React Native and OneSignal: Implementing Push Notifications
Push notifications have become an integral part of the mobile app user experience. They enable real-time communication with users, helping you engage, retain, and re-engage your audience effectively. If you’re developing a mobile app with React Native, integrating push notifications is crucial to keep your users informed and engaged.
Table of Contents
In this guide, we’ll walk you through the process of implementing push notifications in your React Native app using OneSignal, a popular push notification service. With OneSignal, you can easily send notifications to your users on both iOS and Android devices. Let’s get started!
1. Prerequisites
Before we dive into the implementation, ensure you have the following prerequisites in place:
1.1. React Native Development Environment
You should have a React Native development environment set up on your machine. If you haven’t already, follow the official React Native documentation to set up your environment: React Native Getting Started.
1.2. OneSignal Account
Sign up for a OneSignal account if you don’t have one already. OneSignal provides a free tier that is suitable for most small to medium-sized apps. You can create an account here: OneSignal Signup.
1.3. React Native Project
Have a React Native project ready or create a new one using the following command:
bash npx react-native init MyPushNotificationApp
Replace “MyPushNotificationApp” with your desired project name.
2. Setting Up OneSignal
Now that you have your development environment and OneSignal account ready, let’s integrate OneSignal into your React Native project.
2.1. Install the OneSignal React Native SDK
To integrate OneSignal into your React Native app, you’ll need to install the OneSignal React Native SDK. Open your project directory in the terminal and run the following command:
bash npm install react-native-onesignal
or if you prefer using Yarn:
bash yarn add react-native-onesignal
2.2. Link the Native Modules
In React Native, you often need to link native modules to your project. Fortunately, the OneSignal SDK makes this process relatively straightforward. Run the following command to link the native modules:
bash npx react-native link react-native-onesignal
2.3. Configure the OneSignal SDK
Before you can start sending push notifications, you need to configure the OneSignal SDK with your OneSignal App ID. Locate your MainApplication.java file (found in android/app/src/main/java/com/yourappname) and open it.
Add the following import statement at the top of the file:
java import com.onesignal.OneSignal;
Then, inside the onCreate method, add the following code to initialize the OneSignal SDK with your OneSignal App ID:
java OneSignal.initWithContext(this); OneSignal.setAppId("YOUR_ONESIGNAL_APP_ID");
Replace “YOUR_ONESIGNAL_APP_ID” with your actual OneSignal App ID.
2.4. iOS Configuration (if applicable)
For iOS, you’ll also need to make some additional configurations. Open your AppDelegate.m file (found in ios/yourappname) and add the following import statement at the top of the file:
objc #import <OneSignal/OneSignal.h>
Then, inside the didFinishLaunchingWithOptions method, add the following code to initialize OneSignal:
objc [OneSignal initWithLaunchOptions:launchOptions appId:@"YOUR_ONESIGNAL_APP_ID"];
Make sure to replace “YOUR_ONESIGNAL_APP_ID” with your actual OneSignal App ID.
2.5. Initialize OneSignal in Your React Native Code
Now that you’ve configured OneSignal in your native code, it’s time to initialize it in your React Native code. Open your index.js or App.js file and add the following import statement at the top:
javascript import OneSignal from 'react-native-onesignal';
Next, add the following code to initialize OneSignal in your app:
javascript OneSignal.setAppId("YOUR_ONESIGNAL_APP_ID");
Again, replace “YOUR_ONESIGNAL_APP_ID” with your actual OneSignal App ID.
3. Implementing Push Notifications
With OneSignal integrated into your React Native app, you can now start implementing push notifications. We’ll cover the basic steps to send a push notification to your users.
3.1. Request Permission
Before sending push notifications, you need to request permission from the user. This step is essential for both iOS and Android. You can use the following code to request permission:
javascript OneSignal.promptForPushNotificationsWithUserResponse(response => { console.log("Push notification permission:", response); });
This code will display a permission prompt to the user. Once the user grants permission, you can proceed to send notifications.
3.2. Sending a Push Notification
To send a push notification from your server to the devices running your app, you can use the OneSignal REST API or the OneSignal Dashboard. Here, we’ll focus on using the OneSignal Dashboard for simplicity.
- Log in to your OneSignal account and select your app.
- Go to the “Messages” tab in the left sidebar.
- Click the “New Push” button to create a new push notification.
- Configure your notification by specifying the title, message, and target audience.
- Schedule or send the notification immediately as per your requirements.
OneSignal provides a user-friendly interface to compose and send notifications to your users.
3.3. Handling Incoming Notifications
When your app receives a push notification, you can handle it in the JavaScript code to perform specific actions when the user taps on the notification. To do this, you can use the OneSignal.setNotificationOpenedHandler function. Here’s an example:
javascript OneSignal.setNotificationOpenedHandler(notification => { // Handle the notification here console.log("Notification opened:", notification); });
This code allows you to capture and process incoming notifications.
4. Testing Push Notifications
Testing is a crucial part of implementing push notifications. Here are some tips for testing push notifications in your React Native app:
4.1. Testing on Real Devices
Always test push notifications on real devices to ensure they work as expected on both iOS and Android. Use development builds of your app for testing.
4.2. Debugging
To troubleshoot issues with push notifications, check the console logs for any error messages or unexpected behavior. OneSignal and React Native provide useful debugging information.
4.3. Testing Different Scenarios
Test various scenarios, such as receiving notifications while the app is in the background, foreground, or terminated. Ensure that the notifications are displayed correctly and that tapping them triggers the desired actions.
4.4. iOS Device Tokens
Keep in mind that iOS uses device tokens to send notifications. These tokens can change, so handle token updates gracefully in your app.
Conclusion
Implementing push notifications in your React Native app using OneSignal is a powerful way to engage your users, deliver real-time updates, and enhance the overall user experience. With the integration and setup steps covered in this guide, you’re well on your way to effectively using push notifications to keep your users informed and engaged. Happy coding!
Table of Contents
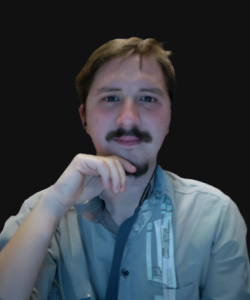
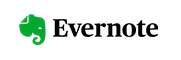