Creating Intuitive Screen Flows in React Native Using React Navigation
The art of creating intuitive, seamless, and smooth navigation between different screens in your mobile application is crucial to ensure a delightful user experience. When it comes to mobile apps, user experience is a top priority. React Native, a popular JavaScript framework for building mobile applications, provides several powerful navigation libraries to help developers create visually appealing, smooth navigation flows. This blog post will provide an in-depth overview of navigation in React Native, focusing on the navigation between different screens in your mobile application.
Introduction to React Native Navigation
React Native, created by Facebook, enables developers to build mobile applications using JavaScript and React. One key advantage of React Native is the ability to share code between iOS and Android, which can lead to significant time and cost savings.
React Native itself does not come with a built-in navigation solution. Instead, developers can choose from several navigation libraries, each with its own set of features and advantages. The two most widely used libraries are React Navigation and React Native Navigation.
React Navigation is a pure JavaScript solution, which is more in line with the React Native philosophy of “Learn once, write anywhere.” React Native Navigation, on the other hand, utilizes native navigation APIs on both iOS and Android, which can provide a more “native-like” feel and performance.
In this blog post, we’ll use React Navigation, as it is more widely used and provides more flexibility for JavaScript developers.
Setting Up React Navigation
Before we get into navigating between screens, we first need to install and set up React Navigation in our project. To do this, we will use npm (node package manager). Follow the steps below:
```bash # Install React Navigation npm install @react-navigation/native # Install dependencies npm install react-native-reanimated react-native-gesture-handler react-native-screens react-native-safe-area-context @react-native-community/masked-view # For iOS only, you need to pod install cd ios && pod install && cd .. ```
Creating Our First Screen
Let’s start by creating a simple screen. In React Native, screens are represented by components. Let’s create a HomeScreen:
```jsx import React from 'react'; import { View, Text, Button } from 'react-native'; const HomeScreen = ({ navigation }) => { return ( <View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}> <Text>Home Screen</Text> <Button title="Go to Details" onPress={() => navigation.navigate('Details')} /> </View> ); }; export default HomeScreen; ```
Here, we have a simple screen with a text that says ‘Home Screen’ and a button to navigate to the ‘Details’ screen.
The ‘navigation’ prop is passed to every screen component from the navigator. It has various methods to navigate between screens, among which the most commonly used one is `navigate`.
Adding a Navigator
To manage our screens and to provide us with the `navigation` prop, we need a navigator. In this case, we will use a Stack Navigator, which provides a way for your app to transition between screens where each new screen is placed on top of a stack.
To use this, we first need to install the stack navigator package:
```bash npm install @react-navigation/stack ```
Then we can create a stack navigator and add our `HomeScreen` to it:
```jsx import React from 'react'; import { createStackNavigator } from '@react-navigation/stack'; import HomeScreen from './HomeScreen'; const Stack = createStackNavigator(); function App() { return ( <NavigationContainer> <Stack.Navigator> <Stack.Screen name="Home" component={HomeScreen} /> </Stack.Navigator> </NavigationContainer> ); } export default App;
“`
Here, the `NavigationContainer` component is a component which manages our app state and links our top-level navigator to the app environment.
Creating a Second Screen
Next, we will create a new `DetailsScreen`:
```jsx import React from 'react'; import { View, Text, Button } from 'react-native'; const DetailsScreen = ({ navigation }) => { return ( <View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}> <Text>Details Screen</Text> <Button title="Go to Home" onPress={() => navigation.navigate('Home')} /> </View> ); }; export default DetailsScreen; ```
And then add it to our navigator:
```jsx import React from 'react'; import { createStackNavigator } from '@react-navigation/stack'; import HomeScreen from './HomeScreen'; import DetailsScreen from './DetailsScreen'; const Stack = createStackNavigator(); function App() { return ( <NavigationContainer> <Stack.Navigator> <Stack.Screen name="Home" component={HomeScreen} /> <Stack.Screen name="Details" component={DetailsScreen} /> </Stack.Navigator> </NavigationContainer> ); } export default App; ```
Now you can navigate between the Home and Details screens by pressing the buttons. The `navigate` function used here does two things: it navigates to the screen in the stack with the given name, and it sets up a back button to return to the previous screen.
Passing Parameters to Routes
You can pass parameters to a route by putting them in an object as a second parameter to the `navigate` function:
```jsx navigation.navigate('Details', { itemId: 86 }); ```
Read the parameters in your screen component:
```jsx const DetailsScreen = ({ route }) => { /* 2. Get the param */ const { itemId } = route.params; return ( <View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}> <Text>Details Screen</Text> <Text>Item Id: {JSON.stringify(itemId)}</Text> </View> ); }; ```
In the example above, we’re accessing the `itemId` parameter from the `route.params` object.
Conclusion
React Navigation offers a flexible and intuitive solution to handle the transition between different screens and passing data among them in a React Native application. It’s for reasons like this that many businesses choose to hire React Native developers. With React Navigation, these developers have access to several types of navigators, such as stack, tab, and drawer. This allows them to create a diverse range of navigation patterns to perfectly suit the application’s requirements.
Remember, the key to great mobile app navigation is to make it intuitive, fast, and accurate for users to find what they’re looking for. Whether you’re a seasoned React Native developer or a business looking to hire React Native developers for your project, always keep these user experience principles at the forefront. Happy coding!
Table of Contents
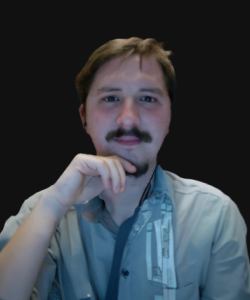
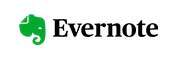