React Native and Google Maps: Integrating Maps and Location Services
React Native has gained immense popularity for building cross-platform mobile applications with native-like performance. One of the key features that can greatly enhance the user experience in many apps is integrating maps and location services. Whether you’re building a ride-sharing app, a food delivery service, or simply want to display maps with custom markers, React Native and Google Maps make it easier than ever to achieve this. In this comprehensive guide, we’ll walk you through the process of integrating Google Maps and location services into your React Native app, complete with code samples and step-by-step instructions.
Table of Contents
1. Prerequisites
Before we dive into the integration process, make sure you have the following prerequisites in place:
1.1. Node.js and npm/yarn
Ensure you have Node.js installed on your system. You can download it from the official Node.js website.
1.2. React Native CLI
You should have the React Native CLI installed globally on your system. You can install it using npm with the following command:
bash npm install -g react-native-cli
1.3. Google Cloud Platform (GCP) Account
To use Google Maps and location services, you’ll need to create a project on the Google Cloud Platform and enable the necessary APIs. Follow the steps in Google’s Get Started with Google Maps Platform guide to set up your project.
1.4. API Key
Once your GCP project is set up, create an API key. You’ll need this key to access Google Maps and location services in your React Native app.
2. Setting Up Your React Native Project
Now that you have all the prerequisites in place, let’s create a new React Native project and set up the necessary dependencies.
2.1. Create a New React Native Project
Open your terminal and run the following commands to create a new React Native project:
bash npx react-native init MapApp cd MapApp
2.2. Install Required Dependencies
To integrate Google Maps into your React Native app, you’ll need to install a few packages. The most commonly used package for this purpose is react-native-maps. Install it along with react-native-dotenv for managing environment variables:
bash npm install react-native-maps react-native-dotenv
2.3. Link Dependencies
After installing the packages, link them to your project:
bash react-native link react-native-maps
2.4. Configure Environment Variables
Create a .env file in the root of your project and add your Google Maps API key like this:
env GOOGLE_MAPS_API_KEY=YOUR_API_KEY_HERE
Replace YOUR_API_KEY_HERE with the API key you obtained from the Google Cloud Platform.
3. Creating a Simple Map Component
With the initial setup complete, let’s create a simple React Native component to display a map.
3.1. Import Required Libraries
In your project directory, navigate to the file where you want to create your map component (e.g., MapScreen.js). Import the necessary libraries:
javascript import React from 'react'; import { View, StyleSheet } from 'react-native'; import MapView from 'react-native-maps'; import { GOOGLE_MAPS_API_KEY } from 'react-native-dotenv';
3.2. Create the Map Component
Now, create a functional component called MapScreen:
javascript const MapScreen = () => { return ( <View style={styles.container}> <MapView style={styles.map} initialRegion={{ latitude: 37.78825, longitude: -122.4324, latitudeDelta: 0.0922, longitudeDelta: 0.0421, }} /> </View> ); };
In this code, we’ve used the MapView component from react-native-maps to display the map. We’ve also set an initial region with specific latitude and longitude coordinates.
3.3. Apply Styles
Define the styles for your map component:
javascript const styles = StyleSheet.create({ container: { ...StyleSheet.absoluteFillObject, justifyContent: 'flex-end', alignItems: 'center', }, map: { ...StyleSheet.absoluteFillObject, }, });
The styles ensure that the map takes up the entire screen.
3.4. Export the Component
Don’t forget to export your MapScreen component:
javascript export default MapScreen;
4. Integrating Google Maps API
Now that we have our basic map component, let’s integrate the Google Maps API and use our API key to enable advanced features.
4.1. Enable Google Maps JavaScript API
In your GCP project, navigate to the “APIs & Services” > “Library” section and enable the “Google Maps JavaScript API” for your project.
4.2. Enable Geocoding API
To enable location services, also enable the “Geocoding API.”
4.3. Configure API Key Restrictions
For security, restrict your API key by setting referrer restrictions and application restrictions in the GCP console.
4.4. Load Google Maps
Back in your MapScreen.js file, import the MapView component and use your API key to load Google Maps:
javascript import React from 'react'; import { View, StyleSheet } from 'react-native'; import MapView, { PROVIDER_GOOGLE } from 'react-native-maps'; import { GOOGLE_MAPS_API_KEY } from 'react-native-dotenv';
In the MapView component, add the provider prop to specify that you want to use Google Maps as the map provider:
javascript <MapView provider={PROVIDER_GOOGLE} style={styles.map} initialRegion={{ latitude: 37.78825, longitude: -122.4324, latitudeDelta: 0.0922, longitudeDelta: 0.0421, }} />
Now, your app should display Google Maps using the API key you provided.
5. Adding Markers and Current Location
To make your map more interactive and useful, you can add markers and display the user’s current location.
5.1. Adding a Marker
To add a marker to your map, you can use the Marker component from react-native-maps. Let’s add a marker for a specific location:
javascript <MapView provider={PROVIDER_GOOGLE} style={styles.map} initialRegion={{ latitude: 37.78825, longitude: -122.4324, latitudeDelta: 0.0922, longitudeDelta: 0.0421, }} > <MapView.Marker coordinate={{ latitude: 37.78825, longitude: -122.4324 }} title="Marker Title" description="Marker Description" /> </MapView>
In this example, we’ve added a marker at the coordinates (latitude: 37.78825, longitude: -122.4324) with a title and description.
5.2. Displaying the User’s Current Location
To display the user’s current location, you can use the MapView component’s showsUserLocation prop. First, import the MapView component with the MapView module:
javascript import MapView, { PROVIDER_GOOGLE, Marker } from 'react-native-maps';
Then, add the showsUserLocation prop to your MapView component:
javascript <MapView provider={PROVIDER_GOOGLE} style={styles.map} initialRegion={{ latitude: 37.78825, longitude: -122.4324, latitudeDelta: 0.0922, longitudeDelta: 0.0421, }} showsUserLocation={true} > {/* Add your markers here */} </MapView>
With showsUserLocation set to true, your app will display the user’s current location on the map.
6. Handling User Permissions
To access the user’s location, you need to request permission. Here’s how you can handle permissions in React Native:
6.1. Install Dependencies
Install the react-native-permissions library to manage permissions:
bash npm install react-native-permissions
6.2. Request Location Permission
Import the necessary modules and request location permission:
javascript import { useEffect } from 'react'; import { Platform } from 'react-native'; import Geolocation from 'react-native-geolocation-service'; import { request, PERMISSIONS } from 'react-native-permissions'; const MapScreen = () => { useEffect(() => { if (Platform.OS === 'android') { request(PERMISSIONS.ANDROID.ACCESS_FINE_LOCATION).then((result) => { if (result === 'granted') { Geolocation.getCurrentPosition( (position) => { console.log(position.coords); }, (error) => { console.log(error); }, { enableHighAccuracy: true, timeout: 15000, maximumAge: 10000 } ); } }); } }, []); // ... rest of the component };
This code checks the platform (Android or iOS), requests location permission for Android, and retrieves the current position using the Geolocation module.
6.3. Handle iOS Permissions
For iOS, you can use the following code:
javascript if (Platform.OS === 'ios') { request(PERMISSIONS.IOS.LOCATION_WHEN_IN_USE).then((result) => { if (result === 'granted') { Geolocation.getCurrentPosition( (position) => { console.log(position.coords); }, (error) => { console.log(error); }, { enableHighAccuracy: true, timeout: 15000, maximumAge: 10000 } ); } }); }
This code requests location permission for iOS when the app is in use.
Conclusion
Integrating Google Maps and location services into your React Native app can greatly enhance its functionality and user experience. In this guide, you’ve learned how to set up your project, create a simple map component, integrate the Google Maps API, add markers, display the user’s current location, and handle permissions.
With these foundational skills, you can build a wide range of location-aware apps, from navigation and location-based services to geotagging and social networking. Experiment with different features and explore the rich capabilities of React Native and Google Maps to create engaging and interactive mobile applications. Happy coding!
Remember that this is just the beginning of your journey in building location-based React Native apps. Continue to explore the documentation and experiment with advanced features to create innovative and useful applications that leverage the power of maps and location services.
Table of Contents
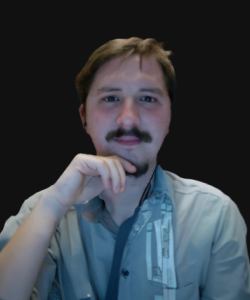
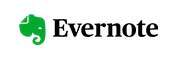