the process of fetching data from a GraphQL API and managing local application state.
Install Apollo Client and its dependencies by running:
bash npm install @apollo/client graphql
3. Introducing Apollo Client
3.1 Role of Apollo Client in React Native
Apollo Client is a comprehensive state management library for JavaScript applications, including React Native. It works seamlessly with GraphQL APIs, offering features such as data caching, automatic refetching, and support for real-time data through subscriptions.
In your React Native app, Apollo Client acts as the bridge between your components and the GraphQL server. It enables you to define queries, mutations, and subscriptions, and then execute them within your app.
4. Defining GraphQL Schema and Queries
Before fetching data, you need to understand your data requirements and design your GraphQL schema and queries accordingly.
4.1 Designing Your Data Requirements
Start by defining the types of data your app needs. This could include user information, posts, comments, and more. Create a schema using GraphQL’s type system, outlining the structure of your data.
4.2 Constructing GraphQL Queries
With your schema in place, construct queries using the GraphQL query language. Queries specify the data you want to fetch and their structure. For instance, to fetch a user’s name and their latest posts, your query might look like:
graphql query { user(id: "user123") { name posts(limit: 5, sortBy: "latest") { title content } } }
5. Fetching Data with React Native and GraphQL
Now that you have your queries defined, it’s time to integrate them into your React Native components using Apollo Client.
5.1 Initializing Apollo Client
In your app’s entry file (e.g., App.js), set up Apollo Client by creating an instance of ApolloClient and wrapping your app with the ApolloProvider component:
jsx import { ApolloClient, InMemoryCache, ApolloProvider } from '@apollo/client'; const client = new ApolloClient({ uri: 'https://your-graphql-api-url.com', cache: new InMemoryCache(), }); const App = () => { return ( <ApolloProvider client={client}> {/* Your app components */} </ApolloProvider> ); }; export default App;
5.2 Executing Queries within Components
Within your components, you can use the useQuery hook from Apollo Client to execute your GraphQL queries:
jsx import { useQuery } from '@apollo/client'; import { GET_USER_DATA } from './queries'; // Import your query const UserProfile = () => { const { loading, error, data } = useQuery(GET_USER_DATA); if (loading) return <p>Loading...</p>; if (error) return <p>Error: {error.message}</p>; const { user } = data; return ( <div> <h1>{user.name}</h1> <ul> {user.posts.map(post => ( <li key={post.id}> <h3>{post.title}</h3> <p>{post.content}</p> </li> ))} </ul> </div> ); };
6. Handling Mutations for Data Manipulation
Apart from fetching data, your app might need to perform mutations to modify data on the server, such as creating a new post or updating a user’s profile.
6.1 Incorporating Mutations in Your App
Define your mutations similarly to how you defined queries, specifying the input parameters and return types. Then, use the useMutation hook to execute mutations in your components:
jsx import { useMutation } from '@apollo/client'; import { CREATE_POST } from './mutations'; // Import your mutation const NewPostForm = () => { const [createPost] = useMutation(CREATE_POST); const handleCreatePost = async () => { try { await createPost({ variables: { title: 'New Post', content: 'This is a new post.' }, }); // Handle success or update UI } catch (error) { // Handle error } }; return ( <div> <button onClick={handleCreatePost}>Create Post</button> </div> ); };
6.2 Updating the UI after Mutations
After a mutation, you might want to update your UI to reflect the changes. Apollo Client provides mechanisms for automatic cache updates or manual cache modifications to ensure your UI remains consistent with the server data.
7. Optimizing Data Fetching
GraphQL and Apollo Client offer optimization techniques to enhance data fetching performance.
7.1 Utilizing Caching for Improved Performance
Apollo Client’s caching mechanism stores fetched data locally, reducing the need to make repetitive network requests. Cached data can be reused across components, enhancing the app’s responsiveness.
7.2 Implementing Pagination in Queries
For scenarios where you’re fetching large amounts of data, implementing pagination is crucial to avoid overloading the app. GraphQL supports pagination through arguments like limit and offset. You can control the number of items fetched in a single query and request more as needed.
8. Error Handling and Feedback
While working with GraphQL, it’s essential to handle potential errors gracefully and provide feedback to users.
8.1 Dealing with GraphQL Errors
Apollo Client returns error objects in the error field of query and mutation results. Handle errors by displaying appropriate messages to users and guiding them on corrective actions.
8.2 Providing User Feedback
Keep users informed about ongoing network requests, loading states, and success/error outcomes. This enhances user experience and reduces confusion.
9. Real-time Data with Subscriptions
One of GraphQL’s standout features is its support for real-time data updates through subscriptions.
9.1 Setting Up Subscriptions in React Native
To enable subscriptions, your GraphQL server needs to support them. Define subscription types in your schema and implement corresponding resolvers on the server. In your React Native app, use the useSubscription hook to subscribe to events and update the UI in real time.
9.2 Updating the UI with Real-time Data
For use cases like chat applications, live feeds, or collaborative editing, subscriptions ensure that your app stays updated without requiring constant manual refreshing.
10. Testing and Debugging
As with any development process, thorough testing and debugging are crucial for maintaining app stability and performance.
10.1 Tools for Testing GraphQL Queries
Tools like Apollo Client Devtools provide insights into your GraphQL queries, enabling you to test them, analyze performance, and ensure efficient data fetching.
10.2 Debugging Common Issues
When encountering issues, check for mistakes in query syntax, ensure proper data types, and utilize debugging tools to identify and resolve errors effectively.
11. Deployment and Beyond
Once your React Native app with GraphQL integration is ready, it’s time to prepare for deployment.
11.1 Building and Deploying Your App
Build your app for production using tools like Metro Bundler and integrate deployment pipelines. Ensure that your GraphQL server is properly configured for production use.
11.2 Monitoring and Scaling GraphQL APIs
As your app gains users, monitor the performance of your GraphQL APIs and scale resources as needed. Implement caching strategies, query optimization, and distributed systems best practices to maintain a seamless experience for users.
Conclusion
Integrating GraphQL APIs into your React Native application opens up a world of possibilities for efficient and flexible data fetching. With Apollo Client as your ally, you can design queries and mutations tailored to your app’s needs, optimize data fetching performance, handle real-time updates, and ensure a smooth user experience. By following the steps outlined in this guide, you’re well on your way to harnessing the power of React Native and GraphQL for your mobile app development endeavors. Happy coding!
Table of Contents
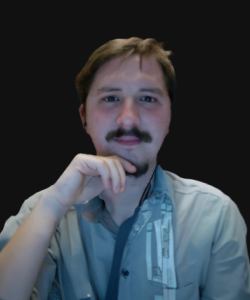
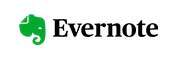