React Native and Image Recognition: Integrating ML Vision
In the ever-evolving world of mobile app development, React Native has proven to be a game-changer. Its ability to create cross-platform applications with a single codebase has made it a favorite among developers. But what if you could take your React Native app to the next level by adding image recognition capabilities powered by machine learning (ML)? In this blog, we’ll explore how to seamlessly integrate ML Vision into your React Native app to unlock a world of possibilities.
Table of Contents
1. Understanding ML Vision
Before we dive into the nitty-gritty of integration, let’s get a grasp of what ML Vision is and how it can benefit your React Native app.
1.1. What is ML Vision?
ML Vision is a part of Google’s Machine Learning Kit (ML Kit) that allows developers to integrate powerful image recognition capabilities into their applications. It leverages Google’s machine learning models to perform tasks like text recognition, face detection, barcode scanning, and label recognition in images and videos.
1.2. Benefits of ML Vision
Integrating ML Vision into your React Native app can provide several key benefits:
- Enhanced User Experience: ML Vision can analyze and interpret images in real-time, allowing your app to respond intelligently to visual cues, providing users with a more interactive experience.
- Increased Efficiency: Automate tasks such as data extraction from images, reducing manual effort and improving the efficiency of your app.
- Wider App Capabilities: Open up new possibilities for your app, such as building augmented reality features, content recommendations, and more.
Now that we understand the potential of ML Vision, let’s dive into the integration process.
2. Prerequisites
Before we begin, make sure you have the following prerequisites in place:
- React Native Development Environment: Ensure that you have React Native set up on your machine. If not, follow the official React Native Getting Started guide.
- Google Cloud Platform (GCP) Account: ML Vision relies on Google’s machine learning models, so you’ll need a GCP account to access the required APIs and services.
- React Native Project: Start with an existing React Native project or create a new one using react-native init.
Step 1: Set Up Google Cloud Platform
To get started with ML Vision, you need to set up a project in Google Cloud Platform (GCP) and enable the Vision API. Here’s how:
Create a GCP Project:
- Go to the Google Cloud Console.
- Click on the project drop-down and select “New Project.”
- Follow the prompts to create a new project. Give it a name and select your billing account.
Enable the Vision API:
- In the Google Cloud Console, navigate to the “APIs & Services” > “Dashboard” section.
- Click on the “+ ENABLE APIS AND SERVICES” button.
- Search for “Cloud Vision API” and select it.
- Click the “Enable” button.
Generate API Key:
- In the Google Cloud Console, navigate to “APIs & Services” > “Credentials.”
- Click on the “+ CREATE CREDENTIALS” button and select “API key.”
- Copy the generated API key; you’ll need it to authenticate your React Native app with the Vision API.
Step 2: Set Up React Native Project
With the GCP configuration in place, let’s move on to setting up your React Native project to work with ML Vision.
Install Required Packages:
Open your terminal and navigate to your React Native project’s root directory. Run the following command to install the necessary packages:
bash npm install @react-native-firebase/app @react-native-firebase/ml-vision
These packages will help you interact with Firebase, which will be used to authenticate your app with GCP and utilize the Vision API.
Initialize Firebase:
After installing the packages, you need to initialize Firebase in your React Native app. This step is crucial for setting up authentication and linking your app to GCP.
javascript // In your app's entry file (e.g., App.js) import { AppRegistry } from 'react-native'; import { FirebaseAppProvider } from '@react-native-firebase/app'; const firebaseConfig = { apiKey: 'YOUR_API_KEY', authDomain: 'YOUR_AUTH_DOMAIN', projectId: 'YOUR_PROJECT_ID', storageBucket: 'YOUR_STORAGE_BUCKET', messagingSenderId: 'YOUR_MESSAGING_SENDER_ID', appId: 'YOUR_APP_ID', }; function App() { return ( <FirebaseAppProvider firebaseConfig={firebaseConfig}> {/* Your app components */} </FirebaseAppProvider> ); } AppRegistry.registerComponent('App', () => App);
Replace the placeholder values in firebaseConfig with your GCP project’s details.
Configure ML Vision:
To configure ML Vision, you’ll need to import the necessary functions and initialize Firebase ML Vision in your app. Add the following code to your app:
javascript // In your app's entry file (e.g., App.js) import { FirebaseAppProvider } from '@react-native-firebase/app'; import { FirebaseVisionProvider } from '@react-native-firebase/ml-vision'; const firebaseConfig = { // ... (your Firebase configuration) }; function App() { return ( <FirebaseAppProvider firebaseConfig={firebaseConfig}> <FirebaseVisionProvider> {/* Your app components */} </FirebaseVisionProvider> </FirebaseAppProvider> ); } AppRegistry.registerComponent('App', () => App);
This code sets up Firebase and initializes Firebase ML Vision.
Step 3: Implement Image Recognition
Now that you’ve set up your project, it’s time to implement image recognition using ML Vision. In this example, we’ll create a simple React Native component that allows users to upload an image and get labels for objects detected in the image.
Create a React Native Component:
Create a new component in your project (e.g., ImageRecognition.js) and add the following code:
javascript import React, { useState } from 'react'; import { View, Text, Image, Button, ActivityIndicator } from 'react-native'; import { FirebaseVisionImage, FirebaseVision } from '@react-native-firebase/ml-vision'; const ImageRecognition = () => { const [imageUri, setImageUri] = useState(null); const [labels, setLabels] = useState([]); const [loading, setLoading] = useState(false); const processImage = async () => { setLoading(true); try { const image = await FirebaseVisionImage.fromFilePath(imageUri); const vision = FirebaseVision.instance().visionLabelDetector(); const detectedLabels = await vision.processImage(image); const labelDescriptions = detectedLabels.map((label) => label.label); setLabels(labelDescriptions); } catch (error) { console.error('Error processing image:', error); } finally { setLoading(false); } }; const selectImage = async () => { // Implement image selection logic here }; return ( <View> <Button title="Select Image" onPress={selectImage} /> {imageUri && ( <Image source={{ uri: imageUri }} style={{ width: 200, height: 200 }} /> )} {loading && <ActivityIndicator size="large" />} {labels.length > 0 && ( <View> <Text>Detected Labels:</Text> {labels.map((label, index) => ( <Text key={index}>{label}</Text> ))} </View> )} </View> ); }; export default ImageRecognition;
This component initializes the ML Vision Label Detector, allows the user to select an image, and processes the selected image to detect labels.
Implement Image Selection:
You’ll need to implement the selectImage function to allow users to choose an image from their device’s gallery. You can use libraries like react-native-image-picker for this purpose.
Render the Component:
Finally, render the ImageRecognition component within your app’s navigation or wherever you want to use image recognition.
Step 4: Test and Optimize
With image recognition implemented in your React Native app, it’s time to test and optimize the feature. Here are a few tips:
- Test with Different Images: Ensure that your image recognition works well with various types of images to provide accurate results.
- Performance Optimization: Image recognition can be resource-intensive. Optimize your code and consider asynchronous processing to avoid blocking the main thread.
- UI/UX Improvements: Enhance the user interface by providing feedback during image processing, such as loading indicators or progress bars.
- Error Handling: Implement robust error handling to gracefully handle failures during image processing.
Conclusion
Integrating ML Vision into your React Native app opens up a world of possibilities for enhancing user experiences and automating tasks. With the power of machine learning, you can create intelligent apps that can interpret and respond to visual data. By following the steps outlined in this guide, you’re well on your way to adding image recognition capabilities to your React Native project. Experiment, iterate, and unlock the full potential of ML Vision in your app development journey. Happy coding!
Table of Contents
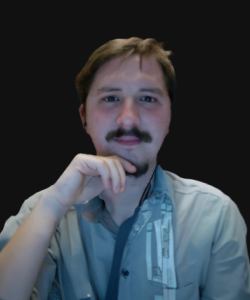
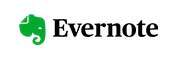