React Native and In-App Purchases: Monetizing Your App
In today’s mobile app ecosystem, monetization is a crucial aspect of a successful project. React Native, a popular framework for building cross-platform mobile apps, offers an excellent platform for creating apps with revenue potential. One effective monetization strategy that can significantly boost your app’s income is integrating in-app purchases.
Table of Contents
This blog post will guide you through the process of monetizing your React Native app with in-app purchases. We will cover everything from understanding the concept of in-app purchases to implementing them in your app, along with best practices and code samples to help you get started.
1. Understanding In-App Purchases
Before diving into implementation details, it’s essential to understand what in-app purchases are and how they work. In-app purchases, often abbreviated as IAPs, allow users to buy digital content or features within your app. This could include premium features, ad removal, virtual goods, or subscriptions.
1.1. Types of In-App Purchases
In-app purchases typically fall into three main categories:
- Consumable: These are one-time purchases of digital items that users consume and may need to repurchase, such as in-game currency or power-ups.
- Non-consumable: Non-consumable purchases are one-time purchases of items that users don’t consume and have permanent access to, like unlocking premium features or removing ads.
- Subscription: Subscriptions grant users access to premium content or features for a set period, often on a monthly or yearly basis. This is a recurring revenue model.
2. Preparing Your React Native Project
To start monetizing your React Native app with in-app purchases, you’ll need to prepare your project. Here are the steps to get started:
2.1. Set Up a Developer Account
First, you’ll need to create a developer account with the respective app store platforms you plan to release your app on, such as the Apple App Store and Google Play Store.
2.2. Add Necessary Dependencies
To handle in-app purchases in React Native, you’ll need to use third-party libraries like react-native-iap. Install it in your project using npm or yarn:
bash npm install react-native-iap --save # or yarn add react-native-iap
2.3. Configure the Libraries
Follow the installation and configuration instructions provided by react-native-iap to integrate it into your project. Ensure that you set up the necessary permissions and app-specific configurations on both iOS and Android.
3. Implementing In-App Purchases
Now that your project is set up, let’s dive into implementing in-app purchases.
3.1. Initialize the Billing Library
To get started with in-app purchases in your React Native app, initialize the billing library with the appropriate configuration. Here’s an example:
javascript import { RNIap } from 'react-native-iap'; async function initializeIAP() { try { await RNIap.initConnection(); console.log('IAP initialized successfully'); } catch (error) { console.error('Failed to initialize IAP', error); } } initializeIAP();
3.2. Fetch Available Products
To display available products for purchase, you can use the getProducts method. This method queries the app store for product details based on the product IDs you provide.
javascript const productIds = ['com.yourapp.premium_feature']; async function fetchProducts() { try { const products = await RNIap.getProducts(productIds); console.log('Available products:', products); } catch (error) { console.error('Failed to fetch products', error); } } fetchProducts();
3.2. Display Products to Users
Now that you have fetched the available products, you can display them to your users within your app’s user interface. This may involve creating a dedicated screen or integrating purchase options into existing features.
javascript function ProductList({ products, onPurchase }) { return ( <ul> {products.map((product) => ( <li key={product.productId}> {product.title} - ${product.price} <button onClick={() => onPurchase(product)}>Buy</button> </li> ))} </ul> ); }
3.3. Handle User Purchases
When a user decides to make a purchase, you need to handle the purchase flow and verify the transaction. Here’s an example of how to do this:
javascript async function purchaseProduct(product) { try { const purchase = await RNIap.buyProduct(product.productId); console.log('Purchase successful:', purchase); // Add logic to grant the user access to the purchased item or feature } catch (error) { console.error('Purchase failed', error); } }
3.4. Implement Consumable Purchases
If you’re offering consumable items, like in-game currency, you’ll need to handle the consumption of these items when the user uses them within your app. Here’s how you can do that:
javascript async function consumeProduct(purchaseToken) { try { await RNIap.consumePurchaseAndroid(purchaseToken); console.log('Item consumed successfully'); } catch (error) { console.error('Failed to consume item', error); } }
3.5. Implement Subscriptions
For subscription-based purchases, you’ll need to manage the subscription status and provide access to premium content or features while the subscription is active. Here’s a simplified example:
javascript async function checkSubscriptionStatus() { try { const subscribed = await RNIap.checkSubscription('com.yourapp.subscription'); if (subscribed) { // Grant access to premium content } else { // Display options to subscribe } } catch (error) { console.error('Failed to check subscription status', error); } }
3.6. Test Your Implementation
Before releasing your app to the app stores, it’s essential to thoroughly test your in-app purchase implementation. Both Apple and Google provide sandbox environments for testing purchases without actual money.
4. Best Practices for In-App Purchases
Successful monetization through in-app purchases requires a thoughtful approach. Here are some best practices to consider:
4.1. Provide Value
Ensure that your in-app purchases genuinely enhance the user experience. Users are more likely to make purchases when they see value in what you offer.
4.2. Offer Free Trials
For subscription-based apps, offering free trials can encourage users to subscribe. Let them experience the premium features before committing to a subscription.
4.3. Regularly Update Content
Keep your app fresh by regularly adding new content or features available through in-app purchases. This can help retain existing users and attract new ones.
4.4. Optimize Pricing
Experiment with pricing to find the sweet spot that maximizes your revenue while keeping users engaged. A/B testing can help you determine the most effective pricing strategy.
4.5. Use Analytics
Leverage analytics tools to track user behavior, purchase patterns, and conversion rates. Data-driven insights can guide your monetization strategy.
4.6. Be Transparent
Clearly communicate to users what they get with each purchase or subscription. Transparency builds trust and reduces the likelihood of refund requests.
4.7. Handle Refunds Gracefully
Be prepared to handle refund requests professionally. Providing excellent customer support can turn refund requests into loyal users.
Conclusion
Monetizing your React Native app with in-app purchases is a powerful way to generate revenue and sustain your app’s growth. By understanding the different types of in-app purchases, implementing them correctly, and following best practices, you can create a profitable app while providing value to your users.
Remember that successful monetization is an ongoing process. Continuously analyze user feedback and data to refine your in-app purchase strategy and keep your app competitive in the ever-evolving mobile app market.
Start implementing in-app purchases in your React Native app today, and watch your revenue soar as your users enjoy the premium content and features you offer. Happy coding and monetizing!
Table of Contents
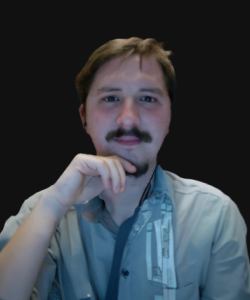
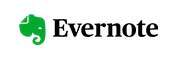