React Native and GraphQL Apollo: Integrating GraphQL in Apps
In today’s fast-paced world of app development, delivering a seamless and responsive user experience is crucial. To achieve this, developers often turn to technologies like React Native and GraphQL. In this blog post, we’ll explore how to integrate GraphQL into React Native apps using Apollo Client. By the end, you’ll have a solid understanding of the process, and you’ll be able to harness the power of GraphQL to supercharge your mobile applications.
Table of Contents
1. Why Use GraphQL with React Native?
Before diving into the integration process, let’s briefly discuss why GraphQL is a great fit for React Native development.
1.1. Efficient Data Fetching
GraphQL allows you to request only the data you need, reducing the over-fetching and under-fetching of data. This results in more efficient data fetching, especially important in mobile apps where bandwidth and performance are critical.
1.2. Strongly Typed Schema
With GraphQL, you define a schema that specifies the structure of your data. This schema serves as a contract between the client and the server, ensuring that data is retrieved in a predictable and type-safe manner, reducing runtime errors.
1.3. Real-time Data
GraphQL supports real-time data updates through subscriptions. This feature is invaluable for building interactive and dynamic mobile applications.
Now that we’ve established the benefits of using GraphQL let’s move on to integrating it with React Native using Apollo Client.
2. Setting Up a React Native Project
Assuming you already have Node.js and React Native development environment set up, let’s create a new React Native project. If you haven’t installed React Native CLI, you can do so by running:
bash npm install -g react-native-cli
Now, create a new React Native project with the following command:
bash npx react-native init MyApp
Replace MyApp with your preferred project name.
3. Installing Apollo Client
To get started with GraphQL in your React Native project, you’ll need to install Apollo Client, a widely used GraphQL client that integrates seamlessly with React Native. Apollo Client provides tools for making GraphQL queries, mutations, and handling caching efficiently.
Let’s install Apollo Client:
bash npm install @apollo/client graphql
Once Apollo Client is installed, you can set up your GraphQL endpoint. In this example, we’ll assume you have a GraphQL server running at https://example.com/graphql.
4. Setting Up Apollo Client
Next, you need to set up Apollo Client in your React Native application. Apollo Client needs to know the URI of your GraphQL server. You can do this by creating an instance of the ApolloClient and providing the uri as an option.
javascript import { ApolloClient, InMemoryCache } from '@apollo/client'; const client = new ApolloClient({ uri: 'https://example.com/graphql', cache: new InMemoryCache(), });
Make sure to replace ‘https://example.com/graphql’ with the actual URL of your GraphQL server.
5. Creating Your First GraphQL Query
With Apollo Client configured, you can start making GraphQL queries. Let’s create a simple query to fetch a list of books from your GraphQL server.
javascript import { useQuery, gql } from '@apollo/client'; const GET_BOOKS = gql` query GetBooks { books { title author } } `; function BooksList() { const { loading, error, data } = useQuery(GET_BOOKS); if (loading) return <p>Loading...</p>; if (error) return <p>Error: {error.message}</p>; return ( <ul> {data.books.map((book, index) => ( <li key={index}> <strong>{book.title}</strong> by {book.author} </li> ))} </ul> ); }
In this code snippet, we define a GraphQL query using the gql template literal and the useQuery hook from Apollo Client to execute the query. We then display the list of books and handle loading and error states.
6. Executing Mutations
GraphQL not only allows you to fetch data but also to modify it using mutations. Let’s create a mutation to add a new book to your server.
javascript import { useMutation, gql } from '@apollo/client'; const ADD_BOOK = gql` mutation AddBook($title: String!, $author: String!) { addBook(title: $title, author: $author) { title author } } `; function AddBookForm() { const [title, setTitle] = useState(''); const [author, setAuthor] = useState(''); const [addBook] = useMutation(ADD_BOOK); const handleSubmit = () => { addBook({ variables: { title, author } }) .then((response) => { console.log('Book added:', response.data.addBook); // You can update your UI as needed }) .catch((error) => { console.error('Error adding book:', error); }); }; return ( <div> <input type="text" placeholder="Title" value={title} onChange={(e) => setTitle(e.target.value)} /> <input type="text" placeholder="Author" value={author} onChange={(e) => setAuthor(e.target.value)} /> <button onClick={handleSubmit}>Add Book</button> </div> ); }
In this example, we use the useMutation hook from Apollo Client to execute the ADD_BOOK mutation. The mutation takes title and author as variables, which we extract from the component’s state. After successfully adding a book, you can update your UI to reflect the changes.
7. Handling Authentication
Authentication is a critical aspect of most mobile apps. With Apollo Client, you can easily handle authentication and send authenticated requests to your GraphQL server.
Here’s a simplified example of how you can handle authentication with Apollo Client:
javascript import { ApolloClient, InMemoryCache, ApolloLink, HttpLink } from '@apollo/client'; // Create an HTTP link to your GraphQL server const httpLink = new HttpLink({ uri: 'https://example.com/graphql' }); // Create an authentication middleware const authMiddleware = new ApolloLink((operation, forward) => { // Add your authentication logic here const token = localStorage.getItem('token'); // Assuming you store the token in localStorage operation.setContext({ headers: { Authorization: token ? `Bearer ${token}` : '', }, }); return forward(operation); }); const client = new ApolloClient({ link: authMiddleware.concat(httpLink), cache: new InMemoryCache(), });
In this code, we create an authMiddleware that adds an Authorization header to outgoing requests, using a token stored in localStorage. You can customize this logic to fit your authentication requirements.
8. Handling Real-time Data with Subscriptions
One of the significant advantages of GraphQL is its support for real-time data through subscriptions. Apollo Client makes it straightforward to implement subscriptions in your React Native app.
Here’s an example of how to set up a subscription:
javascript import { useSubscription, gql } from '@apollo/client'; const NEW_BOOKS_SUBSCRIPTION = gql` subscription OnNewBook { newBook { title author } } `; function BookSubscription() { const { loading, error, data } = useSubscription(NEW_BOOKS_SUBSCRIPTION); if (loading) return <p>Waiting for new books...</p>; if (error) return <p>Error: {error.message}</p>; const newBook = data.newBook; return ( <div> <p>New Book Alert:</p> <strong>{newBook.title}</strong> by {newBook.author} </div> ); }
In this example, we use the useSubscription hook to subscribe to new books being added in real-time. When a new book is added, the subscription updates the UI accordingly.
9. Testing GraphQL Queries and Mutations
To ensure the reliability and correctness of your GraphQL queries and mutations, it’s essential to write unit tests. Apollo Client provides utilities for testing GraphQL operations.
Here’s an example of how you can test a GraphQL query using the MockedProvider from Apollo Client Testing:
javascript import { MockedProvider } from '@apollo/client/testing'; import { render, screen } from '@testing-library/react'; const GET_BOOKS = gql` query GetBooks { books { title author } } `; const mocks = [ { request: { query: GET_BOOKS, }, result: { data: { books: [ { title: 'Book 1', author: 'Author 1' }, { title: 'Book 2', author: 'Author 2' }, ], }, }, }, ]; it('renders book list correctly', async () => { render( <MockedProvider mocks={mocks} addTypename={false}> <BooksList /> </MockedProvider> ); // You can write your assertions here using testing-library queries const book1 = await screen.findByText('Book 1 by Author 1'); const book2 = await screen.findByText('Book 2 by Author 2'); expect(book1).toBeInTheDocument(); expect(book2).toBeInTheDocument(); });
In this test, we use the MockedProvider to simulate a GraphQL query response and then use testing-library functions to assert that the component correctly renders the data.
Conclusion
Integrating GraphQL into your React Native app using Apollo Client opens up a world of possibilities for efficient data fetching, real-time updates, and seamless user experiences. With GraphQL’s flexibility and Apollo Client’s powerful features, you can build high-performance mobile applications that scale with ease.
In this blog post, we covered the basics of integrating Apollo Client into a React Native project, making queries and mutations, handling authentication, and implementing real-time data with subscriptions. Armed with this knowledge, you’re well-prepared to harness the power of GraphQL in your mobile app development journey. So, go ahead, supercharge your React Native apps with GraphQL, and create exceptional user experiences!
Now, it’s time to dive in, experiment, and build amazing GraphQL-powered React Native apps. Happy coding!
Table of Contents
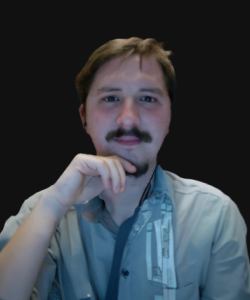
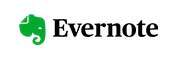