React Native Image Handling: Uploading, Cropping, and Optimizing Images
In the world of mobile app development, images play a pivotal role in creating visually appealing and engaging user experiences. However, managing images efficiently can be a challenging task, especially when dealing with constraints like file size, aspect ratios, and different device resolutions. In this tutorial, we’ll delve into the techniques of handling images in a React Native app. We’ll cover everything from uploading and cropping to optimizing images, equipping you with the tools to enhance performance and user satisfaction.
Table of Contents
1. Understanding the Importance of Image Handling
1.1. The Role of Images in Mobile Apps
Images serve as the visual storytellers of your app, conveying messages, emotions, and functionalities that text alone might struggle to achieve. From profile pictures to product images, images foster user engagement and make your app more memorable.
1.2. Challenges in Image Management
However, images can pose challenges, such as increasing the app’s size, causing slower load times, and even leading to crashes if not handled properly. This is where effective image handling becomes crucial, ensuring that images are optimized for different devices and screen sizes without compromising on quality.
2. Uploading Images in React Native
2.1. Utilizing Libraries for Image Upload
React Native offers a range of libraries that simplify image uploading. Some popular options include react-native-image-picker and react-native-document-picker, which provide a seamless interface for users to select and upload images from their devices.
2.2. Step-By-Step Uploading Process
- Integrate the chosen library into your React Native project using npm or yarn.
- Import the necessary modules from the library and set up the UI components to trigger the image selection process.
- Implement the logic to handle the selected image, convert it to a suitable format if necessary, and initiate the upload to your server or cloud storage.
Code Sample: Uploading an Image
javascript import React, { useState } from 'react'; import { View, Button, Image } from 'react-native'; import ImagePicker from 'react-native-image-picker'; const ImageUploadScreen = () => { const [selectedImage, setSelectedImage] = useState(null); const handleImageUpload = () => { ImagePicker.showImagePicker({}, response => { if (response.didCancel) { console.log('Image upload cancelled'); } else if (response.error) { console.error('Image upload error:', response.error); } else { setSelectedImage(response.uri); // Upload logic here } }); }; return ( <View> {selectedImage && <Image source={{ uri: selectedImage }} style={{ width: 200, height: 200 }} />} <Button title="Select Image" onPress={handleImageUpload} /> </View> ); }; export default ImageUploadScreen;
3. Cropping Images for Consistency
3.1. The Significance of Image Cropping
Maintaining consistent aspect ratios and visual coherence across your app’s images is essential. Cropping allows you to highlight specific parts of an image and adapt it to various layouts.
3.2. Implementing Image Cropping Functionality
To enable image cropping, you can employ libraries like react-native-image-crop-picker. This library not only facilitates image cropping but also offers features like rotation and custom dimensions.
Code Sample: Adding Image Cropping
javascript import React, { useState } from 'react'; import { View, Button, Image } from 'react-native'; import ImageCropPicker from 'react-native-image-crop-picker'; const ImageCropScreen = () => { const [croppedImage, setCroppedImage] = useState(null); const handleImageCrop = () => { ImageCropPicker.openCropper({ path: selectedImage, width: 300, height: 300, cropping: true, }).then(image => { setCroppedImage(image.path); }); }; return ( <View> {croppedImage && <Image source={{ uri: croppedImage }} style={{ width: 200, height: 200 }} />} <Button title="Crop Image" onPress={handleImageCrop} /> </View> ); }; export default ImageCropScreen;
4. Optimizing Images for Performance
4.1. Why Image Optimization Matters
Unoptimized images can lead to slow app performance and increased data usage. Image optimization involves reducing file size without sacrificing quality, ensuring that images load quickly and users have a smooth experience.
4.2. Techniques for Image Compression
There are several strategies to compress images, such as resizing, choosing appropriate formats (JPEG, PNG, WebP), and using compression libraries like react-native-image-resizer.
4.3. Integrating Optimized Images
After compressing images, replace the original images in your app with the optimized versions. This can significantly enhance app performance and reduce loading times.
Code Sample: Image Optimization in React Native
javascript import React from 'react'; import { View, Image } from 'react-native'; const OptimizedImageScreen = () => { return ( <View> <Image source={require('./images/optimized_image.jpg')} style={{ width: 300, height: 200 }} /> </View> ); }; export default OptimizedImageScreen;
Conclusion
Efficient image handling is a critical aspect of creating successful React Native applications. By mastering image uploading, cropping, and optimization techniques, you can strike the right balance between visual appeal and app performance. Remember to choose the right libraries and tools that align with your project’s requirements, and continuously test your app on various devices to ensure consistent image quality and responsiveness. Your ability to handle images effectively will contribute to a seamless and delightful user experience, setting your app apart in today’s competitive mobile landscape.
Table of Contents
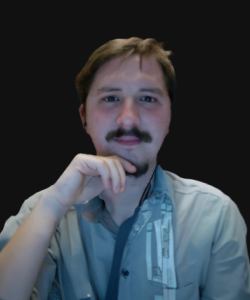
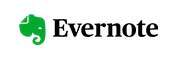