How to Hire React Native Developers – A Comprehensive Interview Guide
React Native has become a leading framework for building cross-platform mobile applications, creating a high demand for skilled React Native developers worldwide. To ensure you find the perfect fit for your projects, it’s crucial to assess candidates’ technical abilities, problem-solving skills, and experience with React Native and its ecosystem.
Table of Contents
This article will serve as your comprehensive interview guide for hiring React Native developers.
1. Top Skills of React Native Developers to Look Out For:
Before diving into the interview process, let’s highlight the key skills you should look for in React Native developers:
- Strong JavaScript Fundamentals: React Native is built on JavaScript, making proficiency in this language essential.
- React Native Expertise: Candidates should have substantial experience working on React Native projects.
- Component-Based Architecture: React Native follows a component-based approach, so candidates should understand how components work and interact.
- State Management Libraries: Familiarity with state management libraries like Redux or MobX is beneficial.
- API Integration: Look for candidates experienced in making API calls and handling data in React Native apps.
- Collaboration and Version Control: Candidates should be comfortable working with version control systems like Git and collaborating with team members.
2. Interview Questions and Sample Answers:
Q1. Explain the concept of React Native Bridge and how it enables communication between JavaScript and native modules.
Sample Answer: “The React Native Bridge is a communication mechanism that allows JavaScript code to interact with native modules written in Objective-C (iOS) and Java (Android). It facilitates seamless integration of native functionality into React Native apps. JavaScript code can call methods on the native module, and vice versa, using the bridge.”
Q2. How do you handle screen navigation and routing in React Native? Are there any navigation libraries you prefer?
Sample Answer: “For screen navigation in React Native, I typically use a navigation library like React Navigation or React Native Navigation. These libraries provide easy-to-use APIs for handling navigation and routing between screens in a React Native app. They offer stack, tab, drawer, and bottom tab navigators to suit various navigation patterns.”
Q3. What are the performance optimization techniques you follow in React Native development?
Sample Answer: “To optimize React Native app performance, I follow several practices, such as using the ‘shouldComponentUpdate’ method to prevent unnecessary re-renders, utilizing memoization for expensive computations, and lazy-loading components to improve initial app load time. I also use the ‘FlatList’ component for efficiently rendering lists with large data sets.”
Q4. How do you handle platform-specific code in React Native applications?
Sample Answer: “React Native allows us to write platform-specific code using the ‘Platform’ module. We can conditionally render components or apply styles based on the platform (iOS or Android). For example:
import { Platform } from 'react-native'; const MyComponent = () => { return ( <View> {Platform.OS === 'ios' ? <IOSComponent /> : <AndroidComponent />} </View> ); };
This ensures that platform-specific code is executed only on the appropriate platform.”
Q5. Can you explain the concept of React Native Gesture Handler, and how it improve touch and gesture interactions in apps?
Sample Answer: “React Native Gesture Handler is a library that improves touch and gesture interactions in React Native apps. It uses a native thread to handle gestures, resulting in smoother and more responsive user interactions. With Gesture Handler, we can handle complex gestures like swipes, pinches, and pans more efficiently.”
Q6. How do you handle app localization and internationalization in a React Native project?
Sample Answer: “To support app localization and internationalization, I use the ‘react-i18next’ library in combination with React Native’s built-in ‘Localization’ module. This allows me to define multiple language files and switch between them based on the user’s device settings or user preferences.”
Q7. What are the debugging tools you use while developing React Native applications?
Sample Answer: “For debugging React Native apps, I primarily rely on React Native Debugger, which provides a powerful interface for inspecting component hierarchy, state, and props. Additionally, I use React DevTools for inspecting component lifecycles and Redux DevTools for monitoring state changes when using Redux.”
Q8. How do you handle app security and prevent common security vulnerabilities in React Native projects?
Sample Answer: “To ensure app security, I follow best practices such as using HTTPS for network requests, sanitizing user inputs to prevent injection attacks, and storing sensitive data securely using encryption. Additionally, I update all dependencies regularly to address potential security vulnerabilities.”
Q9. Have you integrated native modules or third-party native libraries into React Native apps? If so, explain the process and any challenges faced.
Sample Answer: “Yes, I have integrated native modules and third-party libraries in React Native apps. For native modules, I created custom native code in Objective-C or Java and bridged it to JavaScript using the React Native Bridge. For third-party native libraries, I used tools like ‘react-native link’ to automate the linking process. Challenges often revolved around version compatibility and ensuring proper documentation.”
Q10. How do you handle app state persistence and manage data between app launches in React Native?
Sample Answer: “To persist app state, I use ‘AsyncStorage’ or other storage solutions like ‘redux-persist.’ AsyncStorage allows me to save key-value pairs on the device, enabling data persistence between app launches. For complex state management, I prefer using Redux with ‘redux-persist’ to save and rehydrate the store across app restarts.”
Remember, the goal of these questions is to assess the candidate’s practical knowledge and problem-solving abilities related to React Native development. Feel free to modify or add more questions based on your specific project requirements and expectations from the candidate.
Q11. Can you explain the core principles of React Native and how it differs from React for web development?
Sample Answer: “React Native allows us to build mobile applications using JavaScript and React-like components. It uses a bridge to communicate with native modules, enabling cross-platform development. React for web focuses on rendering components to the DOM, while React Native renders to native components on iOS and Android devices, delivering a native look and feel.”
Q12. How do you create and use components in React Native?
Sample Answer: “In React Native, components are the building blocks of the UI. We can create components using functional or class-based syntax. Here’s an example of a functional component:
import React from 'react'; import { View, Text } from 'react-native'; const MyComponent = () => { return ( <View> <Text>Hello, React Native!</Text> </View> ); }; export default MyComponent;
We can use this component by importing and rendering it within other components.”
Q13. How do you manage state in a React Native application?
Sample Answer: “State management is crucial in React Native apps. We can use the ‘useState’ hook to manage state in functional components and ‘this.state’ in class-based components. Here’s an example using the ‘useState’ hook:
import React, { useState } from 'react'; import { View, Button } from 'react-native'; const Counter = () => { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <View> <Button title="Increment" onPress={increment} /> <Text>{count}</Text> </View> ); }; export default Counter;
The ‘useState’ hook allows us to update and access the ‘count’ state in a functional component.”
Q14. How do you handle asynchronous operations like fetching data or handling network requests in React Native?
Sample Answer: “In React Native, we can handle asynchronous operations using async/await or promises. Here’s an example of fetching data using the ‘fetch’ API:
import React, { useState, useEffect } from 'react'; import { View, Text } from 'react-native'; const DataDisplay = () => { const [data, setData] = useState(null); useEffect(() => { const fetchData = async () => { try { const response = await fetch('https://api.example.com/data'); const jsonData = await response.json(); setData(jsonData); } catch (error) { console.error('Error fetching data:', error); } }; fetchData(); }, []); return ( <View> {data ? <Text>{data}</Text> : <Text>Loading...</Text>} </View> ); }; export default DataDisplay;
This example fetches data from an API and updates the state ‘data’ with the response.”
3. The Importance of the Interview Process in Hiring React Native Talent
Hiring the right React Native talent is crucial for the success of your mobile app development projects. An effective interview process allows you to assess candidates’ technical skills, problem-solving abilities, and overall fit with your team and company culture. Finding candidates with the right expertise and experience ensures that your projects are delivered on time and meet the highest standards of quality.
3.1 Technical Expertise and Problem-Solving Abilities
React Native development requires a strong foundation in JavaScript, React concepts, and mobile app development principles. By asking relevant technical questions and presenting coding challenges, you can gauge the candidate’s proficiency in React Native and their ability to solve real-world problems. A thorough evaluation of their coding skills provides insights into their coding style, code organization, and overall approach to development.
3.2 Cultural Fit and Team Collaboration
Beyond technical expertise, evaluating cultural fit is equally important. A developer who aligns with your company’s values and team dynamics is more likely to contribute positively to your projects. During the interview process, assessing soft skills like communication, teamwork, and adaptability helps determine how well the candidate will integrate into your existing development team.
4. How CloudDevs Can Help You Find the Right React Native Developers
The hiring process can be time-consuming and resource-intensive, especially when searching for top React Native developers. This is where platforms like CloudDevs can be instrumental in streamlining the recruitment process.
CloudDevs is a developer platform that offers a curated pool of pre-screened senior developers with expertise in various technologies, including React Native. By partnering with CloudDevs, you gain access to a pool of highly skilled React Native developers without the hassle of conducting extensive interviews and technical assessments.
With CloudDevs, the process of finding the right React Native talent becomes straightforward:
- Consultation and Project Requirements: Start by discussing your project requirements with a CloudDevs consultant. Outline the skills and experience you need in a React Native developer, along with the project timeline and scope.
- Talent Match: Within 24 hours, CloudDevs presents you with shortlisted candidates who match your requirements from their pool of experienced developers. You can review their profiles and select the most suitable fit for your team.
- No-Risk Trial Period: Once you’ve identified a potential candidate, you can get acquainted with them through a call and start a week-long no-risk trial period. This allows you to assess their technical skills and compatibility with your team before making a final decision.
By leveraging CloudDevs’ expertise in talent curation and pre-screening, you save valuable time and resources, ensuring that you only interview candidates who have already been vetted for their technical proficiency and communication skills. This way, you can confidently choose the best-fit React Native developer for your projects, accelerating your development process and delivering high-quality mobile applications.
5. Conclusion
The interview process is a critical step in hiring the right React Native developers who can contribute to your projects’ success. By evaluating technical expertise, problem-solving abilities, and cultural fit, you can identify candidates who align with your project requirements and team dynamics.
Platforms like CloudDevs streamline the hiring process by providing access to a pool of pre-screened React Native developers, saving you time and effort. Partnering with CloudDevs ensures that you find top-tier React Native talent, enabling your company to build cutting-edge mobile applications that exceed customer expectations and drive business growth.
Table of Contents
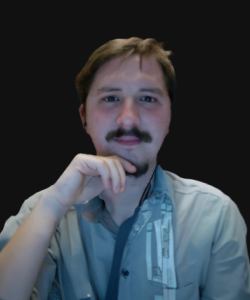
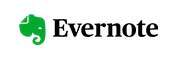