Using React Native with TypeScript: Type-Safe App Development
In the ever-evolving landscape of mobile app development, React Native has emerged as a game-changer, enabling developers to build cross-platform applications using a single codebase. With its ability to provide a native-like experience and expedited development cycles, React Native has become a preferred choice for many developers. When combined with TypeScript, a powerful and statically-typed superset of JavaScript, the development process becomes even more streamlined and secure.
Table of Contents
1. Benefits of Using React Native with TypeScript
1.1. Type Safety: The Foundation of Reliable Apps
One of the most significant advantages of incorporating TypeScript into your React Native projects is the inherent type safety it brings to the table. JavaScript, while flexible and dynamic, lacks the rigorous type-checking that can catch potential errors early in the development process. TypeScript, on the other hand, allows you to define types for your variables, props, states, and more. This feature not only enhances the quality of your code but also minimizes runtime errors by detecting type-related issues during development.
typescript // TypeScript Interface for a user object interface User { id: number; name: string; email: string; } const newUser: User = { id: 1, name: "John Doe", email: "john@example.com", };
1.2. Enhanced Developer Experience
TypeScript provides excellent development tools such as autocompletion, intelligent suggestions, and instant error detection directly in your code editor. This means you can catch and fix issues as you type, reducing the need for time-consuming debugging later on. This feature is particularly valuable in large-scale projects where maintaining code quality and consistency is a challenge.
1.3. Improved Code Maintainability
As React Native applications grow in complexity, maintaining code can become overwhelming. TypeScript’s strong typing system encourages developers to write more structured and self-documenting code. With clearly defined types and interfaces, you can understand the purpose of each component, prop, or function without diving deep into the implementation details.
2. Getting Started: Setting Up Your Project
Before you dive into building your app with React Native and TypeScript, you need to set up your project environment. Follow these steps to get started:
Step 1: Creating a New React Native Project
If you haven’t already, make sure you have Node.js and npm (Node Package Manager) installed. Then, open your terminal and run the following command to create a new React Native project:
bash npx react-native init YourProjectName
Step 2: Adding TypeScript to the Project
Navigate to your project directory and install the required dependencies to use TypeScript with React Native:
bash cd YourProjectName npm install --save-dev typescript @types/react @types/react-native
Step 3: Configuring TypeScript
Create a tsconfig.json file in the root of your project to configure TypeScript settings. Here’s a minimal configuration to get you started:
json { "compilerOptions": { "target": "esnext", "moduleResolution": "node", "jsx": "react-native", "strict": true } }
Step 4: Renaming Files to TypeScript
Change the file extensions of your React Native components from .js to .tsx to indicate TypeScript files. TypeScript will understand and parse JSX syntax within these files.
3. Writing Type-Safe React Native Components
With your project set up for TypeScript, let’s explore how to create type-safe React Native components. Consider a simple example of a user profile component:
tsx import React from 'react'; import { View, Text, Image } from 'react-native'; interface UserProfileProps { username: string; followers: number; avatarUrl: string; } const UserProfile: React.FC<UserProfileProps> = ({ username, followers, avatarUrl }) => ( <View> <Image source={{ uri: avatarUrl }} style={{ width: 100, height: 100 }} /> <Text>{username}</Text> <Text>Followers: {followers}</Text> </View> ); export default UserProfile;
In this example, the UserProfileProps interface defines the expected props for the UserProfile component. The TypeScript compiler will enforce that any usage of this component provides the required props in the correct format.
4. Handling State and Navigation
TypeScript’s benefits extend to handling component state and navigation as well. Let’s see how TypeScript helps in these scenarios.
4.1. Type-Safe State Management
Consider a counter component that utilizes React hooks for state management:
tsx import React, { useState } from 'react'; import { View, Button, Text } from 'react-native'; const Counter: React.FC = () => { const [count, setCount] = useState<number>(0); const increment = () => setCount(count + 1); const decrement = () => setCount(count - 1); return ( <View> <Button title="Increment" onPress={increment} /> <Text>Count: {count}</Text> <Button title="Decrement" onPress={decrement} /> </View> ); }; export default Counter;
In this example, TypeScript ensures that the count state is always treated as a number, and the increment and decrement functions are correctly associated with the button press event.
4.2. Type-Safe Navigation
For navigation between screens, you can use libraries like react-navigation. TypeScript aids in ensuring that the navigation parameters are correctly defined and passed between screens. Here’s a basic example:
tsx import { StackNavigationProp } from '@react-navigation/stack'; import { RouteProp } from '@react-navigation/native'; type RootStackParamList = { Home: undefined; Details: { itemId: number }; }; type DetailsScreenRouteProp = RouteProp<RootStackParamList, 'Details'>; type DetailsScreenNavigationProp = StackNavigationProp<RootStackParamList, 'Details'>; interface DetailsScreenProps { route: DetailsScreenRouteProp; navigation: DetailsScreenNavigationProp; } const DetailsScreen: React.FC<DetailsScreenProps> = ({ route, navigation }) => { const { itemId } = route.params; return ( <View> <Text>Item ID: {itemId}</Text> <Button title="Go Back" onPress={() => navigation.goBack()} /> </View> ); }; export default DetailsScreen;
In this example, TypeScript ensures that the itemId parameter is correctly extracted from the navigation route, minimizing the chances of runtime errors.
Conclusion
Combining the power of React Native with TypeScript offers a fantastic development experience, empowering you to build type-safe and reliable mobile applications. With benefits like type safety, improved developer experience, and enhanced code maintainability, this duo is an excellent choice for both small-scale and large-scale app projects. As you embark on your journey to create cross-platform applications, remember to leverage the benefits of React Native and TypeScript to craft robust and polished mobile experiences. Happy coding!
Table of Contents
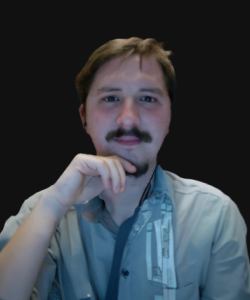
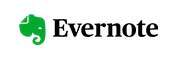