Building and Managing UI Elements with ReactJS Components
ReactJS is a popular JavaScript library for building user interfaces. At the core of ReactJS is its component-based architecture, which allows developers to create reusable and modular UI elements. In this blog post, we’ll take a deep dive into ReactJS components, exploring how to build and manage them effectively.
Table of Contents
1. Understanding ReactJS Components
A ReactJS component is a self-contained unit of functionality that can be composed together with other components to create a complete user interface. Components can be defined using either JavaScript classes or functions.
Class components are more powerful and can include state and lifecycle methods. Function components are simpler and more lightweight. They are typically used for presentational components, while class components are used for more complex components that need to manage state and handle user interactions.
Example of a simple function component:
javascript
function Welcome(props) { return <h1>Hello, {props.name}!</h1>; }
This component takes a “name” prop and uses it to render a personalized greeting to the screen. You can include this component in other components by importing it and rendering it as JSX.
2. JSX Syntax
JSX is a syntax extension for JavaScript that allows you to write HTML-like code in your ReactJS components. JSX is not required to use ReactJS, but it is highly recommended because it makes your components more readable and easier to understand.
Example of a JSX component:
javascript
function Greeting(props) { return <div>Hello, {props.name}!</div>; }
This component takes a “name” prop and uses it to render a personalized greeting to the screen. Notice how the “name” prop is included within curly braces in the JSX.
3. Props and State
In ReactJS, data flows from parent components to child components via props. Props are read-only and cannot be modified by the child component.
Here’s an example of a parent component that passes a prop to a child component:
javascript
function App() { return <ChildComponent name="Alice" />; } function ChildComponent(props) { return <div>Hello, {props.name}!</div>; }
This component hierarchy consists of a parent component (App) and a child component (ChildComponent). The parent component passes a “name” prop to the child component, which uses it to render a personalized greeting.
In addition to props, ReactJS components can also have state. State is used to manage data that changes over time. State is typically used to manage user input, application settings, and other dynamic data.
To manage state in ReactJS, you can use the useState hook. This hook allows you to add state to your function components without having to convert them to class components.
Here’s an example of a component that uses the useState hook to manage a counter:
javascript
function Counter() { const [count, setCount] = useState(0); function handleClick() { setCount(count + 1); } return ( <div> <p>You clicked {count} times.</p> <button onClick={handleClick}>Click me</button> </div> ); }
This component uses the useState hook to add a “count” state variable to the component. The component also includes a button that, when clicked, updates the count state variable.
3. Managing Component State with Lifecycle Methods
Lifecycle methods are a key feature of ReactJS that allow you to perform actions at different stages of a component’s lifecycle. Lifecycle methods can be used to manage component state, fetch data from external APIs, and perform other actions.
There are several lifecycle methods available in ReactJS, including componentDidMount, componentDidUpdate, and componentWillUnmount. These methods are called in a specific order during the component’s lifecycle.
Here’s an example of a class component that uses the componentDidMount lifecycle method to fetch data from an external API:
javascript
class UserList extends React.Component { constructor(props) { super(props); this.state = { users: [] }; } async componentDidMount() { const response = await fetch('https://jsonplaceholder.typicode.com/users'); const data = await response.json(); this.setState({ users: data }); } render() { return ( <div> <h1>User List</h1> <ul> {this.state.users.map(user => <li key={user.id}>{user.name}</li>)} </ul> </div> ); } }
This class component uses the componentDidMount lifecycle method to call the fetchUsers function after the component is mounted. The component also includes a users state variable that is updated with the data fetched from the external API.
4. Building Reusable Components
One of the main benefits of ReactJS is its ability to create reusable and modular UI components. By breaking down your UI into smaller, self-contained components, you can build more maintainable and flexible applications.
To build reusable components in ReactJS, you should follow these best practices:
- Keep components small and focused: Each component should be responsible for a specific task or set of tasks.
- Use props to pass data between components: By passing data between components via props, you can create a more modular and reusable architecture.
- Use composition to build complex components: You can combine multiple smaller components to create more complex UI elements.
- Use the React Developer Tools: The React Developer Tools is a browser extension that allows you to inspect and debug React components in your application. This tool can be invaluable when you’re trying to understand how your components are working.
5. Conclusion
ReactJS is a powerful tool for building reusable and maintainable UI components. By understanding the basics of ReactJS components, you can create dynamic and engaging web applications that meet your users’ needs. In this blog post, we covered the fundamentals of ReactJS components, including JSX syntax, props and state management, and lifecycle methods. We also explored best practices for building reusable components that are easy to maintain and extend. With these skills, you can become a proficient ReactJS developer and build amazing applications that delight your users.
Table of Contents
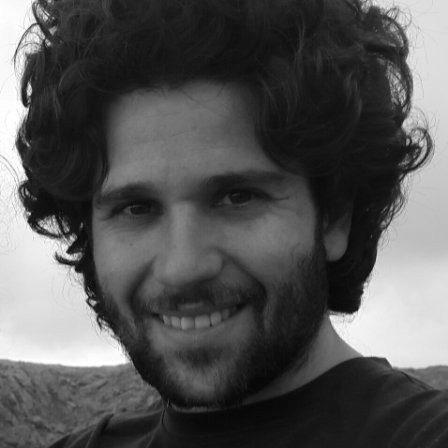
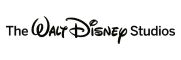