Building Modern Web Applications with ReactJS and GraphQL
Modern web applications require efficient data fetching and management to deliver top-notch user experiences. ReactJS, a popular JavaScript library for building user interfaces, can be paired with GraphQL, a flexible and powerful query language for APIs, to create efficient and scalable web applications.
Table of Contents
In this blog post, we’ll explore how to combine ReactJS and GraphQL to build modern web applications, discuss the benefits of this approach, and walk through key concepts and tools to help you get started.
1. Understanding GraphQL
GraphQL is a query language for APIs developed by Facebook in 2012 and released as an open-source project in 2015. It provides a more efficient, flexible, and powerful alternative to the traditional REST API. Some key features of GraphQL include:
- Flexible data fetching: GraphQL allows clients to request only the data they need, reducing the amount of over- or under-fetching.
- Strongly typed schema: GraphQL enforces a strongly typed schema, ensuring that clients and servers communicate using well-defined types and structures.
- Real-time updates: GraphQL supports real-time updates through subscriptions, enabling clients to receive live updates when data changes.
- Single endpoint: GraphQL APIs have a single endpoint, simplifying API management and versioning.
2. Setting Up a React Application with GraphQL
To integrate GraphQL in a React application, you’ll need to use a GraphQL client library. Apollo Client is a popular and feature-rich GraphQL client that works well with React.
To set up a new React application with Apollo Client, follow these steps:
- Create a new React application using Create React App:
bash
npx create-react-app my-graphql-app
- Install the required Apollo Client packages:
bash
npm install @apollo/client graphql
- In your React application, set up the Apollo Client by wrapping your application with the ApolloProvider component and passing your GraphQL endpoint:
javascript
import { ApolloClient, InMemoryCache, ApolloProvider } from "@apollo/client"; const client = new ApolloClient({ uri: "https://your-graphql-api.com/graphql", cache: new InMemoryCache(), }); ReactDOM.render( <ApolloProvider client={client}> <App /> </ApolloProvider>, document.getElementById("root") );
3. Fetching Data with GraphQL Queries
In a React application, you can use the useQuery hook from Apollo Client to fetch data from your GraphQL API.
First, define your GraphQL query using the gql template literal tag:
javascript
import { gql } from "@apollo/client"; const GET_USERS = gql` query GetUsers { users { id name email } } `;
Next, use the useQuery hook in your React component to fetch the data and render it:
javascript
import { useQuery } from "@apollo/client"; const UsersList = () => { const { loading, error, data } = useQuery(GET_USERS); if (loading) return <p>Loading...</p>; if (error) return <p>Error :(</p>; return ( <ul> {data.users.map((user) => ( <li key={user.id}> {user.name} - {user.email} </li> ))} </ul> ); };
4. Modifying Data with GraphQL Mutations
To modify data in your GraphQL API, you can use GraphQL mutations. In a React application, you can use the useMutation hook from Apollo Client to perform mutations.
First, define your GraphQL mutation using the gql template literal tag:
javascript
import { gql } from "@apollo/client"; const ADD_USER = gql mutation AddUser($name: String!, $email: String!) { addUser(name: $name, email: $email) { id name email } };
vbnet
Next, use the `useMutation` hook in your React component to perform the mutation and update your component state: ```javascript import { useMutation } from "@apollo/client"; const AddUserForm = () => { const [name, setName] = useState(""); const [email, setEmail] = useState(""); const [addUser, { data }] = useMutation(ADD_USER); const handleSubmit = (event) => { event.preventDefault(); addUser({ variables: { name, email } }); setName(""); setEmail(""); }; return ( <form onSubmit={handleSubmit}> <input value={name} onChange={(e) => setName(e.target.value)} placeholder="Name" /> <input value={email} onChange={(e) => setEmail(e.target.value)} placeholder="Email" /> <button type="submit">Add User</button> </form> ); };
5. Real-time Updates with GraphQL Subscriptions
GraphQL subscriptions enable real-time updates in your application when data changes. To use subscriptions in your React application, you’ll need to configure your Apollo Client to use a WebSocket link for subscriptions.
First, install the subscriptions-transport-ws package:
bash
npm install subscriptions-transport-ws
Next, update your Apollo Client setup to use a WebSocket link for subscriptions:
javascript
import { ApolloClient, InMemoryCache, ApolloProvider, split, HttpLink } from "@apollo/client"; import { WebSocketLink } from "@apollo/client/link/ws"; import { getMainDefinition } from "@apollo/client/utilities"; const httpLink = new HttpLink({ uri: "https://your-graphql-api.com/graphql", }); const wsLink = new WebSocketLink({ uri: "wss://your-graphql-api.com/graphql", options: { reconnect: true, }, }); const link = split( ({ query }) => { const definition = getMainDefinition(query); return ( definition.kind === "OperationDefinition" && definition.operation === "subscription" ); }, wsLink, httpLink ); const client = new ApolloClient({ link, cache: new InMemoryCache(), }); ReactDOM.render( <ApolloProvider client={client}> <App /> </ApolloProvider>, document.getElementById("root") );
Finally, use the useSubscription hook in your React component to listen for updates:
javascript
import { useSubscription } from "@apollo/client"; const USER_ADDED = gql` subscription { userAdded { id name email } } `; const UsersList = () => { const { data, loading, error } = useSubscription(USER_ADDED); // ... Render the updated data as needed };
6. Conclusion
Combining ReactJS and GraphQL empowers you to build modern web applications with efficient data fetching and management. This powerful duo provides flexibility and scalability, allowing you to create tailored experiences for your users. By following the key concepts and tools outlined in this blog post, you’ll be well on your way to mastering the art of building modern web applications with ReactJS and GraphQL.
Table of Contents
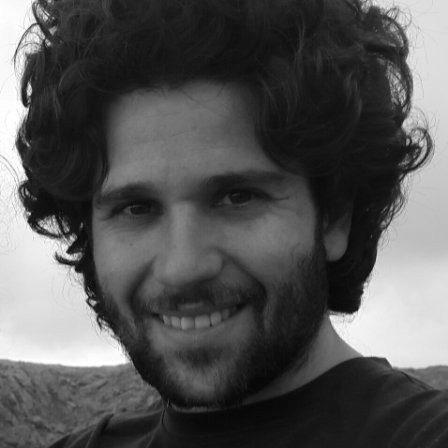
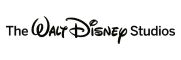