API Calls Made Easy: Crafting a Custom Axios Hook with ReactJS for Efficient Data Fetching
Welcome to our guide on “ReactJS useAxios: Building a Custom Axios Hook for Data Fetching”. This tutorial offers an in-depth exploration of how to maximize the benefits of Axios and ReactJS through the creation of a custom hook named “useAxios”. We’ll undertake a step-by-step process to build “useAxios”, demonstrating its utility for various data operations like GET, POST, PUT, and DELETE requests. This guide is designed to equip both seasoned developers and React newbies with valuable insights for enhancing their data fetching capabilities in React applications. Let’s get started and unlock the full potential of custom Axios hooks in your projects.
Understanding Axios
Axios is a robust, promise-based HTTP client for JavaScript, widely adopted due to its extensive features. It offers browser support, allows request and response interceptors, facilitates error handling, supports automatic data transformation, provides request cancellation, and even includes protections against Cross-Site Request Forgery attacks.
In the context of React, Axios is often the go-to choice for fetching data from APIs, and handling dynamic data rendering in applications. In our guide, we leverage Axios to create a custom React Hook, delivering an efficient, reusable solution for data fetching. This synthesis of Axios’s robustness with React’s efficiency marks a key advancement in modern web development.
Basics of ReactJS and Hooks
ReactJS is a versatile JavaScript library, designed for creating intuitive user interfaces and single-page applications. Its “component” concept promotes reusability and encapsulation.
React Hooks, introduced in version 16.8, revolutionized state and side effect management in functional components. Hooks such as `useState`, `useEffect`, and `useContext` offer simplicity and maintainability by enabling state and other features without classes. Additionally, custom Hooks foster code reusability and readability.
In terms of data fetching, React Hooks simplify the process, providing a way to logically compartmentalize related functionalities. We exploit this feature in our guide, creating a custom Axios hook, “useAxios”, to facilitate efficient data operations.
Understanding useAxios – A Custom Axios Hook
The useAxios custom hook is a powerful tool that combines the abilities of Axios and ReactJS Hooks to simplify the process of fetching data in your React application. It is a reusable function that handles Axios HTTP requests, providing an efficient and clean way to fetch, post, put, and delete data in your React components.
Here are some key features and benefits of the useAxios custom hook:
- Reusable: Once you’ve created the useAxios hook, you can use it in any component that needs to make HTTP requests. This reduces repetition and makes your codebase easier to manage.
- State Management: The useAxios hook encapsulates the handling of request states like loading, success, and error. It uses React’s useState and useEffect Hooks to track these states and update your components when they change.
- Simplified Syntax: By hiding the complexities of Axios and HTTP requests, useAxios provides a simple, intuitive interface for data fetching. Instead of dealing with promises and .then/.catch syntax, you can use a more straightforward syntax to make requests and handle responses.
- Flexibility: The useAxios hook can be customized to fit your needs. For example, you can modify it to provide different configurations for different API endpoints, or to handle unique requirements of your project.
In essence, useAxios is a bridge between the Axios library and ReactJS Hooks. It brings together the capabilities of both, creating a powerful tool that simplifies and optimizes the process of data fetching in your React applications. In the following sections, we’ll provide a step-by-step guide to building the useAxios hook, and demonstrate its use in a practical context.
Step-by-step Tutorial to Build useAxios Hook
Great, let’s dive into creating our custom Axios hook, `useAxios`. This hook will make HTTP requests and handle the request states (loading, success, error).
1. Initialize a new React Project
If you haven’t already, you’ll need to set up a new React project using Create React App or your preferred method.
2. Install Axios
Run the command `npm install axios` or `yarn add axios` to install Axios.
3. Create useAxios.js file
In your project, create a new file called `useAxios.js` where we’ll define our custom hook.
4. Import Necessary Dependencies
import { useState, useEffect } from 'react'; import axios from 'axios';
5. Create and Export useAxios Hook
export const useAxios = (url, method, data = null) => { const [response, setResponse] = useState(null); const [error, setError] = useState(''); const [loading, setLoading] = useState(true); const fetchData = async () => { axios.defaults.headers.post['Content-Type'] ='application/json;charset=utf-8'; axios.defaults.headers.post['Access-Control-Allow-Origin'] = '*'; try { const res = await axios[method](url, data); setResponse(res.data); } catch (err) { setError(err); } finally { setLoading(false); } }; useEffect(() => { fetchData(); }, [url, method, data]); return { response, error, loading }; };
Here’s a breakdown of the above code:
– `useAxios` takes three parameters: the request `url`, HTTP `method` (like ‘get’, ‘post’, ‘put’, ‘delete’), and `data` for the request body (default is `null` for GET requests).
– Three state variables `response`, `error`, and `loading` are declared using `useState`.
– `fetchData` is an asynchronous function that performs the Axios request.
– `setResponse` updates the `response` state with the data received from the request.
– If the request fails, `setError` is called with the error message.
– After the request is completed (regardless of success or failure), `setLoading` is called with `false` to indicate that loading is done.
– `useEffect` is called with `fetchData` as its argument, meaning `fetchData` will run every time the `url`, `method`, or `data` changes.
– `useAxios` returns an object containing `response`, `error`, and `loading`, which can be used by components to dynamically render based on the request’s state.
Now that you have a `useAxios` hook, you can use it in your React components to make HTTP requests, simplifying the process of data fetching and error handling.
Practical Implementation of useAxios in a ReactJS Project
Now that we have our `useAxios` custom hook, let’s see how it can be implemented in a real-world ReactJS project.
Assume we’re building a blog application where we want to fetch a list of posts from an API endpoint. Here’s how you can use `useAxios` in this context:
1. Import useAxios Hook
In the component where you want to fetch data, import the `useAxios` hook:
import { useAxios } from './useAxios';
2. Use the useAxios Hook
Call `useAxios` in your component, passing the appropriate parameters. In this case, the URL of the posts API and the HTTP method (‘get’):
const { response, error, loading } = useAxios('https://jsonplaceholder.typicode.com/posts', 'get');
3. Render the Component
Now, you can use the `response`, `error`, and `loading` values in your component to dynamically render your UI based on the state of the request:
function Posts() { const { response, error, loading } = useAxios('https://jsonplaceholder.typicode.com/posts', 'get'); if (loading) { return <div>Loading...</div>; } if (error) { return <div>Error! {error.message}</div>; } return ( <div> {response.map(post => ( <div key={post.id}> <h2>{post.title} </h2> <p>{post.body}</p> </div> ))} </div> ); }
In this example, while the data is being fetched, a “Loading…” message is displayed. If there’s an error during the request, it displays an error message. Once the data has been fetched, it iterates over the posts in the response and displays their title and body.
Note that `useAxios` is versatile and can be used for other HTTP methods like post, put, and delete. Simply replace the URL and the method, and provide data if needed (like for post and put requests).
With `useAxios`, you have a powerful, reusable data fetching hook that can help you write cleaner, more efficient React applications. It’s a practical demonstration of how custom hooks can be used to encapsulate and simplify complex functionality.
Tips and Best Practices
Having implemented a custom Axios Hook in React, consider these best practices for enhanced usage:
- Error Handling: Prioritize robust error handling. Augment the basic handling in `useAxios` with user-friendly messages and error boundary components or libraries like react-error-boundary.
- Testing: Use libraries like Jest and @testing-library/react-hooks to rigorously test your hooks, ensuring they handle various server responses and states correctly.
- Type Checking: With TypeScript, clearly define types for your hook’s parameters and return values.
- Caching: Implement caching to avoid redundant network requests. Libraries like react-query or SWR can assist with this.
- Throttling/Debouncing: If your hook handles user input, throttle or debounce requests to limit their number.
- Cancellation: Consider a cancellation mechanism for HTTP requests to prevent memory leaks.
- React DevTools: Utilize this tool for inspecting hooks and their states during development.
- Simplicity: Keep your custom hook as uncomplicated as possible, as they’re meant to abstract complex logic.
Adopting these practices can help achieve clean, maintainable, and robust code, optimizing your `useAxios` hook usage in React applications.
Conclusion
Through this guide, we’ve harnessed the strengths of ReactJS and Axios to create a custom hook, `useAxios`, combining React’s simplicity with Axios’s robust data fetching. We’ve delved into the basics of Axios and React Hooks, constructed the `useAxios` hook, and demonstrated its practical application.
No matter the scale of your project, mastering custom hooks like `useAxios` can significantly enhance your development experience and codebase efficiency. The key to mastering such hooks lies in continual practice and exploration. So, keep refining your custom hooks, explore various use-cases, and push your limits with React Hooks and Axios. Happy coding!
Table of Contents
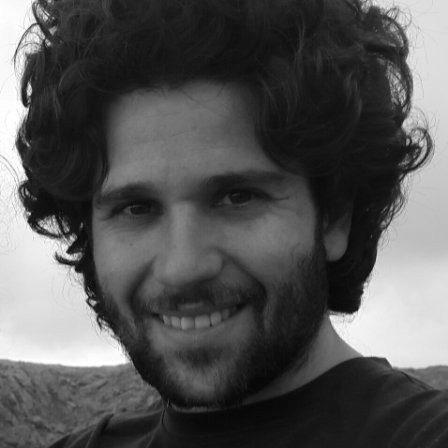
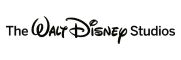