A Guide to Building Progressive Web Apps with ReactJS
Progressive Web Apps (PWAs) are a new generation of web applications that deliver native-like experiences to users through the use of modern web technologies. PWAs are fast, reliable, and engaging, offering a seamless experience across different devices and network conditions.
Table of Contents
In this comprehensive guide, we’ll explore how to build PWAs using ReactJS, a popular JavaScript library for building user interfaces, and discuss the key features and considerations for creating high-quality PWAs.
1. Understanding the Basics of PWAs
PWAs combine the best of both worlds – the discoverability and reach of the web and the performance and user experience of native applications. Some key features of PWAs include:
- Responsive design: PWAs adapt to different screen sizes, devices, and orientations, providing a consistent user experience across different platforms.
- Offline capabilities: PWAs can work offline or on low-quality networks, thanks to the use of service workers and caching strategies.
- App-like experience: PWAs provide an app-like experience through the use of an app shell architecture and smooth navigation.
- Installability: Users can install PWAs on their devices, providing easy access to the application without the need for app stores.
- Secure connections: PWAs are served over HTTPS, ensuring secure data transmission and protecting user data.
2. Setting up a React PWA
Creating a PWA with ReactJS is simple, thanks to the Create React App (CRA) tool. CRA offers a built-in PWA template that includes a service worker and other PWA-related configurations.
To create a new React PWA, run the following command:
bash
npx create-react-app my-pwa --template cra-template-pwa
This command will generate a new React project with PWA configurations in place.
3. Configuring the Service Worker
Service workers are a key component of PWAs, providing offline capabilities, caching strategies, and other enhancements. CRA automatically generates a basic service worker for your PWA. To customize the service worker, you can modify the src/service-worker.js file.
Some common customizations include:
- Adding custom caching strategies for different types of assets (e.g., images, fonts, API responses).
- Implementing background sync to synchronize data when the user regains connectivity.
- Handling push notifications and other background tasks.
4. Implementing an App Shell
An app shell is the minimal HTML, CSS, and JavaScript required to power the user interface of a PWA. The app shell architecture enables fast loading and smooth navigation, providing an app-like experience for users.
To implement an app shell in your React PWA:
- Structure your application components to load the essential UI elements first, followed by the dynamic content.
- Use React’s built-in lazy loading feature to load non-critical components and assets on-demand.
- Leverage code-splitting techniques to minimize the initial JavaScript payload and reduce loading times.
5. Creating a Responsive Design
A responsive design is crucial for providing a consistent user experience across different devices and screen sizes. To create a responsive design for your React PWA:
- Use CSS frameworks like Bootstrap, Material-UI, or Tailwind CSS to build a flexible and adaptive UI.
- Use CSS media queries to apply different styles based on the screen size and device features.
- Leverage React’s built-in features, like conditional rendering, to adapt your application’s UI and behavior based on the device capabilities.
6. Adding Installability
To make your React PWA installable, you’ll need to create a web app manifest file (public/manifest.json). This file provides metadata about your PWA, such as its name, icons, display mode, and start URL.
Make sure your manifest file includes:
- A short and long name for your application.
- App icons in different sizes and formats to support various devices.
- The start_url attribute, which specifies the initial page loaded when the app is launched.
- The display attribute, which controls how the app is displayed (e.g., standalone, fullscreen, or minimal-ui).
Here’s an example of a web app manifest:
json
{ "short_name": "MyPWA", "name": "My Progressive Web App", "icons": [ { "src": "icon-192x192.png", "sizes": "192x192", "type": "image/png" }, { "src": "icon-512x512.png", "sizes": "512x512", "type": "image/png" } ], "start_url": "/", "display": "standalone", "background_color": "#ffffff", "theme_color": "#000000" }
7. Testing and Optimizing Your PWA
It’s important to test and optimize your React PWA to ensure it meets the performance and usability requirements of a high-quality PWA. Some tools and strategies for testing and optimizing your PWA include:
- Use Lighthouse, a performance and quality auditing tool, to assess your PWA’s performance, accessibility, SEO, and other best practices.
- Test your PWA on various devices and network conditions to ensure it provides a consistent user experience.
- Optimize your application’s performance by implementing best practices like minification, compression, and browser caching.
- Regularly update your dependencies and address any performance or security issues that may arise.
8. Conclusion
Building a Progressive Web App with ReactJS is a powerful way to create fast, reliable, and engaging web applications that provide native-like experiences to users. By following this comprehensive guide, you’ll be well on your way to building a high-quality PWA using ReactJS. Keep in mind the key features and best practices discussed in this post, and your PWA will not only provide an outstanding user experience but also help you reach a broader audience across various devices and network conditions.
Table of Contents
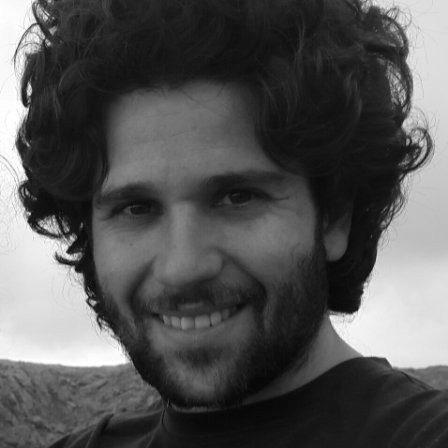
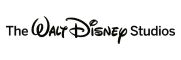