React Native and Redux Toolkit: Simplified State Management
In the ever-evolving landscape of app development, React Native has emerged as a powerful tool for building cross-platform applications with ease. Its ability to write code once and deploy it on both iOS and Android platforms has made it a favorite among developers. However, as applications grow in complexity, managing state becomes a crucial aspect of development. This is where Redux Toolkit comes into play, offering a simplified and efficient solution to state management in React Native applications.
Understanding State Management in React Native
In React Native, state management refers to the management of data that determines the behavior and appearance of components in an application. As an application grows, managing this state becomes increasingly challenging, leading to issues such as prop drilling and complex component hierarchies.
Traditionally, Redux has been a popular choice for state management in React applications. However, setting up Redux can be cumbersome, requiring boilerplate code and multiple layers of abstraction. This is where Redux Toolkit comes in, providing a set of tools and best practices to streamline the Redux workflow and simplify state management.
Introducing Redux Toolkit
Redux Toolkit is a package that includes several utilities to simplify Redux development, including:
- createSlice: A function that generates action creators and reducers for a slice of state, reducing the need for writing boilerplate code.
- configureStore: A function that combines reducers, middleware, and enhancers into a Redux store, providing a single source of truth for the application state.
- createAsyncThunk: A function that generates async action creators, enabling developers to handle asynchronous logic such as API requests with ease.
By leveraging Redux Toolkit, developers can write cleaner and more maintainable code, reducing the cognitive overhead associated with managing application state.
Simplified State Management with React Native and Redux Toolkit
Let’s walk through a practical example of how Redux Toolkit simplifies state management in a React Native application.
Example: Todo List Application
Suppose we’re building a simple todo list application in React Native. We want users to be able to add and delete tasks from the list. Here’s how we can use Redux Toolkit to manage the application state:
- Setting Up the Redux Store:
import { configureStore, createSlice } from '@reduxjs/toolkit'; const todoSlice = createSlice({ name: 'todos', initialState: [], reducers: { addTodo(state, action) { state.push(action.payload); }, deleteTodo(state, action) { return state.filter(todo => todo.id !== action.payload); }, }, }); export const { addTodo, deleteTodo } = todoSlice.actions; export default configureStore({ reducer: { todos: todoSlice.reducer, }, });
- Using Redux Toolkit in Components:
import React, { useState } from 'react'; import { View, TextInput, Button } from 'react-native'; import { useDispatch } from 'react-redux'; import { addTodo } from './store'; const TodoInput = () => { const [text, setText] = useState(''); const dispatch = useDispatch(); const handleAddTodo = () => { dispatch(addTodo({ id: Math.random().toString(), text })); setText(''); }; return ( <View> <TextInput value={text} onChangeText={setText} placeholder="Enter task" /> <Button onPress={handleAddTodo} title="Add Task" /> </View> ); }; export default TodoInput;
- Accessing State in Components:
import React from 'react'; import { View, Text, Button } from 'react-native'; import { useSelector, useDispatch } from 'react-redux'; import { deleteTodo } from './store'; const TodoList = () => { const todos = useSelector(state => state.todos); const dispatch = useDispatch(); const handleDeleteTodo = id => { dispatch(deleteTodo(id)); }; return ( <View> {todos.map(todo => ( <View key={todo.id}> <Text>{todo.text}</Text> <Button onPress={() => handleDeleteTodo(todo.id)} title="Delete" /> </View> ))} </View> ); }; export default TodoList;
In this example, Redux Toolkit simplifies the process of creating reducers and action creators using the createSlice function. The Redux store is configured using configureStore, providing a centralized location for managing application state. Components can then access and modify the state using hooks such as useSelector and useDispatch, leading to a cleaner and more modular codebase.
Conclusion
React Native and Redux Toolkit offer a powerful combination for building scalable and maintainable cross-platform applications. By leveraging Redux Toolkit, developers can simplify state management, reduce boilerplate code, and focus on building innovative features for their applications.
To learn more about React Native and Redux Toolkit, check out the following resources:
- React Native Documentation
- Redux Toolkit Documentation
- Building React Native Apps with Redux Toolkit – Tutorial
Happy coding!
Table of Contents
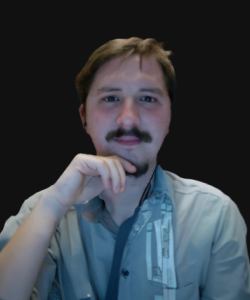
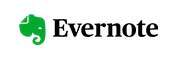