Integrating React Native with Microsoft Cognitive Services
In the rapidly evolving landscape of mobile app development, the fusion of artificial intelligence (AI) capabilities with intuitive user interfaces has become a game-changer. React Native, with its cross-platform flexibility and robust performance, has emerged as a preferred framework for building powerful mobile applications. When combined with Microsoft Cognitive Services, a comprehensive suite of AI tools and APIs, React Native opens up a realm of possibilities for developers to create intelligent, feature-rich applications.
Why Integrate React Native with Microsoft Cognitive Services?
Microsoft Cognitive Services provides developers with pre-trained AI models and APIs to add a variety of intelligent features to their applications, ranging from computer vision and natural language processing to speech recognition and emotion detection. By integrating these services with React Native, developers can enhance user experiences, automate tasks, and unlock new functionalities without the need for extensive AI expertise or infrastructure setup.
How to Integrate React Native with Microsoft Cognitive Services
Step 1: Set Up Your React Native Project
Begin by creating a new React Native project or navigating to an existing one.
bash npx react-native init YourProjectName cd YourProjectName
Step 2: Install Necessary Dependencies
Install the required packages for integrating Microsoft Cognitive Services into your React Native project.
npm install @react-native-community/netinfo @react-native-async-storage/async-storage react-native-camera @react-native-voice/voice
Step 3: Obtain API Keys
Sign up for Microsoft Azure and generate API keys for the Cognitive Services you wish to utilize, such as Azure Computer Vision, Azure Speech Service, or Azure Text Analytics.
Step 4: Implement Cognitive Services APIs
Utilize the installed packages and API keys to integrate desired Cognitive Services functionalities into your React Native app. Below are some examples:
Example 1: Image Recognition with Azure Computer Vision
import { ComputerVisionClient } from '@azure/cognitiveservices-computervision'; import { AzureKeyCredential } from '@azure/core-auth'; const client = new ComputerVisionClient(endpoint, new AzureKeyCredential(apiKey)); const analyzeImage = async (imageUri) => { const results = await client.analyzeImage(imageUri); console.log(results); };
Example 2: Speech Recognition with Azure Speech Service
import * as SpeechSDK from 'microsoft-cognitiveservices-speech-sdk'; const speechConfig = SpeechSDK.SpeechConfig.fromSubscription(apiKey, region); const audioConfig = SpeechSDK.AudioConfig.fromDefaultMicrophoneInput(); const recognizer = new SpeechSDK.SpeechRecognizer(speechConfig, audioConfig); const recognizeSpeech = async () => { recognizer.recognizeOnceAsync(result => { console.log(result.text); }); };
Example 3: Text Sentiment Analysis with Azure Text Analytics
import { TextAnalyticsClient } from '@azure/ai-text-analytics'; import { AzureKeyCredential } from '@azure/core-auth'; const client = new TextAnalyticsClient(endpoint, new AzureKeyCredential(apiKey)); const analyzeSentiment = async (text) => { const result = await client.analyzeSentiment([text]); console.log(result[0].sentiment); };
Step 5: Test and Iterate
Thoroughly test the integrated functionalities within your React Native app, ensuring seamless performance and user experience. Iterate as needed to refine and optimize the implementation.
Real-world Examples
- Prisma: This mobile app leverages React Native and Microsoft Cognitive Services for image recognition to assist visually impaired users in identifying objects and navigating their surroundings.
- HealthPal: HealthPal utilizes React Native alongside Azure Cognitive Services to provide personalized health recommendations and symptom analysis based on user input and medical history.
- TravelBuddy: Integrating React Native with Microsoft Cognitive Services, TravelBuddy offers real-time translation capabilities, enabling travelers to communicate effortlessly in foreign languages.
Conclusion
Integrating React Native with Microsoft Cognitive Services empowers developers to infuse AI capabilities into their mobile applications seamlessly. Whether it’s enhancing image recognition, enabling speech-to-text functionality, or performing sentiment analysis, the possibilities are endless. By harnessing the power of AI, developers can create intuitive, intelligent mobile experiences that redefine the boundaries of what’s possible.
Embrace the fusion of React Native and Microsoft Cognitive Services to unlock the full potential of your mobile app development journey!
External Resources:
- Microsoft Azure Cognitive Services Documentation
- React Native Documentation
- Azure Cognitive Services SDK for JavaScript
Incorporating Microsoft Cognitive Services into React Native apps opens up a world of AI-driven possibilities. Start building intelligent mobile experiences today!
Table of Contents
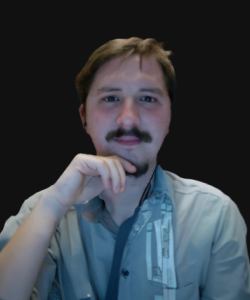
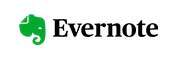