Enhance Performance with Webpack Optimization
In modern web development, managing dependencies, bundling assets, and optimizing your application for production can be a complex and time-consuming process. Webpack, a powerful and popular module bundler, simplifies this process and allows developers to create optimized builds with ease.
Table of Contents
In this blog post, we will provide a comprehensive guide to using Webpack with ReactJS, covering the basics of bundling, optimization techniques, and advanced configuration options.
1. Getting Started with ReactJS and Webpack
Before diving into Webpack configuration, ensure that you have Node.js and npm installed on your machine. Begin by creating a new React project and installing the necessary dependencies.
bash
mkdir react-webpack && cd react-webpack npm init -y npm install --save react react-dom npm install --save-dev webpack webpack-cli webpack-dev-server babel-loader @babel/core @babel/preset-env @babel/preset-react html-webpack-plugin v @babel/preset-react html-webpack-plugin
1.1 Basic Webpack Configuration
Now, create a webpack.config.js file in the root of your project to configure Webpack. This file will define the entry point for your application, specify the output location for your bundled assets, and configure various loaders and plugins.
javascript
const HtmlWebpackPlugin = require("html-webpack-plugin"); module.exports = { entry: "./src/index.js", output: { path: __dirname + "/dist", filename: "bundle.js", }, module: { rules: [ { test: /\.(js|jsx)$/, exclude: /node_modules/, use: { loader: "babel-loader", options: { presets: ["@babel/preset-env", "@babel/preset-react"], }, }, }, ], }, plugins: [ new HtmlWebpackPlugin({ template: "./src/index.html", }), ], resolve: { extensions: [".js", ".jsx"], }, };
In the configuration above, we have defined the following:
- The entry point for our application, which is ./src/index.js.
- The output location and filename for our bundled assets.
- A module rule for transpiling JavaScript and JSX files using Babel.
- A plugin for generating an HTML file that includes our bundled assets.
- A resolve property to automatically resolve file extensions for imports.
2. Optimizing Your React Application
Webpack provides numerous optimization techniques to improve the performance and reduce the size of your React application. Some key optimizations include:
2.1 Code Splitting:
Divide your code into smaller chunks to load only what is necessary for a particular part of your application.
javascript
// Inside your webpack.config.js const { SplitChunksPlugin } = require("webpack").optimize; module.exports = { //... optimization: { splitChunks: { chunks: "all", }, }, //... };
2.2 Minification:
Minify your JavaScript, CSS, and HTML files to reduce their size.
bash
npm install --save-dev terser-webpack-plugin css-minimizer-webpack-plugin html-minimizer-webpack-plugin html-minimizer-webpack-plugin javascript // Inside your webpack.config.js const TerserPlugin = require("terser-webpack-plugin"); const CssMinimizerPlugin = require("css-minimizer-webpack-plugin"); const HtmlMinimizerPlugin = require("html-minimizer-webpack-plugin"); module.exports = { //... optimization: { minimize: true, minimizer: [ new TerserPlugin(), new CssMinimizerPlugin(), new HtmlMinimizerPlugin(), ], }, //... };
2.3 Tree Shaking:
Eliminate dead code and unused exports to reduce the size of your bundled JavaScript files.
javascript
// Inside your webpack.config.js module.exports = { //... mode: "production", // Enables tree shaking in production mode //... };
2.4 Environment Variables:
Use environment variables to conditionally include or exclude code depending on the build environment.
bash
npm install --save-dev dotenv-webpack
javascript
// Inside your webpack.config.js const DotenvWebpackPlugin = require("dotenv-webpack"); module.exports = { //... plugins: [ //... new DotenvWebpackPlugin(), ], //... };
3. Advanced Webpack Configuration
Webpack offers numerous advanced configuration options to further enhance your React application’s build process:
3.1 Loaders for handling other file types, such as CSS, images, and fonts.
bash
npm install --save-dev style-loader css-loader file-loader
javascript
// Inside your webpack.config.js module.exports = { //... module: { rules: [ //... { test: /\.css$/, use: ["style-loader", "css-loader"], }, { test: /\.(png|jpe?g|gif|svg|woff|woff2|eot|ttf|otf)$/, use: ["file-loader"], }, ], }, //... };
3.2 Hot Module Replacement (HMR)
For a better development experience by enabling live reloading of your application without a full page refresh.
javascript
// Inside your webpack.config.js const { HotModuleReplacementPlugin } = require("webpack"); module.exports = { //... devServer: { contentBase: "./dist", hot: true, }, plugins: [ //... new HotModuleReplacementPlugin(), ], //... };
4. Conclusion
Webpack is a powerful and versatile tool that can greatly simplify the process of bundling and optimizing your ReactJS applications. By following this comprehensive guide, you can leverage Webpack’s features to create highly optimized, high-performance applications with ease. With proper configuration, you can take advantage of code splitting, minification, tree shaking, and other advanced features to make your React projects even more efficient and easy to maintain.
Table of Contents
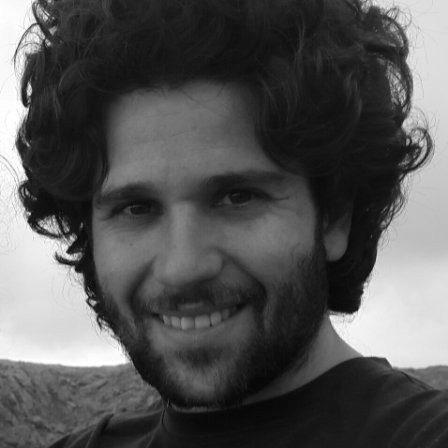
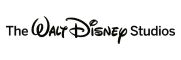