Writing Clean and Efficient Code: Best Practices for ReactJS Development
ReactJS is a popular JavaScript library for building user interfaces. As with any programming language or library, it’s important to follow best practices to ensure that your code is clean, efficient, and maintainable.
Table of Contents
In this blog post, we’ll explore some best practices for writing clean and efficient code in ReactJS.
1. Keep Components Small and Focused
One of the most important best practices in ReactJS is to keep your components small and focused. Components should do one thing and do it well. This makes them easier to test, reuse, and maintain.
If a component becomes too large and complex, it’s a good idea to break it down into smaller, more focused components. This will make your code easier to read and understand, and will also improve performance.
2. Use Pure Components Whenever Possible
Pure components are components that only depend on their props and state. They don’t have any side effects and always return the same output for the same input. This makes them ideal for optimizing performance.
To create a pure component in ReactJS, use the React.memo Higher Order Component (HOC). This HOC will only re-render the component if its props have changed.
Here’s an example:
javascript
import React from 'react'; function MyComponent({ text }) { return <p>{text}</p>; } export default React.memo(MyComponent);
In this example, we define a MyComponent component that only renders a text prop. We wrap the component in the React.memo HOC to make it a pure component.
3. Avoid Using setState in render Method
The render method in a component should only be used for rendering HTML elements. It should not be used to modify state. Doing so can cause an infinite loop and impact performance.
If you need to modify state, use the componentDidMount, componentDidUpdate, or componentWillUnmount lifecycle methods instead.
4. Use key Prop to Improve Performance
When rendering a list of components, ReactJS needs a way to uniquely identify each component. This is where the key prop comes in.
By assigning a unique key prop to each component in the list, ReactJS can more efficiently update the list when changes occur. Here’s an example:
javascript
import React from 'react'; function MyList({ items }) { return ( <ul> {items.map((item) => ( <li key={item.id}>{item.text}</li> ))} </ul> ); }
In this example, we define a MyList component that renders a list of items. We assign a unique key prop to each item in the list.
5. Use PropTypes to Validate Props
PropTypes is a package that allows you to validate the props passed to your components. This can help catch errors and make your code more robust.
To use PropTypes, import the package and define the prop types for your component. Here’s an example:
javascript
import React from 'react'; import PropTypes from 'prop-types'; function MyComponent({ text }) { return <p>{text}</p>; } MyComponent.propTypes = { text: PropTypes.string.isRequired, }; export default MyComponent;
In this example, we define a MyComponent component that expects a text prop of type string. We use PropTypes to validate the prop and ensure that it’s required.
6. Use CSS Modules or Styled Components for Styling
Styling in ReactJS can quickly become complex and difficult to manage. To simplify your styling, consider using CSS Modules or Styled Components.
CSS Modules allow you to write regular CSS styles and import them into your component files. The styles are scoped to the component, so you don’t have to worry about style conflicts between components. Here’s an example:
javascript
import React from 'react'; import styles from './MyComponent.module.css'; function MyComponent() { return <div className={styles.wrapper}>Hello World</div>; } export default MyComponent;
In this example, we define a MyComponent component and import the styles from a separate CSS file. We apply the styles to the component using the className prop.
Styled Components, on the other hand, allow you to write CSS styles directly in your JavaScript code. This makes it easier to manage your styles and ensures that they are only applied to the components that need them. Here’s an example:
javascript
import React from 'react'; import styled from 'styled-components'; const Wrapper = styled.div` background-color: #f0f0f0; padding: 20px; `; function MyComponent() { return <Wrapper>Hello World</Wrapper>; } export default MyComponent;
In this example, we define a Wrapper-styled component that applies a background color and padding. We use the Wrapper component in our MyComponent component to apply the styles.
7. Use React Developer Tools for Debugging
React Developer Tools is a browser extension that allows you to inspect the component tree, view props and state, and debug your ReactJS code. It’s a valuable tool for debugging and optimizing your ReactJS applications.
You can download React Developer Tools for Chrome, Firefox, and Edge from the Chrome Web Store, Firefox Add-ons, and Microsoft Edge Add-ons stores, respectively.
8. Use Functional Programming Concepts
ReactJS lends itself well to functional programming concepts, such as immutability and pure functions. By using these concepts, you can write cleaner and more efficient code.
Immutability refers to the idea that data should not be modified directly. Instead, you should create a new copy of the data with the desired changes. This helps prevent side effects and makes your code more predictable.
Pure functions are functions that only depend on their input and produce the same output for the same input. They don’t modify any external state or have any side effects. This makes them easier to test and reason about.
By using functional programming concepts in your ReactJS code, you can write code that is easier to understand, maintain, and test.
9. Conclusion
In this blog post, we explored some best practices for writing clean and efficient code in ReactJS. We discussed the importance of keeping components small and focused, using pure components, avoiding using setState in the render method, using the key prop, using PropTypes to validate props, using CSS Modules or Styled Components for styling, using React Developer Tools for debugging, and using functional programming concepts.
By following these best practices, you can write code that is easier to understand, maintain, and test. Keep these tips in mind when developing your next ReactJS project and watch as your code becomes more readable, maintainable, and performant.
Table of Contents
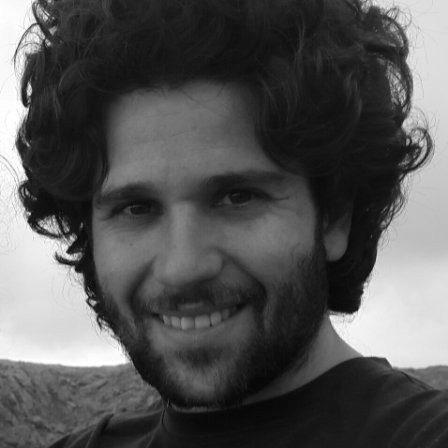
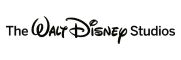