Building Scalable ReactJS Applications: An Architectural Approach
As ReactJS has become more popular, so have the challenges of building scalable applications with it. In this blog post, we’ll explore an architectural approach to building scalable ReactJS applications.
We’ll cover the key principles of scalable architecture, common design patterns, and tools that can help you build scalable applications with ReactJS.
Understanding Scalable Architecture
Scalable architecture refers to the design principles and patterns used to build applications that can handle growing amounts of data, traffic, and users. Key principles of scalable architecture include:
- Separation of concerns: Dividing an application into distinct and independent modules to reduce complexity and increase maintainability.
- Modular design: Building applications as a set of reusable and interchangeable modules, allowing for flexibility and adaptability.
- Loose coupling: Ensuring that modules are independent of one another and can be changed or updated without affecting other parts of the application.
- High availability: Designing applications that are highly available and can handle failures without significant downtime.
Common Design Patterns for Scalable ReactJS Applications
There are several design patterns that can be used to build scalable ReactJS applications, including:
- Container/Component pattern: Separating presentation logic (components) from data handling (containers), making it easier to manage and scale applications.
- Redux pattern: Using a central store to manage application state, making it easier to manage data and communicate between components.
- Higher-Order Component (HOC) pattern: Wrapping components in HOCs to add functionality and reduce duplication of code.
Tools for Building Scalable ReactJS Applications
There are several tools that can help you build scalable ReactJS applications, including:
- React Router: A library for handling routing and navigation in React applications.
- Redux Thunk: A middleware for Redux that allows you to write asynchronous actions.
- React Testing Library: A testing utility for React applications that emphasizes accessibility and user behavior.
- ESLint: A code quality tool that can help you enforce coding standards and prevent common errors.
Building a Scalable ReactJS Application
To build a scalable ReactJS application, you’ll need to apply the key principles of scalable architecture, use common design patterns, and leverage the right tools. Here’s an example of how to apply these principles to a basic ReactJS application:
- Use the container/component pattern to separate presentation logic from data handling:
import React from "react"; import { connect } from "react-redux"; import { fetchData } from "../actions"; const Component = ({ data, fetchData }) => { useEffect(() => { fetchData(); }, []); return <div>{data ? data.map((item) => <p key={item.id}>{item.title}</p>) : "Loading..."}</div>; }; const mapStateToProps = (state) => ({ data: state.data, }); export default connect(mapStateToProps, { fetchData })(Component);
- Use Redux to manage application state:
import { createStore, applyMiddleware } from "redux"; import thunk from "redux-thunk"; const initialState = { data: null, }; const reducer = (state = initialState, action) => { switch (action.type) { case "FETCH_DATA_SUCCESS": return { ...state, data: action.payload }; default: return state; } }; const store = createStore(reducer, applyMiddleware(thunk)); export default store;
- Use React Router for routing and navigation:
import { BrowserRouter as Router, Route, Switch } from "react-router-dom"; import Home from "./components/Home"; import About from "./components/About"; const App = () => { return ( <Router> <Switch> <Route exact path="/" component={Home} /> <Route exact path="/about" component={About} /> </Switch> </Router> ); };
export default App;
- Use ESLint to enforce coding standards and prevent common errors: ```javascript { "extends": [ "eslint:recommended", "plugin:react/recommended", "plugin:prettier/recommended" ], "parserOptions": { "ecmaVersion": 2018, "sourceType": "module", "ecmaFeatures": { "jsx": true } }, "plugins": ["react", "prettier"], "rules": { "prettier/prettier": "error", "react/prop-types": 0 } }
Conclusion
Building scalable ReactJS applications requires a solid understanding of scalable architecture, common design patterns, and the right tools. By applying the key principles of scalable architecture and using common design patterns and tools like React Router, Redux, and ESLint, you’ll be well on your way to building scalable and maintainable ReactJS applications that can handle growing amounts of data and traffic.
Table of Contents
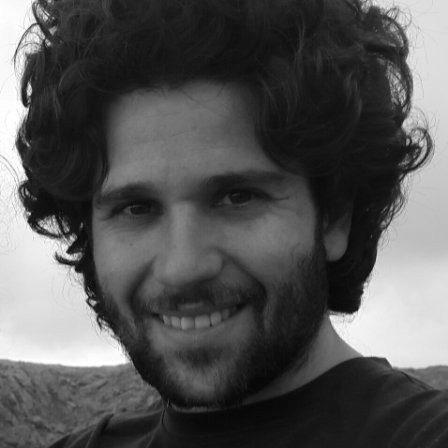
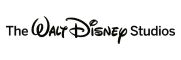