React Native and Apple Pay: Integrating In-App Payments
In the world of mobile app development, creating a smooth and secure payment experience for users is essential. With the advent of technologies like Apple Pay, developers can now offer a seamless in-app payment process that enhances user convenience and trust. In this blog, we’ll explore how to integrate Apple Pay into your React Native application, step by step. We’ll provide code samples and expert guidance to help you get started with in-app payments in no time.
Table of Contents
1. Why Apple Pay?
Before we dive into the technical details, let’s understand why integrating Apple Pay into your React Native app is a smart move.
1.1. Enhanced User Experience
Apple Pay simplifies the payment process by allowing users to make purchases using their stored payment methods, such as credit or debit cards, directly from within your app. This eliminates the need for users to manually enter their payment information, making transactions quicker and more convenient.
1.2. Trust and Security
Apple Pay is known for its robust security features. It uses tokenization to secure users’ payment data, ensuring that sensitive information is never stored on your servers or within the app itself. This level of security builds trust among users, making them more likely to complete transactions.
1.3. Global Reach
Apple Pay is available in numerous countries and regions, making it a suitable choice for apps with a global audience. By integrating Apple Pay, you can cater to a broader user base and increase your app’s accessibility.
Now that we understand the benefits, let’s proceed with the integration process.
2. Prerequisites
Before you start integrating Apple Pay into your React Native app, make sure you have the following prerequisites in place:
2.1. A React Native Project
Ensure you have an existing React Native project or create a new one if you haven’t already. You can set up a new project using the React Native CLI.
2.2. Apple Developer Account
You’ll need an Apple Developer account to configure and enable Apple Pay for your app. If you don’t have one, you can sign up on the Apple Developer website.
2.3. Xcode and a Physical iOS Device
To test Apple Pay integration, you’ll need Xcode installed on a Mac and a physical iOS device. Emulators do not support Apple Pay testing.
2.4. A Payment Processor Account
Choose a payment processor, such as Stripe or Braintree, and create an account. These processors will help you handle payments securely and easily integrate with Apple Pay.
With these prerequisites in place, let’s move on to the integration process.
Step 1: Configure Apple Pay in Your Apple Developer Account
To begin, you’ll need to configure Apple Pay in your Apple Developer account. Follow these steps:
- Log in to your Apple Developer account if you haven’t already.
- Navigate to the Certificates, Identifiers & Profiles section.
- Select App IDs and create a new App ID for your React Native app.
- Enable the Apple Pay capability for your App ID.
- Generate and download a Merchant ID certificate for your app. This certificate is essential for securely processing payments.
- Finally, configure your merchant settings, including specifying the payment processor you’ll use, on the Apple Developer website.
Once you’ve completed these steps, you’ll be ready to integrate Apple Pay into your React Native app.
Step 2: Install Dependencies
To work with Apple Pay in React Native, you’ll need to install some dependencies. The most crucial one is the react-native-in-app-utils package, which provides the necessary APIs for handling in-app payments.
Open your terminal and navigate to your React Native project directory. Then, run the following command to install the package:
bash npm install react-native-in-app-utils --save
After installing this package, you may need to link it to your project using the following command:
bash react-native link react-native-in-app-utils
Step 3: Set Up Your Payment Processor
Before you can accept payments via Apple Pay, you’ll need to set up your chosen payment processor. We’ll use Stripe as an example in this guide. Follow these steps to set up Stripe in your React Native project:
1. Install the Stripe React Native SDK
Stripe offers a dedicated SDK for React Native that simplifies payment processing. Install it by running the following command:
bash npm install @stripe/stripe-react-native --save
2. Configure Stripe
In your project, create a file to configure Stripe. You can name it stripeConfig.js and place it in the root directory. Here’s an example configuration:
javascript import { StripeProvider } from '@stripe/stripe-react-native'; const stripeConfig = { publishableKey: 'your-publishable-key', }; export const stripe = new StripeProvider(stripeConfig);
Replace ‘your-publishable-key’ with your actual publishable API key from your Stripe account.
3. Initialize Stripe in Your App
In your app’s entry file (usually index.js or App.js), import and initialize Stripe using the following code:
javascript import { stripe } from './stripeConfig'; stripe.init();
With Stripe set up, you can now move on to implementing Apple Pay.
Step 4: Implement Apple Pay
Integrating Apple Pay into your React Native app involves several steps:
1. Request User Consent
Before processing payments with Apple Pay, you need to request the user’s consent. Use the following code to do so:
javascript import { requestApplePay } from 'react-native-in-app-utils'; async function requestPayment() { try { const canMakePayments = await requestApplePay(); if (canMakePayments) { // User can make payments with Apple Pay } else { // User cannot make payments with Apple Pay } } catch (error) { console.error('Error checking Apple Pay availability:', error); } }
This code checks whether the user’s device supports Apple Pay and if they have set it up.
2. Create a Payment Request
Next, create a payment request object that defines the details of the transaction, such as the amount, currency, and payment items. Here’s an example:
javascript const paymentRequest = { merchantIdentifier: 'your-merchant-id', supportedNetworks: ['visa', 'mastercard', 'amex'], countryCode: 'US', currencyCode: 'USD', paymentSummaryItems: [ { label: 'Total Amount', amount: '10.00', }, ], };
Replace ‘your-merchant-id’ with your actual merchant identifier obtained from your Apple Developer account.
3. Present the Apple Pay Sheet
To initiate the Apple Pay process, present the payment request as a sheet to the user. You can use the following code:
javascript import { presentApplePay } from 'react-native-in-app-utils'; async function initiateApplePay() { try { const paymentResponse = await presentApplePay(paymentRequest); if (paymentResponse && paymentResponse.status === 'success') { // Payment successful } else { // Payment failed or user canceled } } catch (error) { console.error('Error processing Apple Pay payment:', error); } }
This code opens the Apple Pay sheet, allowing the user to select their payment method and complete the transaction.
4. Handle Payment Response
After the user completes the payment, you’ll receive a payment response. Handle it to update your app’s UI or perform any necessary actions. The response object will contain information about the payment status and a payment method token that you can use for server-side processing if needed.
javascript if (paymentResponse && paymentResponse.status === 'success') { const paymentMethodToken = paymentResponse.token; // Process payment method token (e.g., send it to your server for validation) // Update UI to reflect successful payment } else { // Handle payment failure or user cancellation }
And that’s it! You’ve successfully integrated Apple Pay into your React Native app.
Step 5: Testing and Debugging
Before deploying your app with Apple Pay integration, thorough testing is crucial. Here are some tips for testing and debugging:
1. Use a Sandbox Environment
Most payment processors offer sandbox environments that allow you to test payments without actual money. Make use of these environments to ensure your integration works as expected.
2. Test on Real Devices
Always test Apple Pay on real iOS devices, as emulators do not support it.
3. Handle Errors Gracefully
Implement error handling to gracefully handle scenarios where payments fail or users cancel transactions. Provide clear error messages to guide users.
4. Monitor Server-Side Processing
If your app relies on server-side processing for payments, monitor your server logs to ensure that payment method tokens are processed correctly.
Conclusion
Integrating Apple Pay into your React Native app can significantly enhance the user experience by providing a secure and convenient in-app payment method. By following the steps outlined in this guide and using the provided code samples, you can seamlessly implement Apple Pay and offer your users a hassle-free payment experience.
Remember to test thoroughly, keep your app updated with the latest SDKs, and prioritize security to ensure a smooth payment process for your users. With Apple Pay integration, your React Native app is ready to excel in the competitive world of mobile commerce.
Now, go ahead and revolutionize your app’s payment experience with Apple Pay!
In this comprehensive guide, we’ve covered the integration of Apple Pay into your React Native app, from setting up your Apple Developer account to implementing in-app payments. With the benefits of enhanced user experience, trust, and global reach, integrating Apple Pay is a valuable addition to any mobile app. Follow the steps and best practices outlined here to provide your users with a secure and convenient payment option that will set your app apart from the competition. Happy coding!
Table of Contents
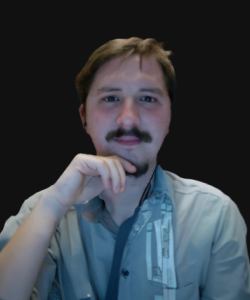
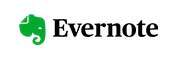