Performance Optimization in React Native: Techniques for Faster Apps
In the world of mobile app development, performance is king. Users expect applications to be fast, responsive, and fluid in their interactions. This is particularly true for React Native, a popular framework that enables developers to build native mobile apps using JavaScript and React. While React Native offers a lot of advantages, ensuring optimal performance can be a challenging task. In this article, we’ll explore various techniques and strategies to optimize the performance of your React Native apps and deliver a smoother user experience.
1. Why Performance Optimization Matters
Before diving into optimization techniques, let’s understand why performance is crucial for the success of your React Native app. Slow and unresponsive apps can lead to user frustration, high bounce rates, and negative reviews on app stores. On the other hand, well-optimized apps can result in increased user engagement, longer session durations, and better overall user satisfaction.
2. Rendering Optimization
2.1 Reconciliation Process
React Native employs a virtual DOM to manage UI updates efficiently. However, unnecessary re-renders can impact performance. To address this, use the React.memo higher-order component to prevent the re-rendering of functional components unless their props change.
jsx import React, { memo } from 'react'; const MyComponent = memo(({ data }) => { // Component rendering logic... }); export default MyComponent;
2.2 Virtualized Lists
For long lists, consider using the FlatList or SectionList components. These components efficiently render only the visible items on the screen, reducing the memory footprint and enhancing overall performance.
jsx import { FlatList } from 'react-native'; const MyList = () => { const data = [...]; // Your list data return ( <FlatList data={data} renderItem={({ item }) => <ListItem data={item} />} keyExtractor={(item) => item.id.toString()} /> ); };
2. Bundle Size Optimization
2.1 Code Splitting
Large bundle sizes can lead to slower app startup times. React Native supports code splitting using dynamic imports, allowing you to load specific components or modules only when they are needed.
jsx import dynamic from 'react-native-dynamic'; const DynamicComponent = dynamic(() => import('./DynamicComponent'), { loading: () => <LoadingComponent />, });
2.2 Tree Shaking
Ensure your app’s bundle includes only the code that is actually used. Tree shaking, a technique supported by tools like Metro Bundler, removes unused code during the bundling process.
javascript // metro.config.js module.exports = { ... optimization: { minimize: true, usedExports: true, concatenateModules: true, }, };
3. Image and Asset Optimization
3.1 Use WebP Format
The WebP image format offers high compression rates without sacrificing quality. Use tools like react-native-webp to incorporate WebP images into your app.
bash npm install react-native-webp
3.2 Icon Fonts
Instead of using individual image assets for icons, consider using icon fonts like FontAwesome. This reduces the number of image requests and can lead to faster loading times.
bash npm install react-native-vector-icons
4. Memory Management
4.1 Avoid Memory Leaks
Improper memory management can lead to memory leaks and app crashes. Use tools like react-native-heapdump to identify memory leaks in your app.
bash npm install react-native-heapdump
4.2 Optimize State Management
Choose the right state management solution for your app. For local component state, use the useState hook, and for global state, consider using libraries like Redux or Mobx. However, be cautious not to overuse global state, as it can lead to unnecessary re-renders.
5. UI/UX Optimization
5.1 Gesture Responder System
React Native’s Gesture Responder System can sometimes cause performance issues, especially on complex gestures. Consider using the more performant react-native-gesture-handler library to improve gesture responsiveness.
bash npm install react-native-gesture-handler
5.2 Animations
Smooth animations enhance user experience but can also impact performance. Utilize the Animated API for optimized animations, and prefer transform and opacity animations over layout-related properties.
jsx import { Animated } from 'react-native'; const MyComponent = () => { const fadeAnim = new Animated.Value(0); Animated.timing(fadeAnim, { toValue: 1, duration: 1000, useNativeDriver: true, }).start(); return ( <Animated.View style={{ opacity: fadeAnim }}> {/* Content */} </Animated.View> ); };
Conclusion
React Native provides a powerful framework for developing cross-platform mobile apps, but achieving optimal performance requires deliberate effort. By following the techniques outlined in this article, you can create faster, more responsive, and more enjoyable experiences for your users. Remember that performance optimization is an ongoing process, and it’s essential to monitor your app’s performance regularly, especially as it evolves and grows.
Incorporating these performance optimization techniques into your React Native development workflow can make a significant difference in the speed and efficiency of your mobile applications. Users today demand apps that not only look great but also deliver seamless and lightning-fast interactions. By rendering efficiently, optimizing bundle sizes, managing memory effectively, and fine-tuning the user interface, you can provide a top-notch user experience that keeps users engaged and satisfied. So, apply these strategies, measure your app’s performance, and continually iterate to ensure that your React Native apps are performing at their best. Your users will thank you with increased engagement, positive reviews, and loyalty to your app.
Table of Contents
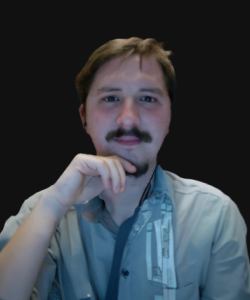
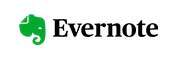